Unable to receive String Data from Python Socket to Java Socket
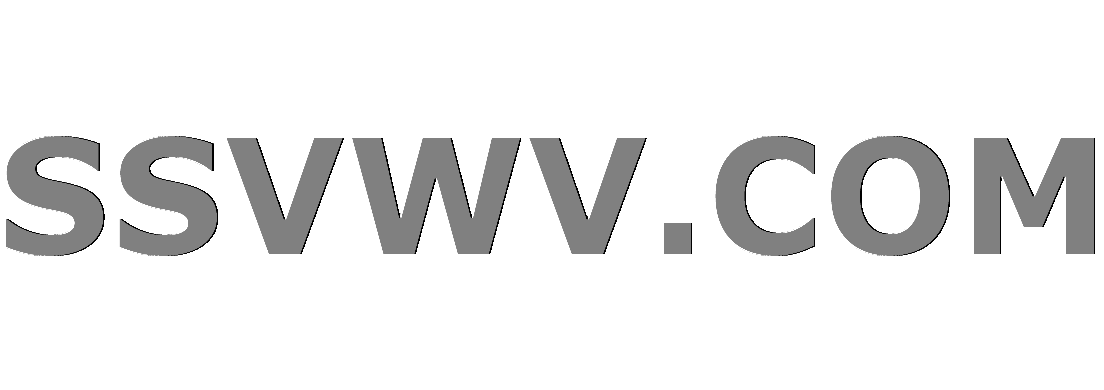
Multi tool use
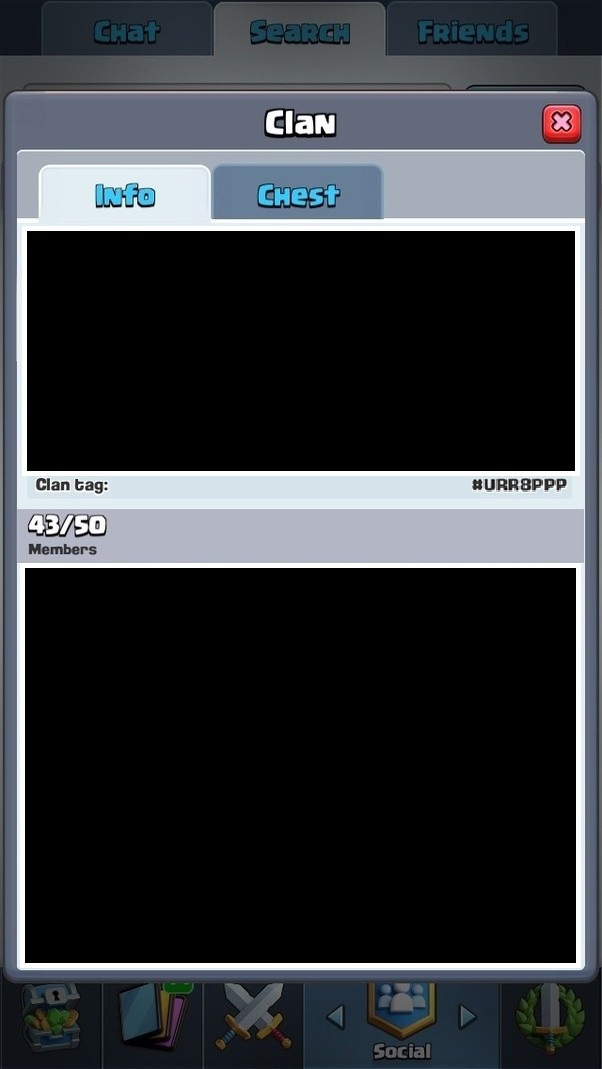

Unable to receive String Data from Python Socket to Java Socket
I am having trouble with using sockets
As you can see the codes worked when I tried to send string from JAVA to PYTHON.
But however I am having trouble when I tried to send string from PYTHON to JAVA ,which is the opposite way. And I need it to convert into bytes and decode it since I encoded the string before I send it over.
Thus the problem now is how or is there anything wrong in my codes when I send a string from Python socket and receiving the string by Java Socket?
I really need help, thank you!
Python (SERVER) Codes:
import socket
import ssl
import hashlib
import os
from Crypto.Cipher import AES
import hashlib
from Crypto import Random
sHost = ''
sPort = 1234
def bindSocket():
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) #IPv4 and TCP
try:
s.bind((sHost,sPort))
print("Socket created and binded")
except socket.error as msgError:
print(msgError)
print("Error in Binding Socket")
return s #so that we can use it
def socketConnect():
s.listen(1) #listen to 1 connection at a time
while True:
try:
conn, address = s.accept() #Accept connection from client
print ("Connected to: " + address[0] + ":" +str(address[1]))
except socket.error as error:
print ("Error: {0}" .format(e))
print ("Unable to start socket")
return conn
def loopCommand(conn):
while True:
passphrase = "Hello Java Client "
data = conn.recv(1024)#receive the message sent by client
print(data)
conn.send(passphrase.encode('utf-8'))
print("Another String is sent to Java")
s = bindSocket()
while True:
try:
conn = socketConnect()
loopCommand(conn)
except:
pass
Java (Client) Codes:
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.net.UnknownHostException;
public class SocketSTesting {
public Socket socketStartConnect() throws UnknownHostException, IOException {
String ip = "192.168.1.16";
int port = 1234;
Socket clientSocket = new Socket(ip, port);
if (clientSocket.isConnected()) {
System.out.println("It is connected to the server which is " + clientSocket.getInetAddress());
} else if (clientSocket.isClosed()) {
System.out.println("Connection Failed");
}
return clientSocket;
}
public void sendString(String str) throws Exception {
// Get the socket's output stream
Socket socket = socketStartConnect();
OutputStream socketOutput = socket.getOutputStream();
byte strBytes = str.getBytes();
// total byte
byte totalByteCombine = new byte[strBytes.length];
System.arraycopy(strBytes, 0, totalByteCombine, 0, strBytes.length);
//Send to Python Server
socketOutput.write(totalByteCombine, 0, totalByteCombine.length);
System.out.println("Content sent successfully");
//Receieve Python string
InputStream socketInput = socket.getInputStream();
String messagetype = socketOutput.toString();
System.out.println(messagetype);
}
public static void main(String args) throws Exception {
SocketSTesting client = new SocketSTesting();
String str = "Hello Python Server!";
client.sendString(str);
}
}
But meanwhile, you don't seem to be even trying to read anything from the inputstream. Instead, you're just converting the output stream to a string and printing that. That's why you're not seeing any data from Python.
– abarnert
47 mins ago
1 Answer
1
You seem to think that String messagetype = socketOutput.toString();
performs I/O. It doesn't, so printing it or even calling it does nothing and proves nothing. You need to read from the socket input stream.
String messagetype = socketOutput.toString();
BTW clientSocket.isConnected()
cannot possibly be false at the point you are testing it. If the connect had failed, an exception would have been thrown. Similarly, clientSocket.isClosed()
cannot possibly be true at the point you are testing it, because you haven't closed the socket you just created. Further, if isClosed()
was true it would not mean 'connection failed', and isConnected()
being false does not entail isClosed()
being true. Remove all this.
clientSocket.isConnected()
clientSocket.isClosed()
isClosed()
isConnected()
isClosed()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I don't know if this is your problem, but it's certainly a problem. TCP is not a stream of messages, it's just a stream of bytes. So how is Java supposed to know when you've sent your whole string? It's like expecting Java to knowwhereawordendswhenyoudon'tputanyspaces. You need some kind of framing, like maybe a newline at the end of the string, so it knows to read until a newline.
– abarnert
49 mins ago