Deleting all consonants from a string using recursion in c++
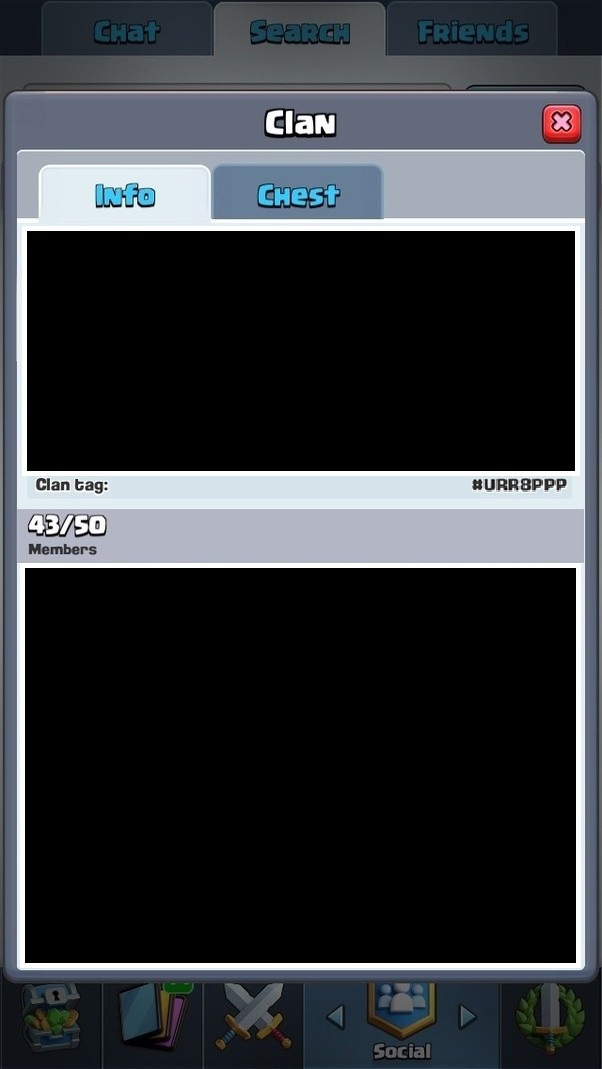

Deleting all consonants from a string using recursion in c++
I'm almost completely new to programming and I try to learn C++. This is the first task where I feel like I hit a wall. I tried to search but because people usually use a loop to solve the problem I could not find anything.
I tried to find a recursive solution to delete all consonants from a string (I think I know how to solve it using loops, but wanted to expand my knowledge on recursion).
#include <iostream>
#include <string>
using namespace std;
int i = 0;
string s("");
string del_cons(string z){
if(i == (z.length()-1) ){
s+= z.substr(i);
return s;
}
else if(z[i] == 'a' || z[i] == 'e' || z[i] == 'i' || z[i] == 'o' || z[i] == 'u'){
i++;
s+= del_cons(z.substr(i));
return s;
}
else{
s+= z.substr(i,1);
i++;
s+= del_cons(z.substr(i));
return s;
}
}
int main(){
string x;
getline(cin, x);
cout << del_cons(x) << endl;
return 0;
}
The code compiles, but when trying it out with a string, I get this error message:
terminate called after throwing an instance of 'std::out_of_range'
what(): basic_string::substr: __pos (which is 3) > this->size() (which is 2)
Abgebrochen (Speicherabzug geschrieben)
When playing around with the code, this line seems to be the problem:
s+= del_cons(z.substr(i));
Could somebody give me a hint what I'm doing wrong? It seems I'm misusing the substr() function, but I don't know how. Thanks a lot.
1 Answer
1
Sorry but I could not resist...
#include <string>
#include <iostream>
#include <algorithm>
int main()
{
std::string s;
std::getline( std::cin, s );
s.erase( std::remove_if( s.begin(),
s.end(),
(unsigned char x){ return ! ( x == 'a' || x == 'e' || x == 'i' || x == 'o' || x == 'u' ); }
),
s.end()
);
std::cout << s << std::endl;
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.