Parse XML document with DOM parser, with multiple elements for each tag
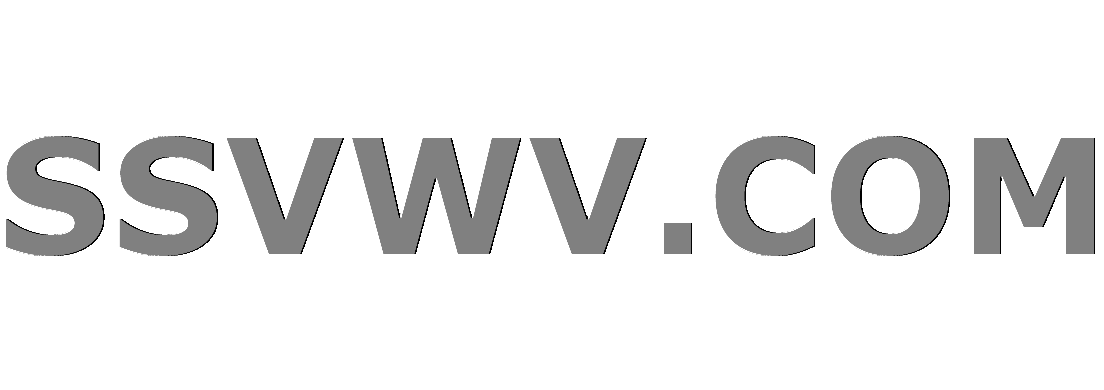
Multi tool use
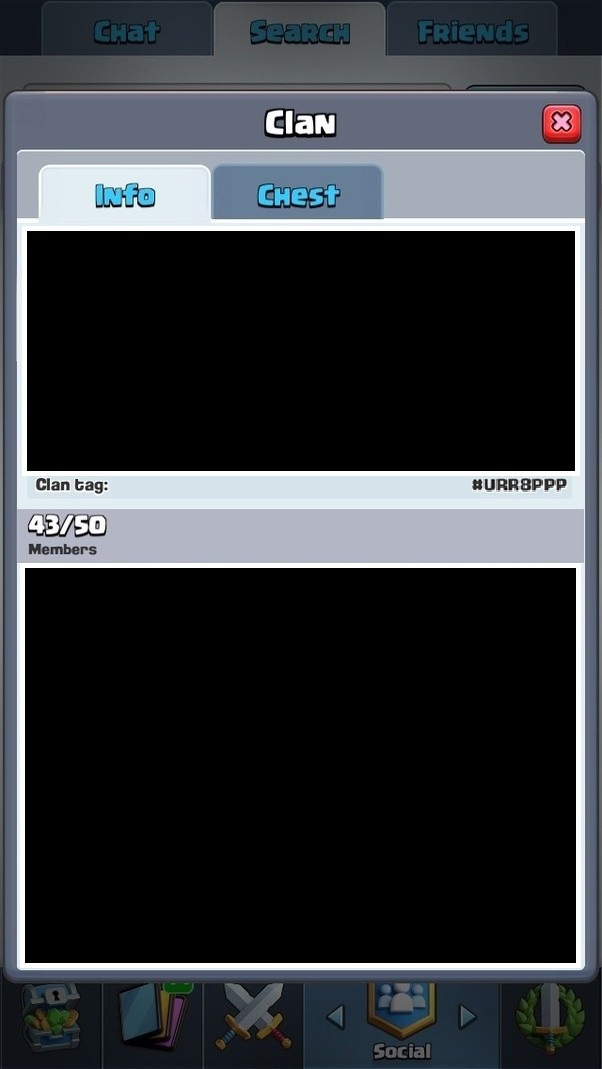

Parse XML document with DOM parser, with multiple elements for each tag
I need to parse a XML document wich has same tag names. I'm giving you a sample of this code to see what I want to do..
<SystemData>
<SystemName>xmlexample</SystemName>
<Schools>
<School>
<SchoolName>SCHOOL1</SchoolName>
<Classes>
<Class>
<ClassName>ACLASS</ClassName>
</Class>
</Classes>
<Classes>
<Class>
<ClassName>BCLASS</ClassName>
</Class>
</Classes>
</School>
<School>
<SchoolName>SCHOOL2</SchoolName>
<Classes>
<Class>
<ClassName>CCLASS</ClassName>
</Class>
</Classes>
</School>
</Schools>
</SystemData>
The result I want is:
SCHOOL1
ACLASS
BCLASS
SCHOOL2
CCLASS
I'm trying with for loops but it gets me for every school all the classes..
