My app crashes on google sign-in
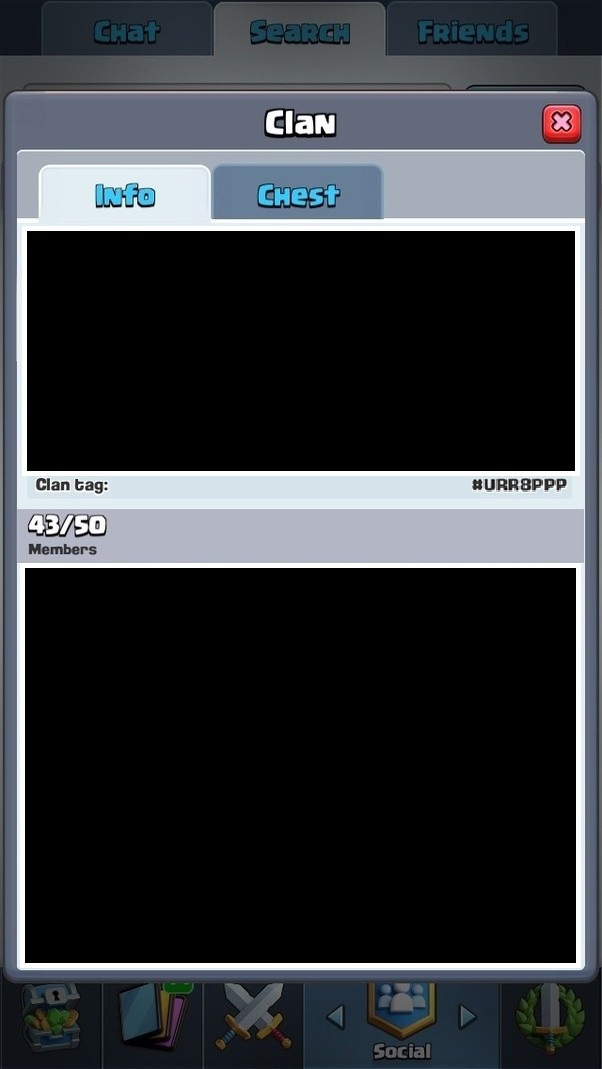

My app crashes on google sign-in
I am integrating google sign-in into my android project using Firebase. Following this tutorial, I was able to get the login. But when I choose the account, the app crashes.
By the way I am using Kotlin language.
The code snippet is as follows:
class SignupActivity:AppCompatActivity(){
lateinit var googleSignInClient: GoogleSignInClient
lateinit var gso: GoogleSignInOptions
val RC_SIGN_IN :Int =1
lateinit var signOut:Button
lateinit var mAuth: FirebaseAuth
private var callbackManager: CallbackManager?=null
@RequiresApi(Build.VERSION_CODES.JELLY_BEAN_MR1)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.signup)
header_signup_button.setOnClickListener {
startActivity(Intent(this,SplashscreenActivity::class.java))
}
gso = GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestIdToken(getString(R.string.default_web_client_id))
.requestEmail()
.build()
googleSignInClient = GoogleSignIn.getClient(this,gso)
mAuth = FirebaseAuth.getInstance()
signOut = findViewById<View>(R.id.signOutBtn) as Button
signOut.visibility= View.INVISIBLE
google_signup.setOnClickListener {
view:View?->signInGoogle()
}
signupemail_button.setOnClickListener {
startActivity(Intent(this,EmailSignupActivity::class.java))
}
val fblogin_button= findViewById<Button>(R.id.facebook_signup)
fblogin_button.setOnClickListener {
callbackManager = CallbackManager.Factory.create()
LoginManager.getInstance().logInWithReadPermissions(this, Arrays.asList("email"))
if (!isloggedIn()) {
LoginManager.getInstance().registerCallback(callbackManager,
object : FacebookCallback<LoginResult> {
override fun onSuccess(loginResult: LoginResult) {
Log.d("LoginActivity", "Facebook token" + loginResult.accessToken.token)
startActivity(Intent(baseContext, MainActivity::class.java))
}
override fun onCancel() {
Log.d("LoginActivity", "Facebook oncancel")
}
override fun onError(error: FacebookException?) {
Log.d("LoginActivity", "Facebook onError")
}
})
} else {
LoginManager.getInstance().unregisterCallback(callbackManager, object : FacebookCallback<LoginResult> {
override fun onSuccess(result: LoginResult?) {
GraphRequest(AccessToken.getCurrentAccessToken(), "/{user-id}/permissions/", null, HttpMethod.DELETE, GraphRequest.Callback() {
AccessToken.setCurrentAccessToken(null)
LoginManager.getInstance().logOut()
}).executeAsync()
Log.d("LoginActivity", "Facebook token" + result?.accessToken?.token)
}
override fun onCancel() {
Log.d("LoginActivityunregister", "Facebook oncancel")
}
override fun onError(error: FacebookException?) {
Log.d("LoginActivityunregister", "Facebook onError")
}
})
}
}
}
private fun signInGoogle() {
val signIntent:Intent =googleSignInClient.signInIntent
startActivityForResult(signIntent,RC_SIGN_IN)
}
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == RC_SIGN_IN) {
if (resultCode!= Activity.RESULT_OK) {
val task: Task<GoogleSignInAccount> = GoogleSignIn.getSignedInAccountFromIntent(data)
try {
val account = task.getResult()
val credential = GoogleAuthProvider.getCredential(account.idToken,null)
mAuth.signInWithCredential(credential)
.addOnCompleteListener(this, OnCompleteListener<AuthResult>(){
if (task.isSuccessful) {
val user = mAuth.currentUser
if (user != null) {
updateUI(user)
}
}else{
updateUI(null)
}
})
