How to specialize mapM for IO in Haskell
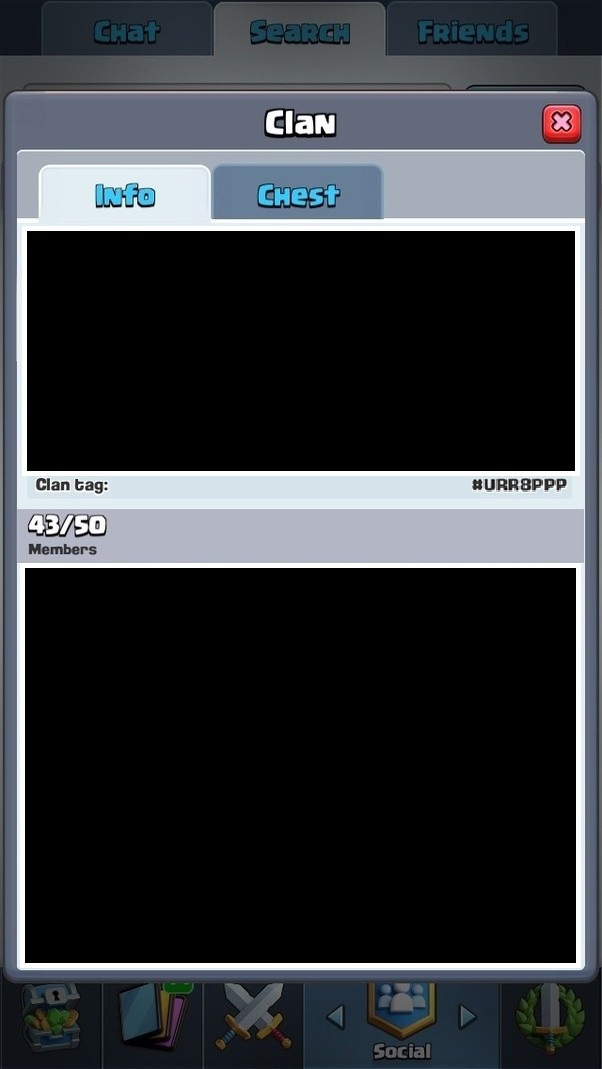
Clash Royale CLAN TAG #URR8PPP How to specialize mapM for IO in Haskell Say I have a task that represents some computation from k to v where some inputs have to be fetched externally. k v newtype Task k v = Task { run ∷ ∀ f. Monad f ⇒ (k → f v) → f v } For some tasks mapM will be used, e.g. to fetch multiple keys. I want to specialize mapM for some monads. Specifically for the IO monad I want to use Control.Concurrent.Async.mapConcurrently to perform actions concurrently. mapM mapM IO Control.Concurrent.Async.mapConcurrently My first instinct is to introduce a wrapper type newtype AsyncIO a = AsyncIO { io :: IO a } and then introduce instance Monad AsyncIO However this doesn't work because in the current GHC implementation mapM is defined in term of traverse , which is in Traversable . mapM traverse Traversable Is there an elegant solution for this? I don't quite understand what you mean by "for some tasks mapM will be used". Could