jQuery: eliminate white screen “pause” between animations
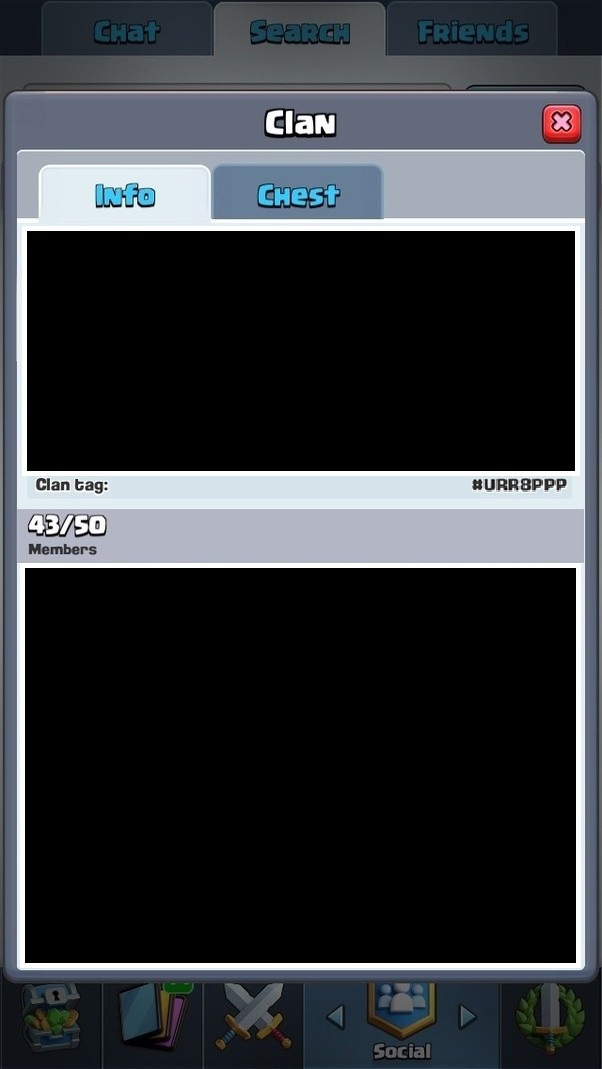

jQuery: eliminate white screen “pause” between animations
I have just discovered Barba.js and find it very useful. It provides smooth transitions between URLs of the same website.
I have put together a Plunker consisting of two pages (index.html and about.html) that are loaded smoothly, with the help of jQuery’s fadeIn()
and fadeOut()
methods.
fadeIn()
fadeOut()
$(document).ready(function() {
var transEffect = Barba.BaseTransition.extend({
start: function() {
this.newContainerLoading.then(val => this.fadeInNewcontent($(this.newContainer)));
},
fadeInNewcontent: function(nc) {
nc.hide();
var _this = this;
$(this.oldContainer).fadeOut(1000).promise().done(() => {
nc.css('visibility', 'visible');
nc.fadeIn(1000, function() {
_this.done();
});
$('html, body').animate({
scrollTop: 300
},1000);
});
}
});
Barba.Pjax.getTransition = function() {
return transEffect;
}
Barba.Pjax.start();
});
The problem with this animations is that there is a white screen interval between them.
How could I eliminate this interval, to make the transition smoother? By "smoother" I mean similar to this one (click "view case").
3 Answers
3
The white screen is the body color because you're using promise().done(..)
jquery
apply the fadeIn
after the fadeOut
finish so the body color will appear. So, this is an example sort of similar to what you have:
promise().done(..)
jquery
fadeIn
fadeOut
<style type="text/css">
.One{
width: 100%;
height: 100%;
position: absolute;
margin: 0px;
padding: 0px;
top: 0px;
left: 0px;
background-color: #476d80;
cursor: pointer;
z-index: 1;
}
.Two{
width: 100%;
height: 100%;
position: absolute;
margin: 0px;
padding: 0px;
top: 0px;
left: 0px;
background-color: #03A9F4;
cursor: pointer;
display: none;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('div.one').click(function(){
$(this).fadeOut(1000).promise().done(function(){
$('div.Two').fadeIn(1000);
});
});
});
</script>
<div class="One"></div>
<div class="Two"></div>
like you see in the example above the white screen also appears so if you don't want that just don't use promise().done(..)
