How to get an integer value from a 'for' loop in Python and print it
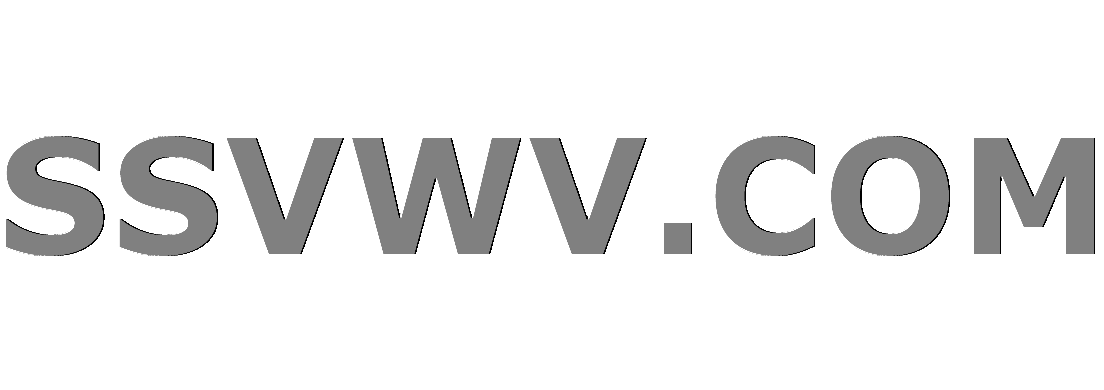
Multi tool use
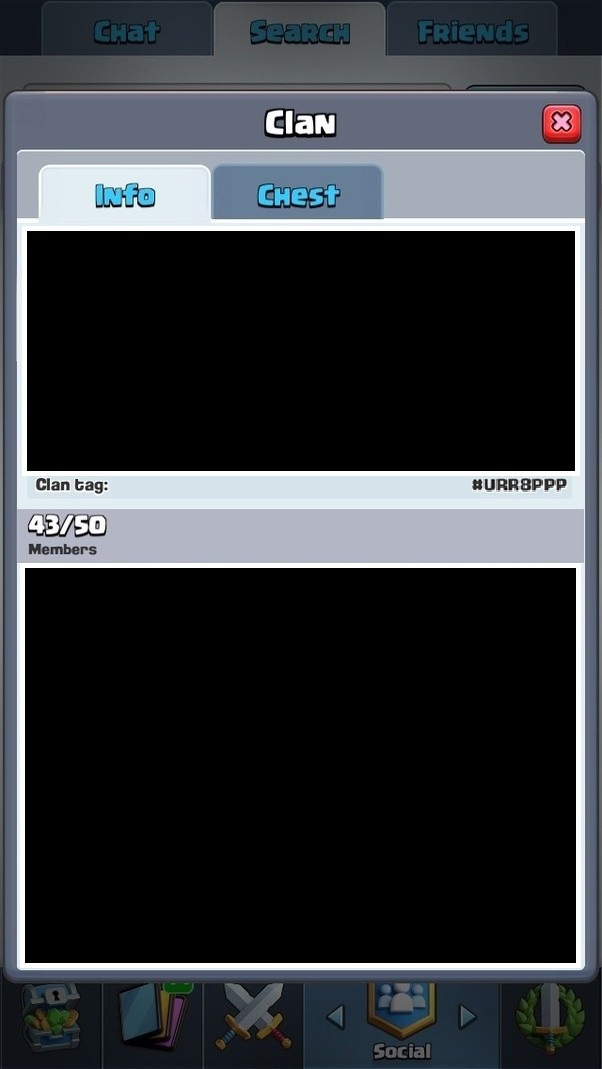

How to get an integer value from a 'for' loop in Python and print it
I am currently using Canopy to run my code. I was trying to fetch some integer data from the entry box in my GUI according to the number that I give in the for
loop and print it. It is not working.
for
Here is the error:
Traceback (most recent call last):
File "C:Program FilesEnthoughtCanopyAppappdatacanopy-1.5.5.3123.win- x86_64liblib-tkTkinter.py", line 1532, in __call__
return self.func(*args)
File "C:UsersSIMULATORDesktoppythonlife cycle graphtry4.py", line 24, in const
z=int(s)
ValueError: invalid literal for int() with base 10: ''
My code is:
from Tkinter import *
root = Tk()
root.geometry('1000x600+400+400')
def const():
const_entries =
for i in range(0, 4):
en = Entry(root)
en.pack()
en.place(x=50, y = 200 + 25*i)
s = en.get()
z = int(s)
const_entries.append(z)
j = i + 1
label = Label(root, text="Alternative %r"%j)
label.pack()
label.place(x = 200, y = 200 + 25*i)
print const_entries
button1 = Button(root, text="Construction cost", command = const).grid(row = 15, column = 0)
root.mainloop()
z=int(s)
fails because s
is an empty string. Did you miss something at s=en.get()
?– jbndlr
Dec 16 '15 at 8:09
z=int(s)
s
s=en.get()
Is there an error like
TclError: cannot use geometry manager pack inside . which already has slaves managed by grid
– WaKo
Dec 16 '15 at 8:17
TclError: cannot use geometry manager pack inside . which already has slaves managed by grid
4 Answers
4
Clarification: Assuming that s
isn't empty (en.get()
returns some number).
