One to many table relationship
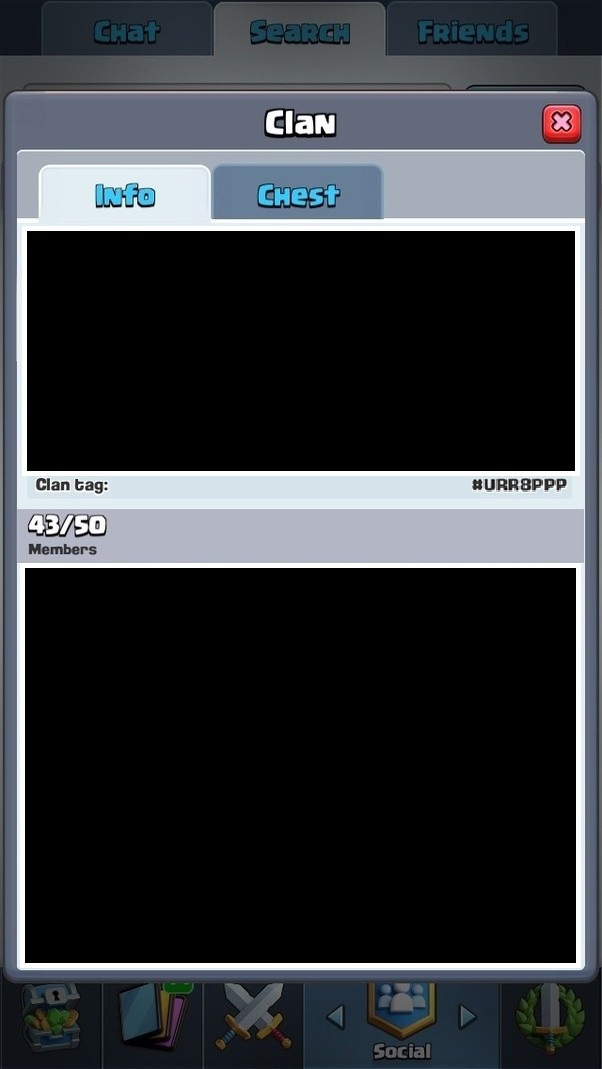

One to many table relationship
I want to implement one to many table relationship with JPA:
Main table Merchants
@Entity
@Table
public class Merchants {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "id", updatable = false, nullable = false)
private int id;
@Column
private String name;
@Column
private String login;
}
Second table Terminals which holds merchant id
@Entity
@Table
public class Terminals {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "id", updatable = false, nullable = false)
private int id;
@Column
private int merchant_id;
@Column
private String mode;
}
I tried this code implementation:
@Entity
@Table
public class Merchants {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "id", updatable = false, nullable = false)
private int id;
@Column
private String name;
@OneToMany(mappedBy="id")
private List<Terminals> terminals;
@Column
private String login;
}
@Entity
@Table
public class Terminals {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "id", updatable = false, nullable = false)
private int id;
@ManyToOne(fetch=FetchType.LAZY)
@JoinColumn(name="id")
private Merchants merchants;
@Column
private int merchant_id;
@Column
private String mode;
}
I tried to implement this code but I don't understand how I can store only the merchant_id for reference. What is the proper way to implement this?
This doesn't make sense. The unique ID of the terminal can't also be the join column referencing the merchant ID. Otherwise, you couldn't have several terminals for a merchant. Ang mappedBy is supposed to hold the name of the property which is annotated by ManyToOne. Why don't you simply read the documentation? docs.jboss.org/hibernate/orm/current/userguide/html_single/…
– JB Nizet
31 mins ago
This is the desired result - several terminals for one merchant.
– Peter Penzov
27 mins ago
You haven't read my comment. I know that this is what is desired. I understand what OneToMany does. The problem is precisely that you don't, since you're using the unique ID of a terminal as a foreign key to a merchant.
– JB Nizet
21 mins ago
Can you post official answer with code example, please?
– Peter Penzov
19 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Possible duplicate of Spring Data one to many mapping
– horatius
31 mins ago