How do I add a string and an int object in Python?
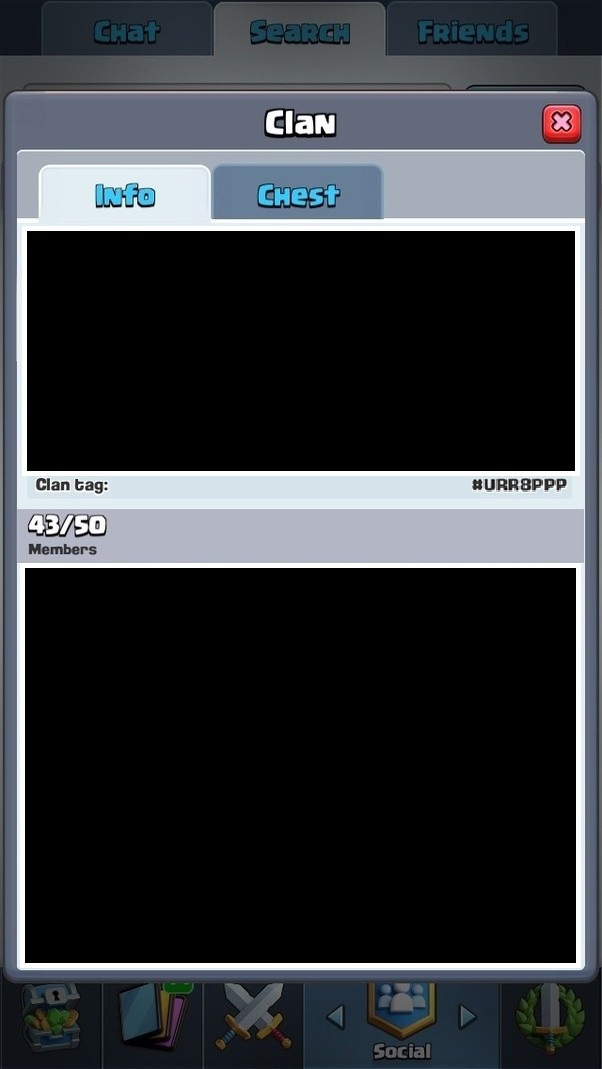

How do I add a string and an int object in Python?
What do I need?
I have SQL content like:
('a', 1),
So I do:
return_string = '('
for column in columns:
return_string += "'" + column + "', "
return_string = return_string[:-2] + '),'
return return_string
But it fails with the same error.
>>> a = 'a'
>>> a + 1
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: cannot concatenate 'str' and 'int' objects
>>> 1 + "1"
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'str'
>>>
However, if I convert an int into a string, everything works and I get:
('a', '1'),
But I need
('a', 1),
where 1 is unquoted '
'
4 Answers
4
String concatenation in python only works between strings, it doesn't infer types based on need like other languages.
There are two options, cast the integer to a strings and add it all together:
>>> x ="a"
>>> y = 1
>>> "("+x+","+str(y)+")"
'(a,1)'
>>> "('"+x+"',"+str(y)+")"
"('a',1)"
>>> "("+repr(x)+","+str(y)+")"
"('a',1)"
Or use string formatting to take care of some of this behind the scenes. Either using (deprecated) "percent formatting":
>>> "(%s,%d)"%(x,y)
'(a,1)'
>>> "('%s',%d)"%(x,y)
"('a',1)"
>>> "(%s,%d)"%(repr(x),y)
"('a',1)"
Or the more standard and approved format mini-language:
>>> "({0},{1})".format(x,y)
'(a,1)'
>>> "('{0}',{1})".format(x,y)
"('a',1)"
>>> "({0},{1})".format(repr(x),y)
"('a',1)"
%
str.format
@KonradRudolph Percent is quicker to type and get an answer up. Once its in I always add format after (I can never remember some of the more interesting syntax of it).
– user764357
Jan 21 '14 at 23:42
@KonradRudolph percent is also quicker execution!
– mhlester
Jan 21 '14 at 23:45
@mhlester Do you have statistics to prove this? Even so, “correct” is better than “fast but broken”, and the documentation says specifically that “… [% substitutions] are obsolete and may go away in future versions of Python”.
– Konrad Rudolph
Jan 22 '14 at 0:28
@KonradRudolph, yes, but hard to fit in a comment:
timeit(lambda: "('%s', %d)" % ('a', 1), number=1000000)
and timeit(lambda: "('{0}', {1})".format('a', 1), number=1000000)
format took 35% longer. I may be mistaken but think they undepricated it for that reason.– mhlester
Jan 22 '14 at 1:16
timeit(lambda: "('%s', %d)" % ('a', 1), number=1000000)
timeit(lambda: "('{0}', {1})".format('a', 1), number=1000000)
It finally clicked what you want and what your input is! It's for arbitrary length columns object! Here ya go:
return_string = "(" + ', '.join((repr(column) for column in columns)) + ")"
Output is exactly as requested:
('a', 1)
All previous answers (including my deleted one), were assuming a fixed two-item input. But reading your code (and wading through the indent corruption), I see you want any columns
object to be represented
columns
you can create a function to represent your type correctly
def toStr(x):
if isinstance(x, int):
return str(x)
#another elif for others types
else:
return "'"+x+"'"
and use
myTuple = ('a', 1, 2, 5)
print "("+", ".join(toStr(x) for x in myTuple)+")"
to print in correct format
You cant concatenate (or join) integers with strings. Also repeated string concatenations like that are really slow. repr
will put quots around strings but not integers, while turning them both into strings. Try this:
repr
return "(%s)," % ", ".join(repr(c) for c in columns)
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Actually
%
is deprecated – usestr.format
instead.– Konrad Rudolph
Jan 21 '14 at 23:39