Textbox not updating when static object method to update property is called
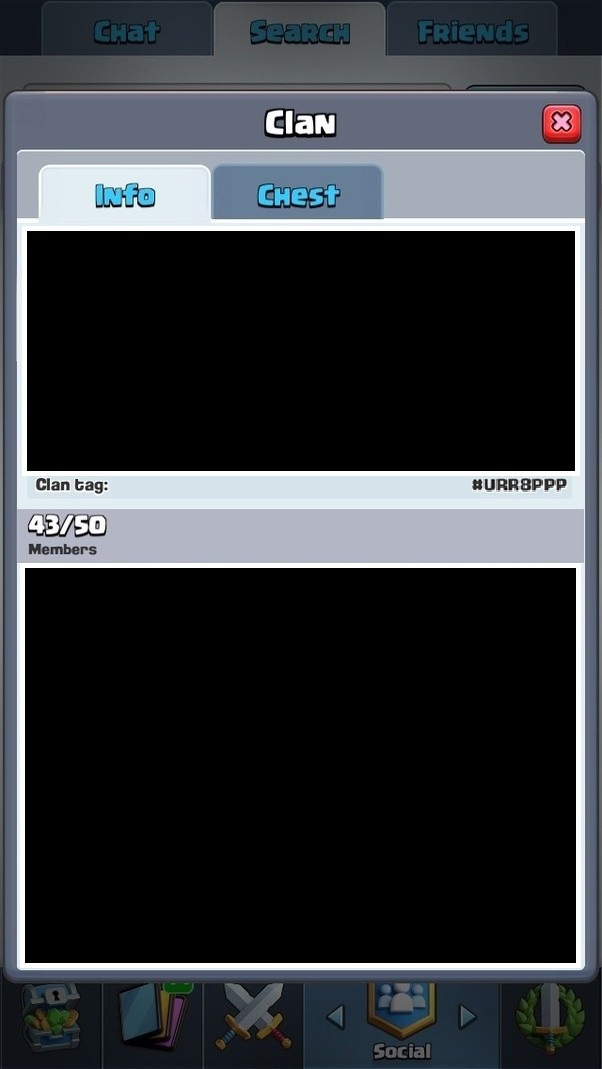

Textbox not updating when static object method to update property is called
First, let me state I am new to C# altogether. At the moment I am working on a program, and have been trying to get something that I thought would be rather simple to do. This is apparently not the case. I'll show here a very small example of what I have been trying to do. This is functionally identical, but without all the extra things that I have in the real project. We have 3 files here.
MainWindow.xaml
<Window.Resources>
<CollectionViewSource x:Key="data1ViewSource" d:DesignSource="{d:DesignInstance {x:Type local:Data1}, CreateList=True}"/>
</Window.Resources>
<Grid>
<Button x:Name="TestButton" Content="Button" HorizontalAlignment="Left" Margin="78,25,0,0" VerticalAlignment="Top" Width="75" Click="TestButton_Click"/>
<TextBox x:Name="testStringTextBox" HorizontalAlignment="Left" Height="24" Margin="78,86,0,309" Grid.Row="0" Text="{Binding TestString}" VerticalAlignment="Center" Width="120"/>
<TextBlock x:Name="testStringTextBlock" HorizontalAlignment="Left" Margin="78,143,0,0" TextWrapping="Wrap" Text="{Binding TestString}" VerticalAlignment="Top" Height="181" Width="245"/>
</Grid>
MainWindow.xaml.cs
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void TestButton_Click(object sender, RoutedEventArgs e)
{
Class1.TheButton();
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
System.Windows.Data.CollectionViewSource data1ViewSource = ((System.Windows.Data.CollectionViewSource)(this.FindResource("data1ViewSource")));
// Load data by setting the CollectionViewSource.Source property:
// data1ViewSource.Source = [generic data source]
}
}
Class1.cs
public static class Class1
{
private static int Inc = 1;
public static Data1 testData = new Data1("Starting testn");
public static void TheButton()
{
testData.TestString += "Test number " + Inc.ToString() + ".n";
Inc++;
}
}
public class Data1 : INotifyPropertyChanged
{
public Data1(string input)
{
TestString = input;
}
private string _testString;
public event PropertyChangedEventHandler PropertyChanged;
public string TestString
{
get { return _testString; }
set { _testString = value; OnPropertyChanged("TestString"); }
}
protected void OnPropertyChanged(string name)
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(name));
}
}
}
The program compiles as is, without error, and when I set a breakpoint, I can view the object Class1.testData, and I can see the proper value in Class1.testData.TestString and _testString.
I have spent several days searching for some sort of simple example of what I'm trying to do here, and I have yet been able to find any solution to get it actually working. I've come to the conclusion that I must be doing something wrong or just plain missing something, but I also cannot find anything to give me insight on what that is.
1 Answer
1
Try setting your binding mode to OneWay.
<Window.Resources>
<CollectionViewSource x:Key="data1ViewSource" d:DesignSource="{d:DesignInstance {x:Type local:Data1}, CreateList=True}"/>
</Window.Resources>
<Grid>
<Button x:Name="TestButton" Content="Button" HorizontalAlignment="Left" Margin="78,25,0,0" VerticalAlignment="Top" Width="75" Click="TestButton_Click"/>
<TextBox x:Name="testStringTextBox" HorizontalAlignment="Left" Height="24" Margin="78,86,0,309" Grid.Row="0" Text="{Binding TestString, Mode="OneWay"}" VerticalAlignment="Center" Width="120"/>
<TextBlock x:Name="testStringTextBlock" HorizontalAlignment="Left" Margin="78,143,0,0" TextWrapping="Wrap" Text="{Binding TestString, Mode="OneWay"}" VerticalAlignment="Top" Height="181" Width="245"/>
</Grid>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.