.
promise().done(..)
$(document).ready(function(){
$('div.one').click(function(){
$(this).fadeOut(1000);
$('div.Two').fadeIn(1000);
});
});
you can edit your code like this:
$(this.oldContainer).fadeOut(1000).promise().done(() => {
$('html, body').animate({
scrollTop: 300
},1000);
});
nc.css('visibility', 'visible');
nc.fadeIn(1000, function() {
_this.done();
});
I have used the script you provided on this page. Click on "Studiu de caz" to see the transition. It has improved a lot indeed but the script has introduced a bug: clicking the browser's back button ruins the homepage slider.
– Razvan Zamfir
yesterday
How about using setTimeout()
to overlap the fade out and fade in? That should keep it from blanking out completely, which want to avoid.
setTimeout()
You could try something like the following:
$(document).ready(function() {
var transEffect = Barba.BaseTransition.extend({
start: function() {
this.newContainerLoading.then(val => this.fadeInNewcontent($(this.newContainer)));
},
fadeInNewcontent: function(nc) {
nc.hide();
var _this = this;
// manipulate these values
let fadeOutTime = 1000;
let fadeInTime = 1000;
let overlapTime = 100;
$(this.oldContainer).fadeOut(fadeOutTime);
// use setTimeout() to begin fadeIn before fadeOut is completely done
setTimeout(function () {
nc.css('visibility', 'visible');
nc.fadeIn(fadeInTime, function() {
_this.done();
});
$('html, body').animate({
scrollTop: 300
}, fadeInTime);
}, fadeOutTime - overlapTime)
}
});
Barba.Pjax.getTransition = function() {
return transEffect;
}
Barba.Pjax.start();
});
NOTE: This is just a stab at it, the plunker is helpful, but it's hard to see the animation in action.
UPDATE
I think you'll need something like the above, but if you want to fade in/out of black, then you'll also want to do something like create a div wrapper around all of your content within your body and give that div the background color of your app, #eff3f6, and then making the actual body background black. You'll have some work to get the exact effect you desire, but something like that or in the SO link in the comments below should help.
By "smoother" I mean similar to this one (wait 2, 3 seconds, then click "view case"). I mean, an "invisible transition".
– Razvan Zamfir
Jul 19 at 4:15
You mean that it fades in and out of black instead of white? Or one that fades in/out of a certain direction?
– Chad Moore
Jul 19 at 4:19
I am looking for the same effect as at the address I have showed you. I do not no how do do that. It might work. How do I fade to black?
– Razvan Zamfir
Jul 19 at 4:21
Yep, that is a beautiful effect. However, working a full solution to provide the exact same functional feature would exceed the purpose of StackOverflow. I would take a look at stackoverflow.com/questions/967815/…, and other similar questions on StackOverflow. This will take you in the right direction, but you'll have to put together the exact solution. Also, have you inspected the sources and the html on that page you like? You should be able to find what they're doing by inspection.
– Chad Moore
Jul 19 at 12:40
This is hat we have Plunker for.
– Razvan Zamfir
Jul 19 at 18:12
I came out with this version of the script:
$(function(){
var transEffect = Barba.BaseTransition.extend({
start: function() {
this.newContainerLoading.then(val => this.fadeInNewcontent($(this.newContainer)));
},
fadeInNewcontent: function(nc) {
var _this = this;
nc.css('visibility', 'visible');
nc.show().promise().done(() => {
$(this.oldContainer).fadeOut(50);
if (!isMobile.any()) {
$('html, body').delay(100).animate({
scrollTop: 200
},700);
}
});
}
});
Barba.Pjax.getTransition = function() {
return transEffect;
}
Barba.Pjax.start();
});
It is better, but not smooth enough. See the the effect on a real life project. How can I improve it?
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I'll take a look at it tomorrow, if you've not figured it out.
– Chad Moore
Jul 19 at 0:26