Sort array by committee and then by user role
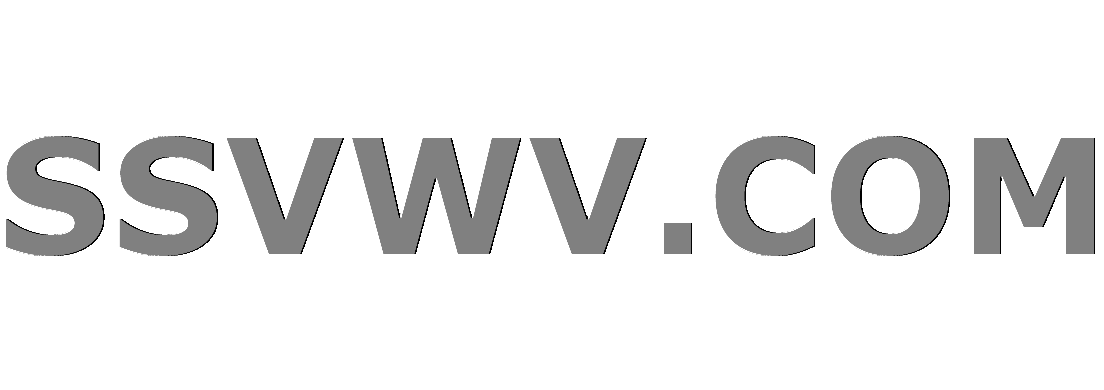
Multi tool use
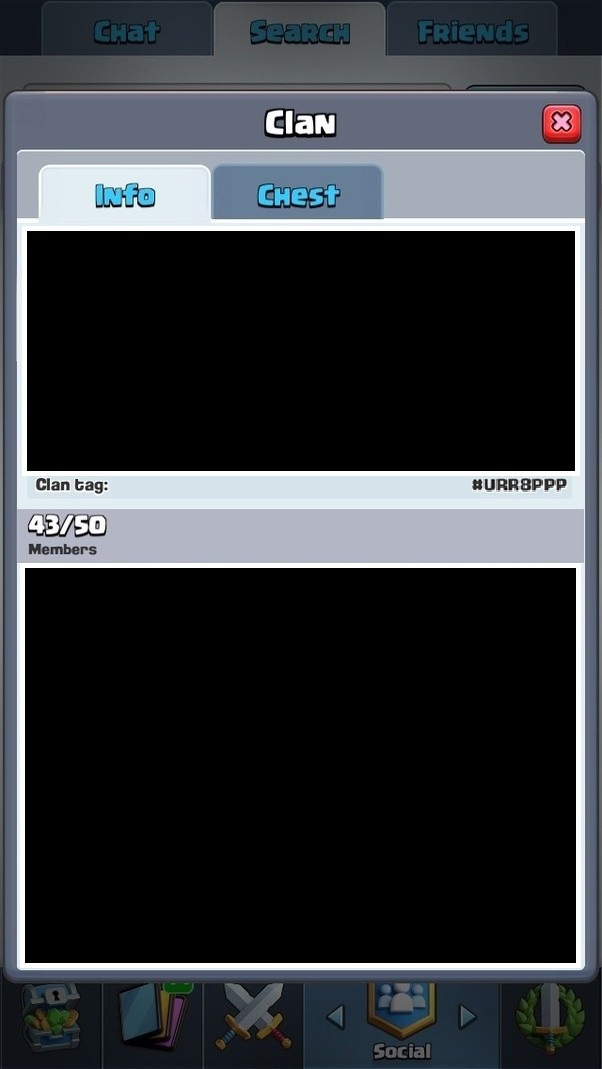

Sort array by committee and then by user role
I have an array that contains data similar to this:
var committees = [
{
committee: "Apple Com",
userRole: "Member",
membersNum: 11
},
{
committee: "Orange Com",
userRole: "Member",
membersNum: 9
}, {
committee: "Banana Com",
userRole: "",
membersNum: 13
}, {
committee: "Strawberry Com",
userRole: "Vice President",
membersNum: 15
}, {
committee: "Lemon Com",
userRole: "",
membersNum: 13
},
{
committee: "Mango Com",
userRole: "Chair",
membersNum: 11
},
]
I want them sorted first, by the User Role and then by the Committee. The Committee field will always have a value, while the User Role field sometimes will be null.
Currently, what I am doing to achieve this is break the array into two arrays. The first array will return only items which User Role is not null. The second array returns items which User Role is null. Next, I sort each array by Committee ascendingly and then I concatenate them. Thus the logic goes like this:
//get committees user is member of and committees user is not member of
var memberOf = ;
var nonMemberOf = ;
for(var i=0; i<committees.length; i++){
if(committees[i].userRole){
memberOf.push(committees[i]);
} else {
nonMemberOf.push(committees[i]);
}
}
memberOf.sort(function(a,b){return (a.committee > b.committee) ? 1 : -1; });
nonMemberOf.sort(function(a,b){return (a.committee > b.committee) ? 1 : -1; });
var allComs = memberOf.concat(nonMemberOf);
I've spent more than 3 hours reading and trying to implement what I read on some posts here:
I believe that there is a better way doing what I'm doing but just can't figure it out. If someone can shed some light here, I will appreciate that very much.
P.S. I'm not asking for someone to do this for me, I want to understand how to do it. For those who can help, thanks in advance!
1 Answer
1
Try sort
ing by the Boolean difference between each compared item's userRole
, or if that comes out to 0
(that is, if both have a role or both don't have a role), then sort by their committee
s:
sort
userRole
0
committee
var committees=[{committee:"Apple Com",userRole:"Member",membersNum:11},{committee:"Orange Com",userRole:"Member",membersNum:9},{committee:"Banana Com",userRole:"",membersNum:13},{committee:"Strawberry Com",userRole:"Vice President",membersNum:15},{committee:"Lemon Com",userRole:"",membersNum:13},{committee:"Mango Com",userRole:"Chair",membersNum:11},]
committees.sort((a, b) => (
Boolean(b.userRole) - Boolean(a.userRole)
|| a.committee.localeCompare(b.committee)
));
console.log(committees);
This certainly does the trick. I believe then, that I will need to understand more in detail how the sort() works and also the localeCompare()
– Morfinismo
1 min ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
also please share the array
– brk
20 mins ago