}catch (e:ApiException){
Toast.makeText(this,e.toString(),Toast.LENGTH_SHORT).show()
}
}
}
else {
callbackManager?.onActivityResult(requestCode, resultCode, data)
}
}
private fun updateUI(user: FirebaseUser?) {
val disptext = findViewById<View>(R.id.disptxt) as TextView
if (user != null) {
disptext.text = user.displayName
}
signOut.visibility=View.VISIBLE
signOut.setOnClickListener { view:View->
googleSignInClient.signOut().addOnCompleteListener {
task -> disptext.text = " "
signOut.visibility= View.INVISIBLE
}
}
}
@SuppressLint("NewApi")
override fun onBackPressed() {
super.onBackPressed()
startActivity(Intent(this,SplashscreenActivity::class.java))
}
}
private fun Any.unregisterCallback(callbackManager: CallbackManager?, facebookCallback: FacebookCallback<LoginResult>) {
}
fun isloggedIn(): Boolean {
val accessToken = AccessToken.getCurrentAccessToken()
return accessToken != null
}
The manifest file: In the manifest file I have added the metadata.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.vishwa.imaginators">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/myicon"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".SplashscreenActivity"
android:theme="@style/AppTheme"
android:noHistory="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".LoginActivity">
</activity>
<activity android:name=".SigninActivity">
</activity>
<activity android:name=".SignupActivity">
</activity>
<activity android:name=".EmailSignupActivity">
</activity>
<meta-data
android:name="com.google.android.gms.version"
android:value="@integer/google_play_services_version" />
<meta-data android:name="com.facebook.sdk.ApplicationId"
android:value="@string/facebook_app_id" />
<activity android:name="com.facebook.FacebookActivity"
android:configChanges=
"keyboard|keyboardHidden|screenLayout|screenSize|orientation"
android:label="@string/app_name"/>
<activity android:name=".MainActivity">
</activity>
</application>
</manifest>
The error that comes is Caused by: com.google.android.gms.tasks.RuntimeExecutionException: com.google.android.gms.common.api.ApiException: 10
. I have looked up this issue and no answer solves my problem.
Caused by: com.google.android.gms.tasks.RuntimeExecutionException: com.google.android.gms.common.api.ApiException: 10
Moreover, you'll also notice that I have Facebook sign-in integration as well for my project. But now that too is not working after adding the google sign-in. Therefore I am looking forward to some help. Any help is appreciated.
@AlexMamo I have edited the question including the manifest file.
– Eswar
21 mins ago
Error code 10 usually indicates a problem with your SHA1 fingerprint. See Sam Stern's comment at this post.
– Bob Snyder
13 mins ago
1 Answer
1
Your AndroidManifest.XML
looks correct to me. I encountered the same error:
AndroidManifest.XML
Caused by: com.google.android.gms.tasks.RuntimeExecutionException: com.google.android.gms.common.api.ApiException: 10
Which basically means that you are providing an unknown server client id.
In Google Developer Console, select your project you generate: OAuth client ID
-> Web Application and use this web application client id in your Android app.
OAuth client ID
Where should I add the client Id. In the manifest file?
– Eswar
2 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Please add your manifest file.
– Alex Mamo
25 mins ago