Send Image with Ajax to database (php) without reloading [duplicate]
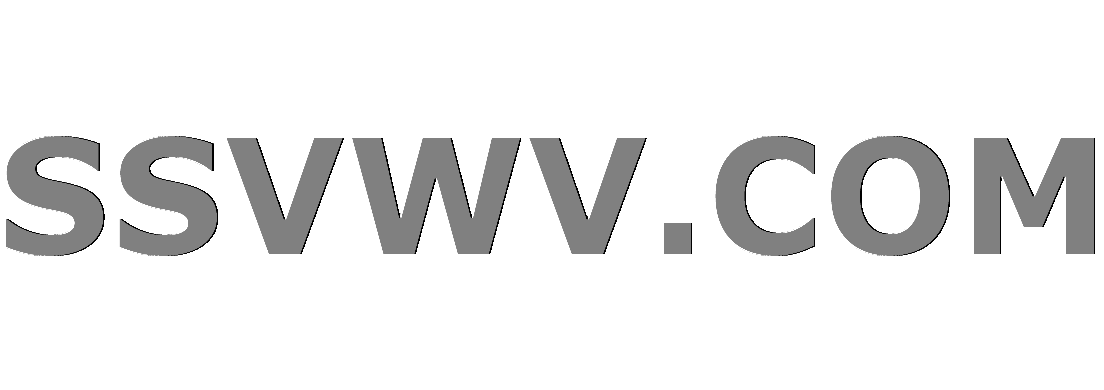
Multi tool use
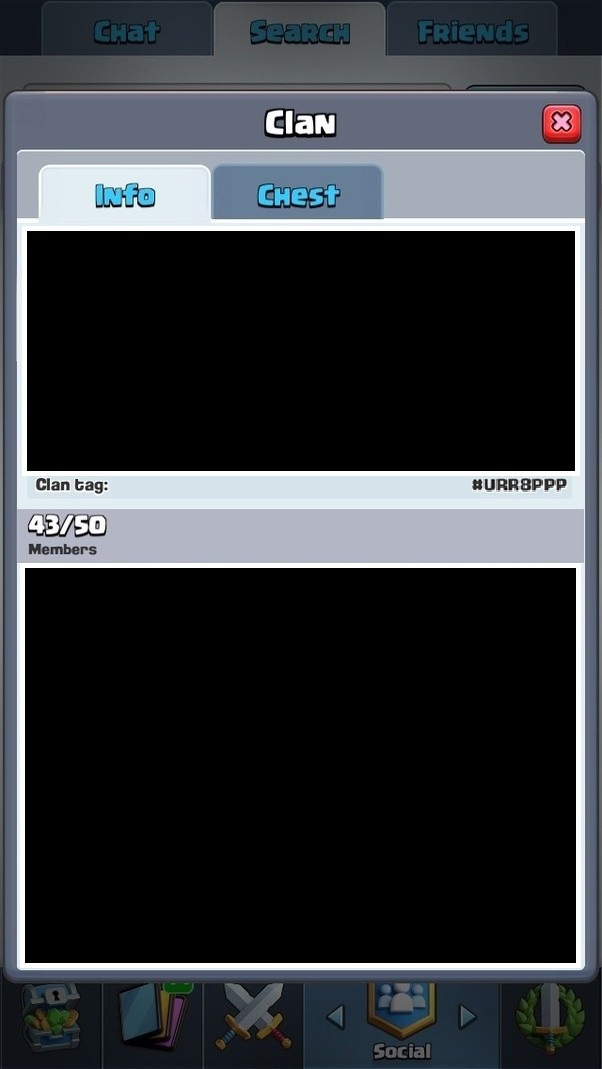

Send Image with Ajax to database (php) without reloading [duplicate]
This question already has an answer here:
I create a bootstrap modal popup form to add picture in gallery page, I developed the php & ajax code because i want without page reloading here :-
the problem is when I want to send image to database I show this error
Notice: Undefined index: photo in ....
Notice: Undefined index: photo in ....
I can't send image.