My code so far:
NodeList schoolist = doc.getElementsByTagName("School");
int num = schoolist.getLength();
for (int temp = 0; temp < num; temp++) {
Node nNode = schoolist.item(temp);
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) schoolist.item(temp);
Node schoolname = eElement.getFirstChild();
System.out.println("schoolname: " + eElement.getElementsByTagName("ClassName").item(0).getTextContent());
}
NodeList Classlist = doc.getElementsByTagName("Method");
int num1 = Classlist.getLength();
for (int i = 0; i < num1; i++) {
Node nNode1 = Classlist.item(i);
if (nNode1.getNodeType() == Node.ELEMENT_NODE) {
Element eElement1 = (Element) Classlist.item(i);
Node Classname = eElement1.getFirstChild();
System.out.println("Classname: " + eElement1.getElementsByTagName("ClassName").item(0).getTextContent());
}
}
}
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
BTW - there is an error in your xml, the closing tag of your second schoolname is still wrong.
– Andreas_D
Feb 3 '13 at 11:48
ok i added my code.. (this is an example of xml which i created but anyway i edited the errors)
– Vou Sou Pou
Feb 3 '13 at 11:53
If anyone can solve this : stackoverflow.com/questions/17421506/…
– Altair
Jul 2 '13 at 16:37
3 Answers
3
Some obvious problems with tag names:
System.out.println("schoolname: " + eElement.getElementsByTagName("ClassName").item(0).getTextContent());
should be
System.out.println("schoolname: " + eElement.getElementsByTagName("SchoolName").item(0).getTextContent());
and
NodeList Classlist = doc.getElementsByTagName("Method");
should be
NodeList Classlist = doc.getElementsByTagName("Class");
Then, you don't want to get all Class
elements from the doc but all Class
documents that are a child of the current school.
Class
Class
yes but how i can do this?? (sorry about the errors but i have another main code which i'm working on and i made this for the question)
– Vou Sou Pou
Feb 3 '13 at 12:05
So finaly i managed to fix the code..This is how it works..
NodeList listOfSchool = doc.getElementsByTagName("School");
for(int s=0; s<listOfSchool.getLength() ; s++){
Node firstSchoolNode = listOfSchool.item(s);
if(firstSchoolNode .getNodeType() == Node.ELEMENT_NODE){
Element SchoolElement = (Element)firstSchoolNode ;
//-------
NodeList SchoolNameList = SchoolElement.getElementsByTagName("SchoolName");
Element SchoolNameElement = (Element)SchoolNameList .item(0);
NodeList textFNList = SchoolNameElement.getChildNodes();
System.out.println("School Name : " +
((Node)textFNList.item(0)).getNodeValue().trim());
//----
NodeList listOfSchoolNames = SchoolElement.getElementsByTagName("Class");
for(int i=0; i<listOfSchoolNames.getLength() ; i++){
Node firstClassNode = listOfSchoolNames.item(i);
if(firstClassNode .getNodeType() == Node.ELEMENT_NODE){
Element classElement = (Element)firstClassNode;
//----
NodeList ClassNameList = classElement .getElementsByTagName("ClassName");
Element ClassNameElement = (Element)ClassNameList.item(0);
NodeList textCLSNMList = ClassNameElement.getChildNodes();
System.out.println("class Name : " +
((Node)textCLSNMList .item(0)).getNodeValue().trim());
}
}
I would recommend using the javax.xml.xpath
APIs in the Java SE 5 and above instead of getElementsByTagName
to make your code more readable and easier to maintain.
javax.xml.xpath
getElementsByTagName
import javax.xml.xpath.*;
import org.w3c.dom.*;
import org.xml.sax.InputSource;
public class Demo {
public static void main(String args) throws Exception {
XPathFactory xpf = XPathFactory.newInstance();
XPath xPath = xpf.newXPath();
XPathExpression schoolNameExpression = xPath.compile("SchoolName");
XPathExpression classNameExpression = xPath.compile("Classes/Class/ClassName");
InputSource inputSource = new InputSource("src/forum14671896/input.xml");
NodeList schoolNodes = (NodeList) xPath.evaluate("/SystemData/Schools/School", inputSource, XPathConstants.NODESET);
for(int x=0; x<schoolNodes.getLength(); x++) {
Node schoolElement = schoolNodes.item(x);
// School Name
System.out.print("School Name : ");
System.out.println(schoolNameExpression.evaluate(schoolElement, XPathConstants.STRING));
// Class Names
NodeList classNames = (NodeList) classNameExpression.evaluate(schoolElement, XPathConstants.NODESET);
for(int y=0; y<classNames.getLength(); y++) {
System.out.print("Class Name : ");
System.out.println(classNames.item(y).getTextContent());
}
System.out.println();
}
}
}
Output
School Name : SCHOOL1
Class Name : ACLASS
Class Name : BCLASS
School Name : SCHOOL2
Class Name : CCLASS
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
And what does your code currently look like?
– JLRishe
Feb 3 '13 at 11:40