Unique_ptr: pointer being freed was not allocated when emplaced in list
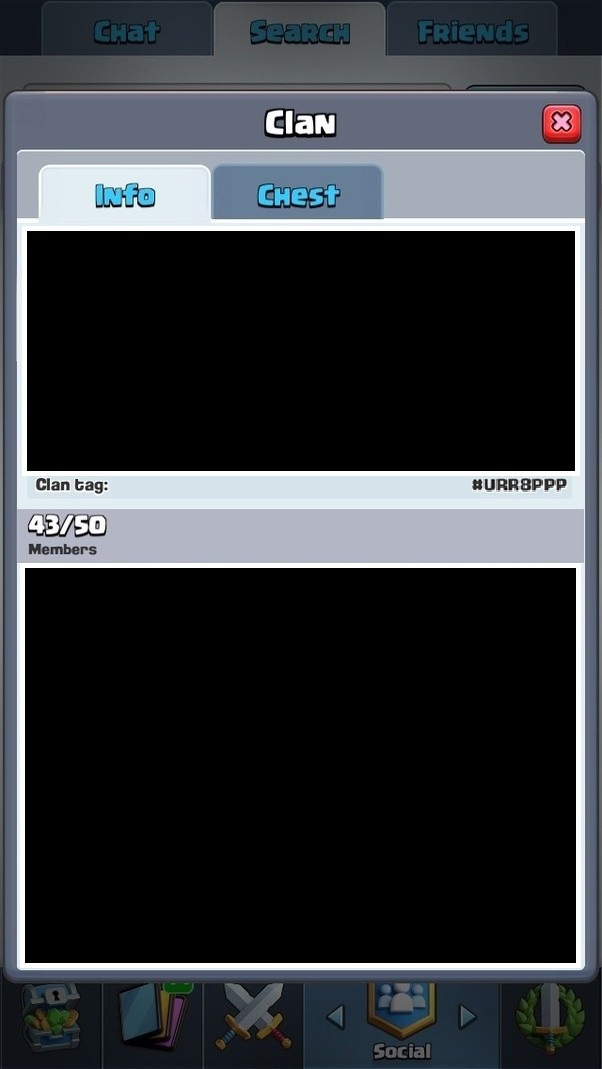

Unique_ptr: pointer being freed was not allocated when emplaced in list
I have a simple C++ program like so:
#include <iostream>
#include <list>
#include <memory>
int main(void) {
std::list<std::unique_ptr<int>> l;
int x = 5;
// throws runtime error: pointer being freed was not allocated
l.emplace_back(&x);
}
When the program is run I obtain the following output:
a.out(59842,0x7fffb13d1380) malloc: *** error for object 0x7ffee81489ac: pointer being freed was not allocated
*** set a breakpoint in malloc_error_break to debug
[1] 59842 abort ./a.out
a.out(59842,0x7fffb13d1380) malloc: *** error for object 0x7ffee81489ac: pointer being freed was not allocated
*** set a breakpoint in malloc_error_break to debug
[1] 59842 abort ./a.out
This tells me that during the destruction of the list the unique_ptr object that had been created is being destroyed, and during that destruction the pointer to x
is being freed when it was never malloc
ed.
x
malloc
However I would expect that the same would occur in this situation where a unique_ptr
falls out of scope and is destroyed
:
unique_ptr
destroyed
#include <iostream>
#include <list>
#include <memory>
int main(void) {
int x = 5;
// does not throw runtime error when falling out of scope and being destructed
std::unique_ptr<int> p(&x);
}
However when the unique_ptr p
above falls out of scope and is destroyed the program runs fine. Why is the first program giving me an error when destroying the unique_ptr
but the second one is not? Why does emplacing the unique_ptr
in a list cause its destruction to fail?
unique_ptr p
unique_ptr
unique_ptr
2 Answers
2
I think what happened is that the variable p
in your second example got just optimized away and thus not resulted in a run-time error.
p
But the error which occurs is clear, it is trying to free a memory which was allocated on a stack. Note that smart pointers are usually used in conjunction with heap allocated memory, not a memory allocated on a stack.
@bipll That's a great point actually :-)
– ProXicT
3 mins ago
I've copied your code and compiled it:
$ g++ omg.cpp && ./a.exe
Aborted (core dumped)
You may try to add another level of indirection:
int main(void) {
int x = 5;
{
std::unique_ptr<int> p(&x);
}
}
and see if it helps.
gcc-8.1 compiles the snippet just fine
– ProXicT
4 mins ago
Sure it does as does my 5.3...what happens when you run the binary?
– bipll
3 mins ago
I didn't run in because godbolt only allows compiling the code but not running it. But even if I ran that, it would throw the same error, as freeing a memory allocated on stack is just a no-no.
– ProXicT
1 min ago
That is not a level of indirection.
– Lightness Races in Orbit
1 min ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You probably can make it work correctly with stack-allocated objects using a no-op deleter...just out of curiosity.
– bipll
5 mins ago