this's my ajax code
$(function() {
//twitter bootstrap script
$("button#submit").click(function(){
$.ajax({
type: "POST",
url: "image.php",
data: $('form.contact').serialize(),
success: function(msg){
$("#thanks").html(msg)
$("#myModal").modal('hide');
},
error: function(){
alert("failure");
}
});
});
});
And this's Image.php file
include 'db.php';
if(isset($_POST['titre'])){
$titre = strip_tags($_POST['titre']);
$image_name = $_FILES['photo']['name'];
$image_tmp = $_FILES['photo']['tmp_name'];
$image_size = $_FILES['photo']['size'];
$image_ext = pathinfo($image_name, PATHINFO_EXTENSION);
$image_path = '../images/'.$image_name;
$image_db_path = 'images/'.$image_name;
if($image_size < 1000000000){
if($image_ext == 'jpg' || $image_ext == 'png' || $image_ext = 'gif'){
if(move_uploaded_file($image_tmp,$image_path)){
$ins_sql = "INSERT INTO media (Images_md, Id_Pro, Value, Title) VALUES ('$image_db_path', '0', 'galerie', '$titre')";
if(mysqli_query($conn,$ins_sql)){
echo '<div class="alert alert-success alert-dismissable">
<button aria-hidden="true" data-dismiss="alert" class="close" type="button"> × </button>
Success! Well done its submitted. </div>';
}else {
echo '
<div class="alert alert-danger alert-dismissable">
<button aria-hidden="true" data-dismiss="alert" class="close" type="button"> × </button>
Error ! Change few things. </div>';
}
}else {
echo '
<div class="alert alert-danger alert-dismissable">
<button aria-hidden="true" data-dismiss="alert" class="close" type="button"> × </button>
Sorry, Image hos not been uploaded. </div>
';
}
}
else {
echo '
<div class="alert alert-danger alert-dismissable">
<button aria-hidden="true" data-dismiss="alert" class="close" type="button"> × </button>
Please Add a jpg OR png OR gif Image. </div>
';
}
}else {
echo '
<div class="alert alert-danger alert-dismissable">
<button aria-hidden="true" data-dismiss="alert" class="close" type="button"> × </button>
Image File Size is much bigger then Expect. </div>
';
}
}
and this's myModal code :
<div class="modal fade" id="myModal" role="dialog">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Ajouter Photo</h4>
</div>
<div class="modal-body">
<form class="contact">
<div class="form-group">
<label class="col-sm-2 control-label" for="inputEmail3">Titre</label>
<div class="col-sm-10">
<input type="text" name="titre" class="form-control" placeholder="Titre"/>
</div>
</div>
<br><br>
<div class="form-group">
<label class="col-sm-2 control-label" for="inputEmail3">Photo</label>
<div class="col-sm-10">
<input type="file" name="photo" class="form-control" />
</div>
</div>
</form>
</div><br><br>
<div class="modal-footer">
<button class="btn btn-success" name="submit" id="submit">submit</button>
<a href="#" class="btn btn-default" data-dismiss="modal">Close</a>
</div>
</div>
</div>
</div>
Can you help me to send image with ajax jQuery?
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
@saad Now I see, you need to send the whole form. Look at the comment above me, that is how you need to solve this. Always research first, then make a post, as this is a duplicate.
– Nytrix
Jun 30 '16 at 0:53
@RajdeepPaul The problem is I'm beginner In Ajax Language I don't know what I need to add in my code, And the big problem I need to finish my project today because I need to give it tomorrow to my teacher :'(
– saad
Jun 30 '16 at 0:56
@saad I've given an answer below. Hopefully this will solve your issue.
– Rajdeep Paul
Jun 30 '16 at 1:23
1 Answer
1
You need to make few changes in your AJAX script, such as:
Set the following options, processData: false
and contentType: false
in your AJAX request. From the documentation,
processData: false
contentType: false
contentType (default: 'application/x-www-form-urlencoded; charset=UTF-8'
)
Type: Boolean
or String
When sending data to the server, use this content type. Default is "application/x-www-form-urlencoded; charset=UTF-8", which is fine for most cases. If you explicitly pass in a content-type to $.ajax()
, then it is always sent to the server (even if no data is sent). As of jQuery 1.6 you can pass false
to tell jQuery to not set any content type header.
'application/x-www-form-urlencoded; charset=UTF-8'
Boolean
String
$.ajax()
false
and
processData (default: true
)
Type: Boolean
By default, data passed in to the data option as an object (technically, anything other than a string) will be processed and transformed into a query string, fitting to the default content-type "application/x-www-form-urlencoded". If you want to send a DOMDocument, or other non-processed data, set this option to false
.
true
Boolean
false
And if you're uploading file through AJAX, use FormData object. But keep in mind that old browsers don't support FormData object, FormData support starts from the following desktop browsers versions: IE 10+, Firefox 4.0+, Chrome 7+, Safari 5+, Opera 12+.
So change your jQuery script in the following way,
$(function(){
//twitter bootstrap script
$("button#submit").click(function(){
$.ajax({
type: "POST",
url: "image.php",
data: new FormData($('form')[0]),
processData: false,
contentType: false,
success: function(msg){
$("#thanks").html(msg)
$("#myModal").modal('hide');
},
error: function(){
alert("failure");
}
});
});
});
Thanksssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssssss Yeeeeeeeeeeeeeeeeeeeeeeeeeeeeeep It's work
– saad
Jun 30 '16 at 1:44
@saad You're very welcome! :-)
– Rajdeep Paul
Jun 30 '16 at 1:45
@Nytrix I think I need to add something to ajax code but I'm beginner in ajax language for that I make this post
– saad
Jun 30 '16 at 0:48