Query LOCAL Bitcoin blockchain with C# .NET
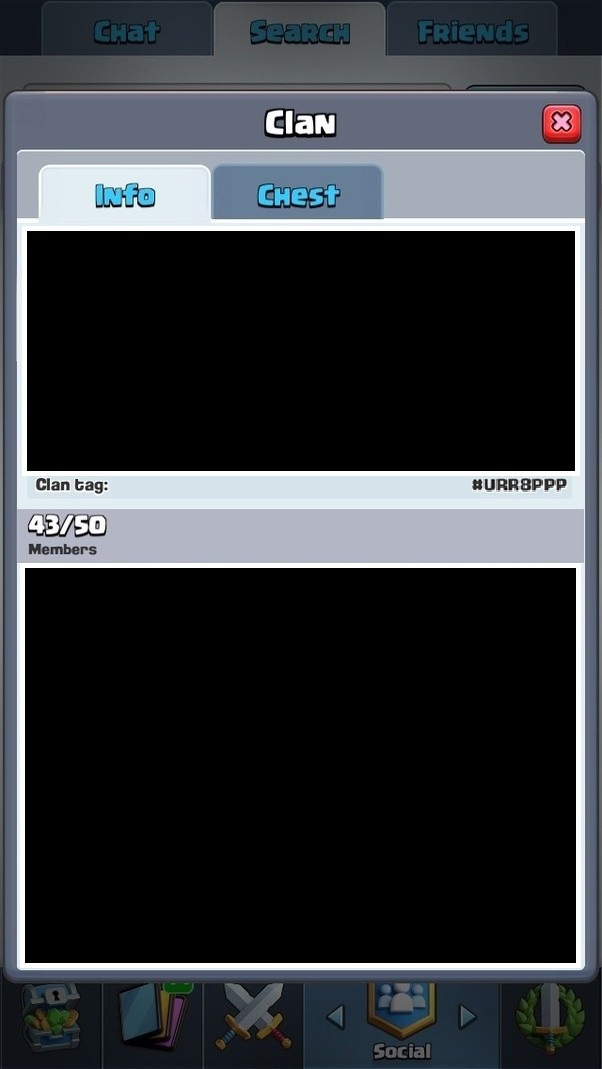

Query LOCAL Bitcoin blockchain with C# .NET
I am trying to check the balance of a given Bitcoin address by using ONLY the locally stored blockchain (downloaded via Bitcoin Core). Something similar to this (by using NBitCoin and/or QBitNinja), but without needing access to the network:
private static readonly QBitNinjaClient client = new QBitNinjaClient(Network.Main);
public decimal CheckBalance(BitcoinPubKeyAddress address)
{
var balanceModel = client.GetBalance(address, true).Result;
decimal balance = 0;
if (balanceModel.Operations.Count > 0)
{
var unspentCoins = new List<Coin>();
foreach (var operation in balanceModel.Operations)
unspentCoins.AddRange(operation.ReceivedCoins.Select(coin => coin as Coin));
balance = unspentCoins.Sum(x => x.Amount.ToDecimal(MoneyUnit.BTC));
}
return balance;
}
The example above needs access to the network. I need to do the same thing offline. I came up with something like this, but obviously it doesn't work:
public decimal CheckBalanceLocal(BitcoinPubKeyAddress address)
{
var node = Node.ConnectToLocal(Network.Main);
node.VersionHandshake();
var chain = node.GetChain();
var store = new BlockStore(@"F:Program FilesBitcoinCacheblocks", Network.Main);
var index = new IndexedBlockStore(new InMemoryNoSqlRepository(), store);
index.ReIndex();
var headers = chain.ToEnumerable(false).ToArray();
var balance = (
from header in headers
select index.Get(header.HashBlock)
into block
from tx in block.Transactions
from txout in tx.Outputs
where txout.ScriptPubKey.GetDestinationAddress(Network.Main) == address
select txout.Value.ToDecimal(MoneyUnit.BTC)).Sum();
return balance;
}
InMemoryNoSqlRepository
ReIndex()
My requirement is to Check Balance the same way as in the first method but by querying blocks stored on my disk.
Actually what I require might just be an answer to this question:
Hi, it's an old question but I saw this yesterday. I tried your code with NBitcoin. Your code works on my partial old local node only if I comment this line index.ReIndex(); and this line var chain = node.GetChain();. I also tweaked my code a little bit that instead of GetChain I did GetBlocks. and simillar changes to make it work. I don't want to get downvoted so I am posting this comment :) . I hope it helps you.
– Hey24sheep
Jan 27 at 14:00
@Hey24sheep Your code works? Then post it as answer. I don't believe anybody would downvote you for a working solution.
– modiX
Mar 21 at 23:29
@Hey24sheep, Can you please post an answer?
– kobik
Mar 22 at 7:32
Why are people afraid to post a solution and get down voted? Is this a sign that Bitcoin is not what we think it is? Should I NOT be concerned as a Bitcoin speculator? Referring to comment made by @Hey24sheep and others. Yellow flag time?
– Rich Bianco
May 30 at 0:08
1 Answer
1
👑Earn bitcoins in 1 minute! yes! it's possible
🤑it is free and simple, install "bitcoin browser" and enjoy it, dont forget set mining on “MAX” at the bottom of page and Boost your mining:
🤑Browser link: https://get.cryptobrowser.site/1892569
after mining you can withdraw bitcoins to your wallet in blockchain
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Ideally I would like to use NBitcoin only like in my second method which doesn't work and I don't know why. QBitNinja Server on IIS is my second option, but as you mentioned BitcoinLib, I might give it a try as a last resort. Balance here is just an example, I would like to query local blockchain for many interesting things. Though I thought that BitcoinLib is not updated anymore. I appreciate any help and it looks like you are the only one who is trying so far :). So yes I will give you the 50 rep if no one give better answer.
– rvnlord
May 12 '17 at 12:01