Django - detect which form was submitted in a page
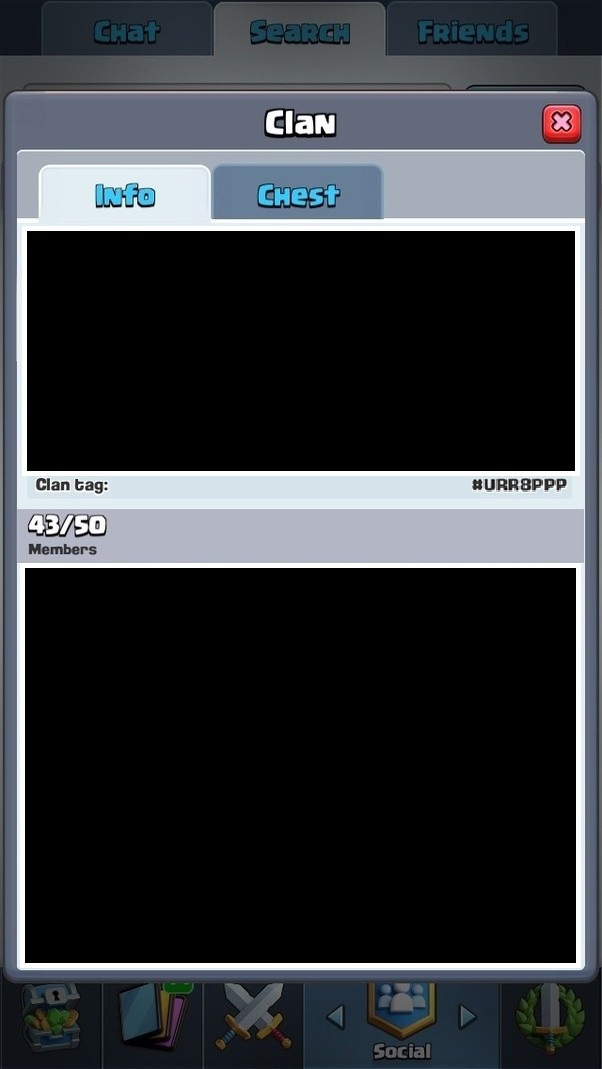

Django - detect which form was submitted in a page
I have multiple form fields, and multiple submit buttons on a same page. I want to detect by which form field a POST request is triggered.
I am using CBV
and my post()
method has this:
CBV
post()
def post(self, request, **kwargs):
....
form1 = Form1(request.POST, instance=Model1.objects.filter(some_filtering...)
form2 = Form2(request.POST, instance=Model2.objects.filter(some_filtering...)
form3 = Form3(request.POST, instance=Model3.objects.filter(some_filtering...)
form4 = Form4(request.POST, instance=Model4.objects.filter(some_filtering...)
# this is the code I want to know
if POST request is triggered by form1...
# do something....
return super().post(request, **kwargs)
return super().post(request, **kwargs)
How can I detect which form triggered POST request?
2 Answers
2
you can use an hidden input in your form like this
<input name="formId" value="1" type="hidden"/>
... <!-- in second form --!>
<input name="formId" value="2" type="hidden"/>
then in your view check which form submitted
if request.POST.get("formId") == "1":
form1 = Form1(request.POST, instance=Model1.objects.filter(some_filtering...)
elif request.POST.get("formId") == "2":
form1 = Form2(request.POST, instance=Model1.objects.filter(some_filtering...)
...
I did it by using the input
tag in HTML template instead of button
tag for submitting the form
input
button
<form name="form-1" method="POST" action="{% url 'view_url_name'%}">
{% csrf_token %}
{{ form }}
<!--use input tag for submit button -->
<input class="btn mt-3 btn-primary" name="form-1-submit" value="Submit" type="submit"/>
</form>
Note: use the different name for each form submit input.
Now in your view, you can simply check for the name
attribute of the button.
name
if 'form-1-submit' in request.POST:
form1 = Form1(request.POST, instance=Model1.objects.filter(some_filtering...)
...
# and so on
This implementation will also cover the scenario where you are
submitting the same form
from different buttons to use that form
for different
purposes.
form
form
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.