Check out this example:
s
en.get()
int('55.500000')
int('55.500000')
Gets you a: ValueError: invalid literal for int() with base 10: '55.500000'
ValueError: invalid literal for int() with base 10: '55.500000'
And:
float('55.500000')
float('55.500000')
Gets you:55.5
55.5
So just cast to float
instead of int
.
float
int
No. This will raise a
ValueError
because the str s
still is empty.– jbndlr
Dec 16 '15 at 8:16
ValueError
s
I clarified my answer on the first line=]
– Idos
Dec 16 '15 at 8:16
As already stated in the other answers your code is failing because your entries are empty when you call the const
method.
const
Assuming that you want to enter something in your entry boxes and then print the alternatives I guess a code like this would do the job:
from Tkinter import *
root=Tk()
root.geometry('1000x600+400+400')
def const():
const_entries =
for i in range(4):
s = entryArray[i].get()
z = int(s)
const_entries.append(z)
print const_entries
button1= Button(root,text="Construction cost", command = const).grid(row=15,column = 0)
entryArray =
for i in range(0,4):
entryArray.append(Entry(root))
#Insert default value 0
entryArray[i].insert(0, 0)
entryArray[i].pack()
entryArray[i].place(x=50,y=200+25*i)
label=Label(root,text="Alternative %d"%(i+1))
label.pack()
label.place(x=200,y=200+25*i)
root.mainloop()
This way you create the entries at startup and you give the user the time to enter something. When you press the Construction cost
button the const
function is called which will read the content of your entries and print them out.
Construction cost
const
Update
If you then want the user to enter the number of entries I'd suggest using a class and defining two buttons:
one for creating the entries after the user has entered the number
one for processing the number the user enters in the created entries
from Tkinter import *
root=Tk()
root.geometry('1000x600+400+400')
class MainWindow():
def __init__(self):
Label(root,text='Number of Entries').grid(row = 15, column = 0)
self.numEntry = Entry(root)
self.numEntry.grid(row = 15, column = 1)
self.numEntry.insert(0, 0)
Button(root,text="Build entries", command = self.buildEntries).grid(row = 15,column = 3)
Button(root,text="Construction cost", command = self.const).grid(row = 18,column = 0)
def buildEntries(self):
self.entryArray =
numEntries = int(self.numEntry.get())
for i in range(numEntries):
self.entryArray.append(Entry(root))
#Insert default value 0
self.entryArray[i].insert(0, 0)
self.entryArray[i].pack()
self.entryArray[i].place(x=50,y=200+25*i)
label=Label(root,text="Alternative %d"%(i+1))
label.pack()
label.place(x=200,y=200+25*i)
def const(self):
const_entries =
numVal = len(self.entryArray)
for i in range(numVal):
s = self.entryArray[i].get()
z = int(s)
const_entries.append(z)
print 'Const Entries:', const_entries
m = MainWindow()
root.mainloop()
Thanks for the answer..it really helped..i just want to add one more question here...What if i want the user to input the number of Entry boxes first and then take the user input in those entries into a list for further calculation...
– Saif uddin
Dec 16 '15 at 12:33
You mean to create the entries based on an user input and then having the user fill these entries with values?
– toti08
Dec 16 '15 at 12:40
yes..that way it be more dynamic i think...
– Saif uddin
Dec 16 '15 at 12:43
I would really appreciate it, if you could help me make it..!
– Saif uddin
Dec 16 '15 at 12:44
the No. of Entries should be input by user in an Entry box..!
– Saif uddin
Dec 16 '15 at 12:47
When literal s
couldn't be converted to int
, int(s)
failed.
s
int
int(s)
try:
z = int(s)
except ValueError:
z = 0
So you want that your code works wrong? Because
0
is not the value which is expected during the runtime.– Psytho
Dec 16 '15 at 9:29
0
When you call en.get()
at this point, it will return ''
and then int('')
will raise an error. Maybe before getting the integer you want to set an integer as string to the entry
.
en.get()
''
int('')
entry
When you write button1 = Button(root, text="Construction cost", command = const).grid(row = 15, column = 0)
button1
will not be a Button
instance; button1
should be the result of the grid
function.
button1 = Button(root, text="Construction cost", command = const).grid(row = 15, column = 0)
button1
Button
button1
grid
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
It is obvious that i need to convert the Entry into integer to save it into the list but the program shows error as soon as i declare the Entry as Integer,
– Saif uddin
Dec 16 '15 at 8:09