A generic interface with type constraints accepting a default type
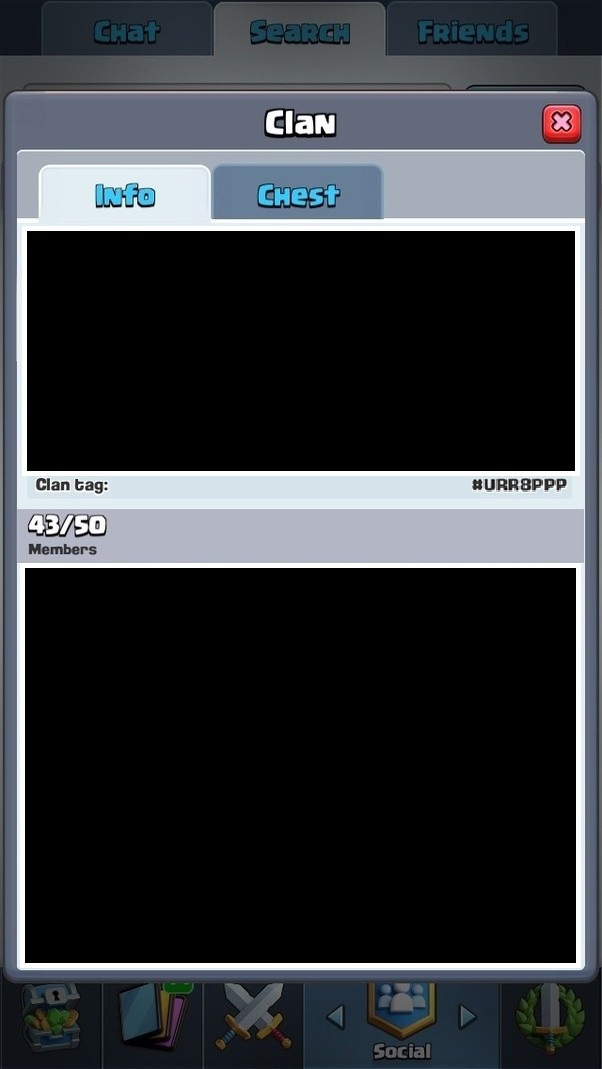

A generic interface with type constraints accepting a default type
I've created a simple generic interface in typescript
interface DateAdapter<T> {
clone(): T;
}
and I have a simple class which implements said interface
class StandardDateAdapter implements DateAdapter<StandardDateAdapter> {
clone: () => StandardDateAdapter;
}
I then have a generic Options
interface which accepts a type extending DateAdapter
. By default, I'd like the type argument to be StandardDateAdapter
so I set it up like so:
Options
DateAdapter
StandardDateAdapter
interface Options<T extends DateAdapter<T> = StandardDateAdapter> {
until?: T;
}
Unfortunately, typescript doesn't like this and is throwing an error
Type 'StandardDateAdapter' does not satisfy the constraint 'DateAdapter<T>'.
Types of property 'clone' are incompatible.
Type '() => StandardDateAdapter' is not assignable to type '() => T'.
Type 'StandardDateAdapter' is not assignable to type 'T'.
Any ideas how I can work around this? Is this a bug? Thanks!
note: DateAdapter
is only generic because I want the return type of clone()
to equal the type of the class implementing DateAdapter
. I had initially tried clone(): this;
in the DateAdapter, but typescript was smart enough to realize that StandardDateAdapter#clone() returning new StandardDateAdapter
!== this
DateAdapter
clone()
DateAdapter
clone(): this;
new StandardDateAdapter
this
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.