setState doesn't update the state immediately
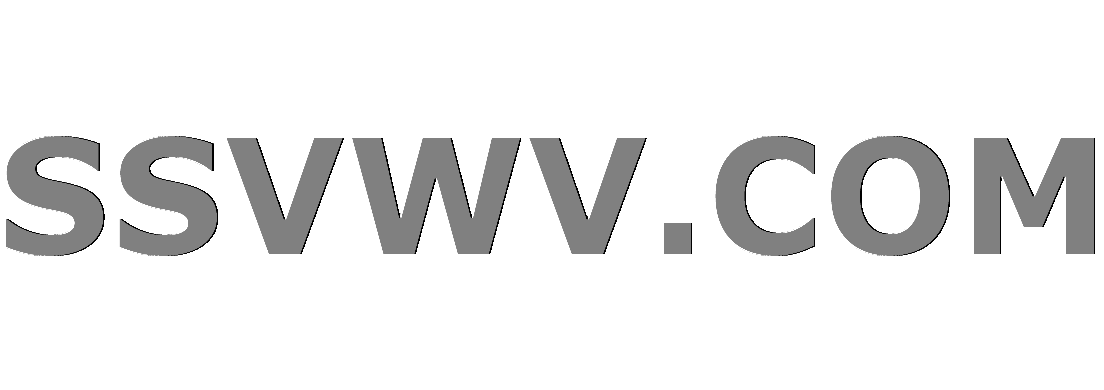
Multi tool use
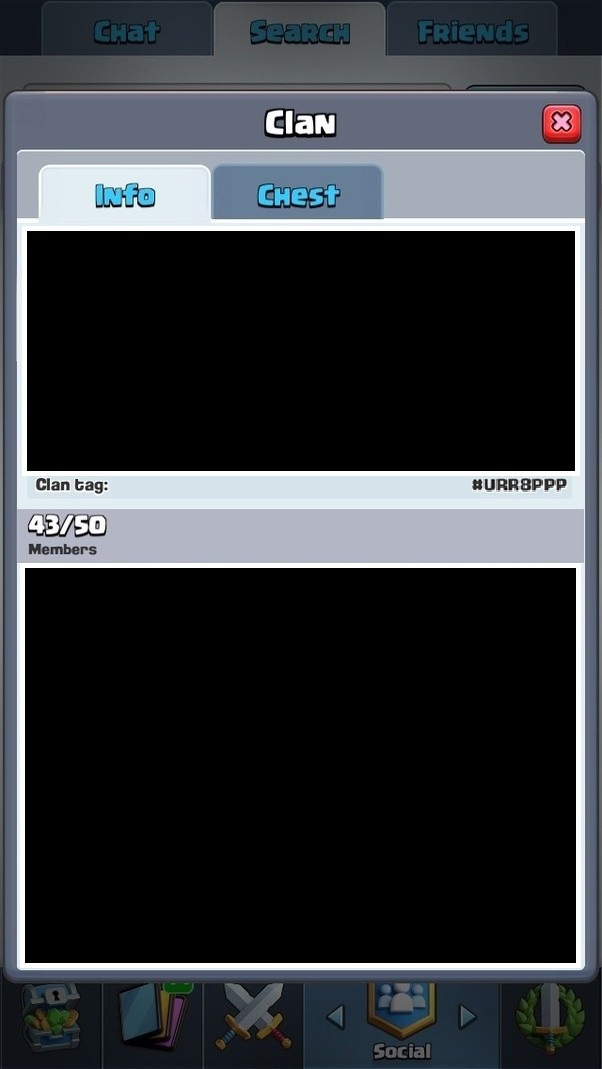

setState doesn't update the state immediately
I would like to ask why my state is not changing when I do an onclick event. I've search a while ago that I need to bind the onclick function in constructor but still the state is not updating. Here's my code:
import React from 'react';
import Grid from 'react-bootstrap/lib/Grid';
import Row from 'react-bootstrap/lib/Row';
import Col from 'react-bootstrap/lib/Col';
import BoardAddModal from 'components/board/BoardAddModal.jsx';
import style from 'styles/boarditem.css';
class BoardAdd extends React.Component {
constructor(props){
super(props);
this.state = {
boardAddModalShow: false
}
this.openAddBoardModal = this.openAddBoardModal.bind(this);
}
openAddBoardModal(){
this.setState({ boardAddModalShow: true });
// After setting a new state it still return a false value
console.log(this.state.boardAddModalShow);
}
render() {
return (
<Col lg={3}>
<a href="javascript:;" className={style.boardItemAdd} onClick={this.openAddBoardModal}>
<div className={[style.boardItemContainer,style.boardItemGray].join(' ')}>
Create New Board
</div>
</a>
</Col>
)
}
}
export default BoardAdd
4 Answers
4
Your state needs some time to mutate, and since console.log(this.state.boardAddModalShow)
executes before the state mutates, you get the previous value as output. So you need to write the console in the callback to the setState function
console.log(this.state.boardAddModalShow)
openAddBoardModal(){
this.setState({ boardAddModalShow: true }, function () {
console.log(this.state.boardAddModalShow);
});
}
setState
is asynchronous. It means you can’t call setState on one line and assume state has changed on the next.
setState
according to React docs
setState()
does not immediately mutate this.state
but creates a
pending state transition. Accessing this.state
after calling this
method can potentially return the existing value. There is no
guarantee of synchronous operation of calls to setState and calls may
be batched for performance gains.
setState()
this.state
this.state
Why would they make setState async
This is because setState alters the state and causes rerendering. This
can be an expensive operation and making it synchronous might leave
the browser unresponsive.
Thus the setState calls are asynchronous as well as batched for better
UI experience and performance.
Since setSatate is a asynchronous function so you need to console the state as a callback like this.
openAddBoardModal(){
this.setState({ boardAddModalShow: true }, () => {
console.log(this.state.boardAddModalShow)
});
}
This actually help me
openAddBoardModal(){
this.setState({ boardAddModalShow: true },()=>{
console.log(this.state.boardAddModalShow)
});
}
setState takes a callback. Fortunately!! This is where we get updated state.
this.setState(
{ name: "Mustkeom" },
() => {
console.log(this.state.name) // Mustkeom
}
);
So When callback fires, this.state is the updated state. You can get mutated/updated data in callback.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Thanks Man! This one works
– Sydney Loteria
Dec 22 '16 at 8:14