Animate TextView to increase integer and stop at some point?
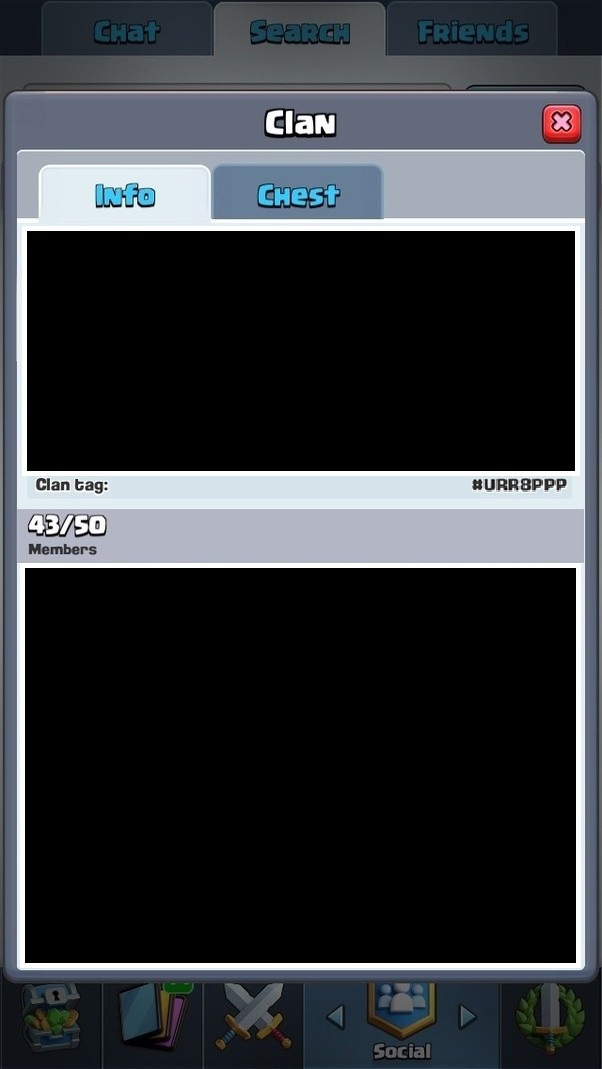

Animate TextView to increase integer and stop at some point?
I have a TextView showing integer value. Integer value is transferred from previous activity, and I want to add nice animation. I want to if for example int value is 73, I want textView to increase shown number by 1 until 73, so it would be 1-2-3-4-5...etc etc.
How can I do this?
you want animation why?? i mean you can simply use for loop and set the value in textview..and use thread or timer to upadte the text view on particular interval of time
– Meenal
Oct 22 '14 at 8:16
I don't see how could I achieve what I want with NumberPicker. My TextView is more like a score.
– user3094736
Oct 22 '14 at 8:17
@MeenalSharma Well it would look like its animated but it doesn't have to be. Your idea looks like a solution.
– user3094736
Oct 22 '14 at 8:22
try this github.com/uguratar/countingtextview
– Kishan Vaghela
Apr 13 '16 at 10:03
4 Answers
4
Here is a simple function to animate the text of a textView according to an initial and final value
public void animateTextView(int initialValue, int finalValue, final TextView textview) {
DecelerateInterpolator decelerateInterpolator = new DecelerateInterpolator(0.8f);
int start = Math.min(initialValue, finalValue);
int end = Math.max(initialValue, finalValue);
int difference = Math.abs(finalValue - initialValue);
Handler handler = new Handler();
for (int count = start; count <= end; count++) {
int time = Math.round(decelerateInterpolator.getInterpolation((((float) count) / difference)) * 100) * count;
final int finalCount = ((initialValue > finalValue) ? initialValue - count : count);
handler.postDelayed(new Runnable() {
@Override
public void run() {
textview.setText(String.valueOf(finalCount));
}
}, time);
}
}
this guy deserves a cookie
– ziggs
Nov 10 '15 at 16:22
Isn't it better to use Animator instead of handle it directly?
– HendraWD
Jan 29 at 2:46
The best solution in my opinion is to use this method :
public void animateTextView(int initialValue, int finalValue, final TextView textview) {
ValueAnimator valueAnimator = ValueAnimator.ofInt(initialValue, finalValue);
valueAnimator.setDuration(1500);
valueAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator valueAnimator) {
textview.setText(valueAnimator.getAnimatedValue().toString());
}
});
valueAnimator.start();
}
Best function! Work perfectly!
– Arief Rivai
Jul 20 '15 at 15:57
wonderful ! Just a remark, though : no need to cast
initialValue
and finalValue
to int since ValueAnimator.ofInt
expects integers ;-)– Mackovich
Dec 30 '15 at 14:41
initialValue
finalValue
ValueAnimator.ofInt
Perfect solution!
– Volodymyr Kulyk
Apr 27 '16 at 8:51
Oops! Works like a charm. the above solution by Pedro Oliveira do not work with max to min number animation.... This solution is awesome... the animation is smooth...better performance.
– Vishal Kumar
Jun 3 '17 at 23:24
This is awesome +1
– Harin Kaklotar
Jun 16 at 5:47
I think this project in github is what you want: https://github.com/sd6352051/RiseNumber
The RiseNumberTextView extends TextView and use the ValueAnimator to implement the rising number effect.
try this code..showing increment value with animation
public class MainActivity extends Activity implements AnimationListener {
private TextView textView;
AlphaAnimation fadeIn, fadeOut;
private static int count = 0, finalValue = 20;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.demo);
textView = (TextView) findViewById(R.id.textView);
fadeIn = new AlphaAnimation(0.0f, 1.0f);
fadeOut = new AlphaAnimation(1.0f, 0.0f);
fadeIn.setDuration(1000);
fadeIn.setFillAfter(true);
fadeOut.setDuration(1000);
fadeOut.setFillAfter(true);
fadeIn.setAnimationListener(this);
fadeOut.setAnimationListener(this);
textView.startAnimation(fadeIn);
textView.startAnimation(fadeOut);
}
@Override
public void onAnimationEnd(Animation arg0) {
// TODO Auto-generated method stub
Log.i("mini", "Count:" + count);
runOnUiThread(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
textView.setText("" + count);
}
});
if (count == finalValue) {
textView.setText("" + finalValue);
} else {
++count;
textView.startAnimation(fadeIn);
textView.startAnimation(fadeOut);
}
}
@Override
public void onAnimationRepeat(Animation arg0) {
// TODO Auto-generated method stub
}
@Override
public void onAnimationStart(Animation arg0) {
// TODO Auto-generated method stub
}
}
Works perfectly, thanks!
– user3094736
Oct 22 '14 at 9:12
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
are you aware of this: developer.android.com/reference/android/widget/…
– pskink
Oct 22 '14 at 8:09