Get file size given file descriptor in Go
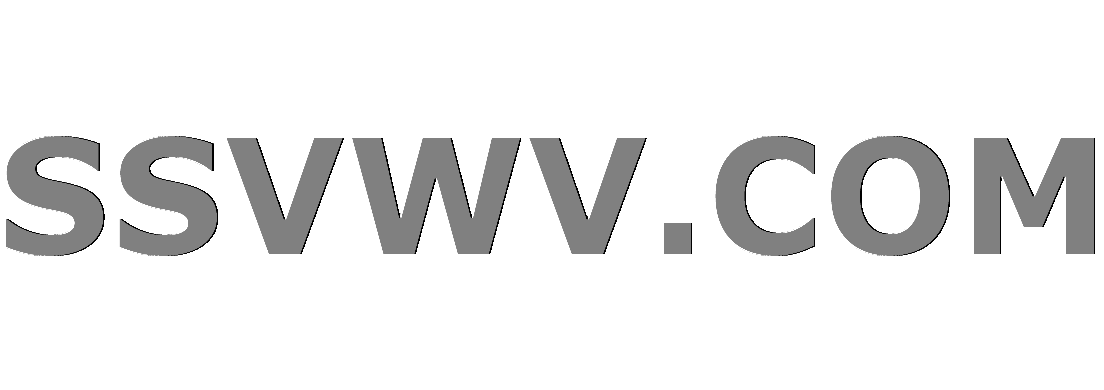
Multi tool use
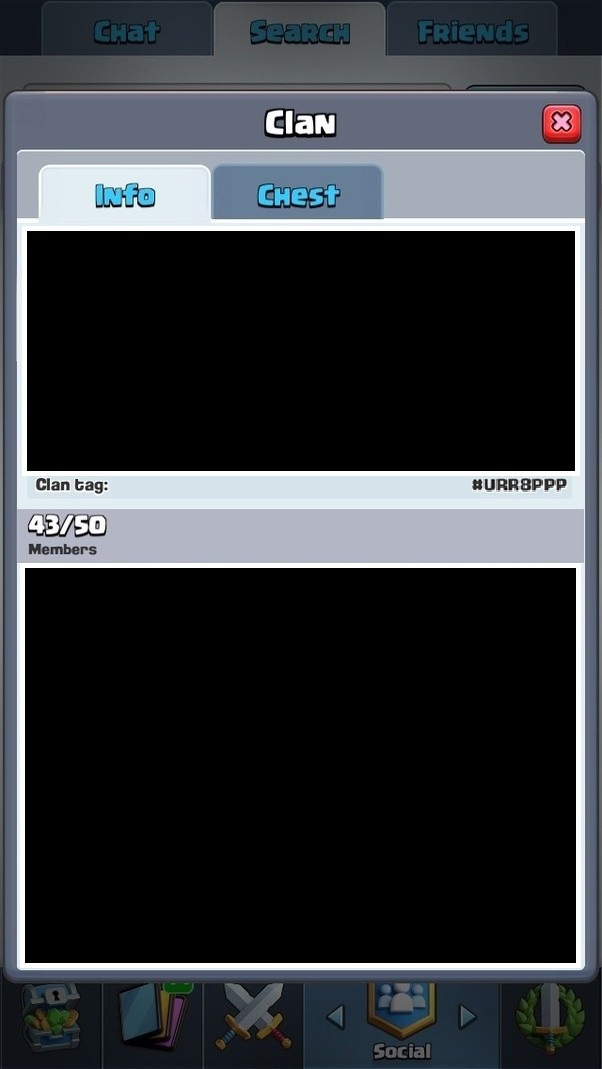

Get file size given file descriptor in Go
If given a path, I would use this to get file size
file, _ := os.Open(path)
fi, _ := file.Stat()
fsuze := fi.Size()
But if only given fd
, how can I get the file size?
Is there any way in golang like
fd
lseek(fd, 0, SEEK_END)
in C
Can I get File stat without opening the file?
Just like C, given path
int stat(const char*path, struct stat *statbuf)
I just looked into the golang os package and found
func Stat(name string) (FileInfo, error)
just like C's
int stat(const char*path, struct stat *statbuf)
fd
open()
Yes. I get this fd by calling os.File.stat().Fd()
– Cubey Mew
27 mins ago
2 Answers
2
You create a new *os.File
from a file descriptor using the os.NewFile
function.
*os.File
os.NewFile
You can do it exactly the same way as in C, using Seek
Seek
offset, err := f.Seek(0, os.SEEK_END)
But since you have the *os.File
already, you can call Stat
even if it was derived directly from the file descriptor.
*os.File
Stat
What will happen if I call os.NewFile, with the current file open. Does the new file a copy of the current file?
– Cubey Mew
20 mins ago
@CubeyMew: It's the same file descriptor, so nothing happens. Even if you opened it with a new fd, opening a file does not copy it.
– JimB
17 mins ago
I want to use Open first to get a *File. And later use Create(name string)(*File, error) to get the *os.File. Will this cause any effect on the file? (I dont want to pass *os.File as parameter or make it as struct member)
– Cubey Mew
12 mins ago
@CubeyMew: opening a file has doesn't effect the file. If the file doesn't exist, the obviously
os.Create
will have an effect.– JimB
6 mins ago
os.Create
ok. Thanks for your answer
– Cubey Mew
5 mins ago
try to get the file start
fileInfo, err := file.Stat()
if err != nil {...
}
files fileInfo.Size())
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Just to clarify, does
fd
mean an integer file descriptor, as one would get fromopen()
in C?– Jonathon Reinhart
41 mins ago