Mock imports to test function logic
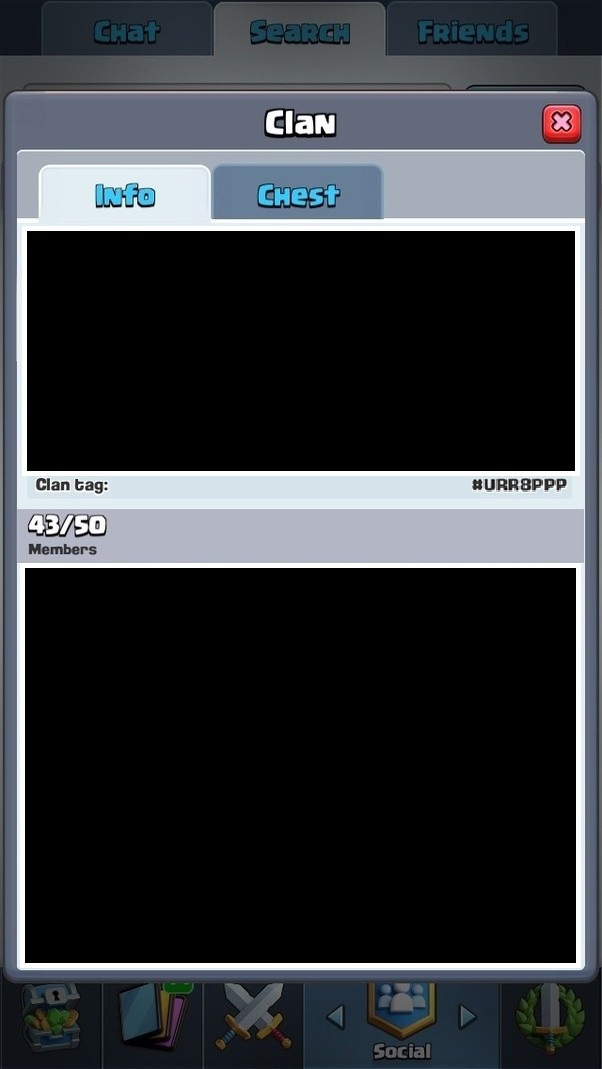

Mock imports to test function logic
Lets say I have the following file.
This file imports promiseFunction
from a library and I'd like to test the doSomething()
function.
promiseFunction
doSomething()
In particular, assert the states when the promise resolves or fails
// file: MyComponent.js
import promiseFunction from 'somewhere';
class MyClass extends Component {
doSomething = () => {
promiseFunction()
.then((data) => {
this.setState({...this.state, name: data.name});
}.catch(() => {
this.setState({...this.state, error: 'error'});
});
}
}
How do I mock the promiseFunction
thats being imported. Or really just any function that's being imported.
promiseFunction
// file: MyClass.spec.js
it('sets error in state when doSomething() is rejected', () => {
const wrapper = shallow(<MyClass />);
// How do I mock promiseFunction here?
wrapper.instance().doSomething();
expect(wrapper.state().error).toEqual('error');
});
1 Answer
1
You can use spyOn in combination with mockResolvedValue and mockRejectedValue
Here is a simple example that stubs the default export of lib.js:
// ---- lib.js ----
const libFunc = () => {
return Promise.resolve(1);
}
export default libFunc;
// ---- app.js ----
import libFunc from './lib';
const useLibFunc = () => {
return libFunc();
}
export default useLibFunc;
// ---- app.test.js ----
import useLibFunc from './app';
// import * so we can mock the 'default' export
import * as lib from './lib';
describe('useLibFunc', () => {
it('should normally resolve to 1', () => {
return useLibFunc().then((result) => {
expect(result).toBe(1);
});
});
it('should resolve to 2 when lib is stubbed', () => {
// use 'default' to mock the default export
const stub = jest.spyOn(lib, 'default').mockResolvedValue(2);
return useLibFunc().then((result) => {
expect(result).toBe(2);
stub.mockReset();
});
});
it('should fail when lib is stubbed to reject', () => {
// use 'default' to mock the default export
const stub = jest.spyOn(lib, 'default')
.mockRejectedValue(new Error('something bad happened'));
return useLibFunc().catch((error) => {
expect(error.message).toBe('something bad happened');
stub.mockReset();
});
});
});
In your case you would need to do something like this:
// ---- MyComponent.js ----
import promiseFunction from 'somewhere';
class MyClass extends Component {
doSomething = () => {
// return the promise so we can use it in the test
return promiseFunction()
.then((data) => {
this.setState({...this.state, name: data.name});
}.catch(() => {
this.setState({...this.state, error: 'error'});
});
}
}
// ---- MyClass.spec.js ----
import * as somewhere from 'somewhere';
it('sets error in state when doSomething() is rejected', () => {
// use 'default' to mock the default export
const stub = jest.spyOn(somewhere, 'promiseFunction')
.mockRejectedValue(new Error('something bad happened'));
const wrapper = shallow(<MyClass />);
// return the promise so the test waits for it to resolve
return wrapper.instance().doSomething().then(() => {
expect(wrapper.state().error).toEqual('error');
});
});
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.