Angular 6 - Cannot Process data from web api
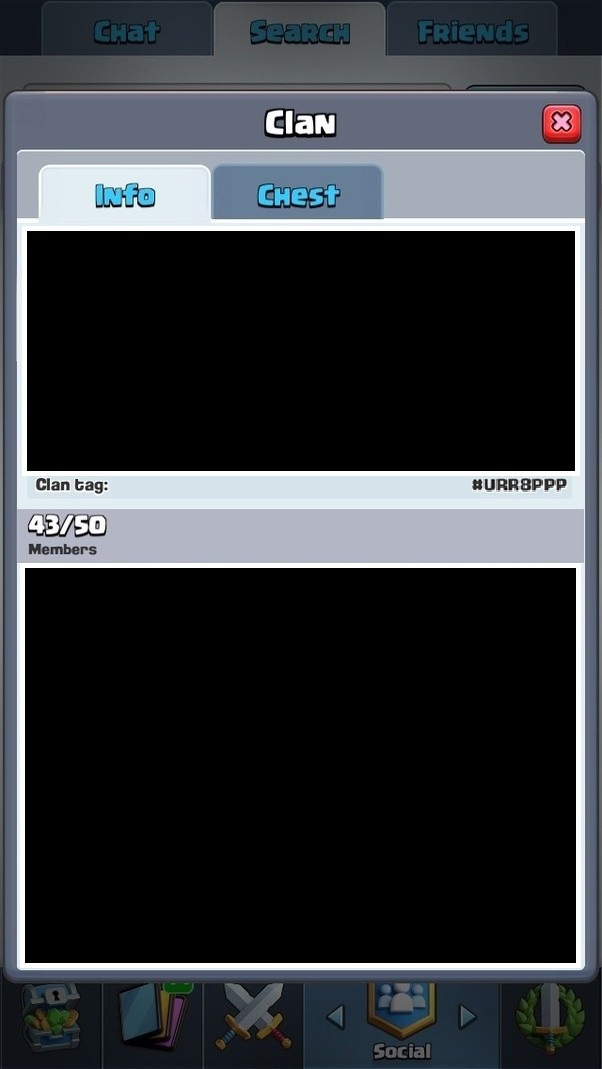

Angular 6 - Cannot Process data from web api
I am attempting to process some data from an api in Angular 6. However, even though I can see in Network tab that the data below is being returned, I cannot process the data after this call completes.
My data returned by service:
{"profile": "German DJ and producer based in Berlin. He is the founder of a label."}
My fetch:
public fetchData(): Observable<DiscogsRecord> {
return this.http.get(this.url).pipe(
map(response => response["profile"]),
catchError(this.errorHandler("Error loading music data.", ))
);
}
My interface:
export interface DiscogsRecord {
profile: string;
}
My ngOnInit:
ngOnInit() {
this.recs = ;
this.dataService.fetchData().subscribe(records => (this.recs = records));
console.log(this.recs);
... etc
When I log this.recs, I get no data, just an empty array: . What am I doing wrong?
map(response:DiscogsRecord => response.profile)
2 Answers
2
The problem in where you have your logging.
this.dataService.fetchData().subscribe(records => (this.recs = records));
console.log(this.recs);
Http requests are asynchronous, so when you call fetchData, it returns an observable and then executes your log statement. At that point it has NOT yet retrieved the data. When the data is retrieved, it calls the code within your subscribe.
So you need to move your logging INSIDE your subscribe.
this.dataService.fetchData().subscribe(records =>
{
this.recs = records;
console.log(this.recs);
});
Well, of course they're asynchronous. :( It's been working all along. Thanks!
– Scott
8 mins ago
Just return the response in your service,
return this.http.get(this.url).pipe(
map(response => response)
and you need to access within the subscribe
this.dataService.fetchData().subscribe((records) => {
this.recs = records;
console.log(this.recs[0].profile);
});
why the downvote
– Sajeetharan
27 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Have you tried this
map(response:DiscogsRecord => response.profile)
– Alf Moh
38 mins ago