Confusion on C++ return reference from function
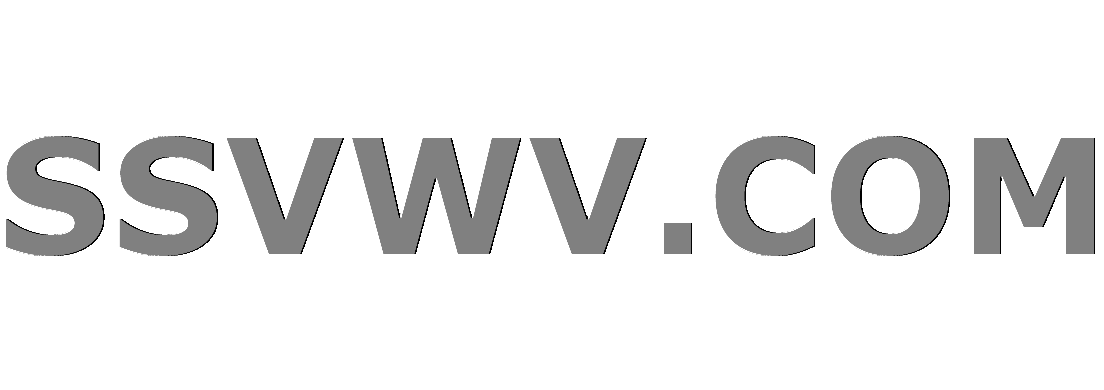
Multi tool use
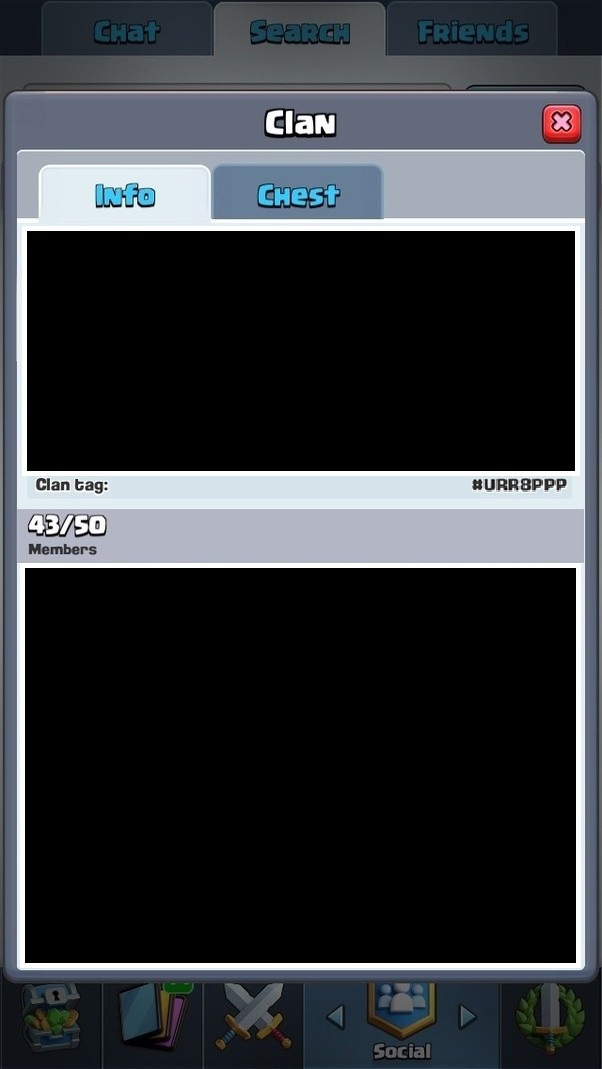

Confusion on C++ return reference from function
I have been having some confusion on the reference in C++, so I did a little experiment with the following function
std::vector<float> & fun(std::vector<float> & x) {
return x;
}
Then I call the function using the following two ways:
std::vector<float> x(10000);
std::vector<float> result1 = fun(x);
std::vector<float> & result2 = fun(x);
Now the variable result2 is indeed a reference of x, but result1 seems to be a copy of x instead of a reference. So here I am confused:
Why can I declare a non-reference variable to be the return value of a function that returns a reference?
Does C++ changes my returned reference to a non-reference variable because my declaration of result1 is not a reference?
3 Answers
3
There's no real change happening.
What happens is that a reference refers to something, and when you use the reference in an expression, you (usually) get what it refers to. So, if I have something like:
int x = 1;
int &rx = x;
std::cout << rx;
What gets printed out is the value of x
--the reference gets dereferenced to get the value, and that's what gets printed out. Likewise, if I had something like:
x
int x = 1;
int y = 2;
int rx = x;
int ry = y;
int z = rx + ry;
...the compiler is going to dereference rx
and ry
to get the values of x
and y
. Then it adds those together, and assigns the result to z
.
rx
ry
x
y
z
A reference is an alias for some object. Under most circumstances (essentially anything other than initializing another reference) using the reference in an expression uses the object that the reference refers to, not the reference itself.
That's basically the same as
std::vector<float> x(10000);
std::vector<float> & result2 = x;
std::vector<float> result1 = result2;
The language allows it since result2
is a reference of x
, each mention of result2
is the same as x
. You could see the reference as being an alias, result2
is an alias of x
.
result2
x
result2
x
result2
x
Since fun(x)
simply accepts a reference to x
and returns it, your code (on the left) is equivalent to the code on the right:
fun(x)
x
a std::vector<float> x(10000); a std::vector<float> x(10000);
std::vector<float> result1 = fun(x); std::vector<float> result1 = x;
std::vector<float> &result2 = fun(x); std::vector<float> &result2 = x;
In other words, result1
becomes a copy of x
, while result2
becomes a reference to x
. That means result1
is distinct from x
, while result2
and x
refer to the same underlying data.
result1
x
result2
x
result1
x
result2
x
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.