JFrame size is too small
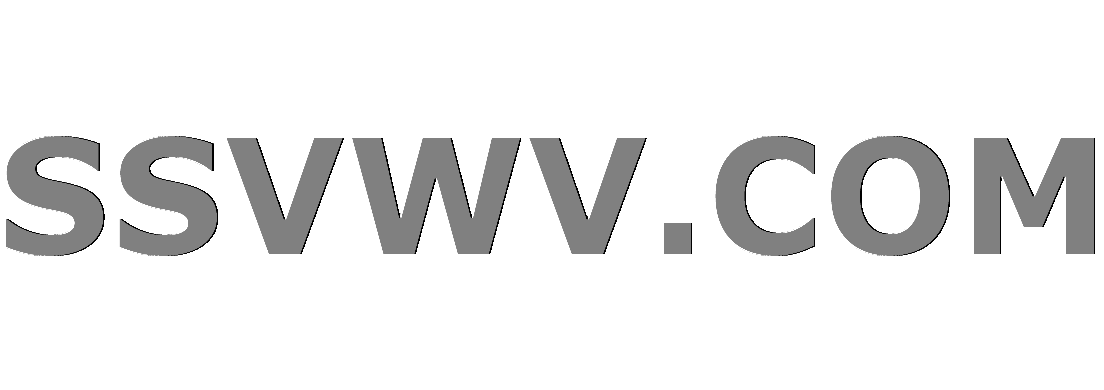
Multi tool use
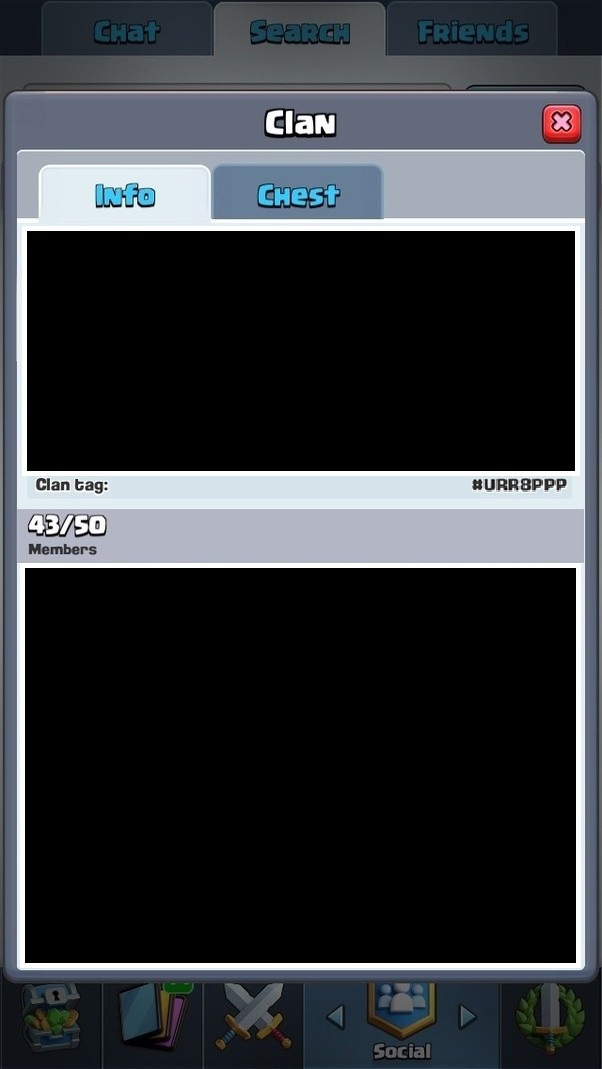

JFrame size is too small
i have created a JFrame in netbeans. But when i run the program, the Jframe size is too small.
here is my code.
import javax.swing.JFrame;
public class Window {
private static void demo()
{
JFrame frame =new JFrame();
frame.setVisible(true);
}
public static void main(String args) {
javax.swing.SwingUtilities.invokeLater(new Runnable(){
public void run()
{
demo();
}
});
}
}
"frame size is too small" Put components in it, then call
pack()
. The frame will become the smallest size it needs to be, in order to display the components inside it.– Andrew Thompson
Oct 27 '12 at 14:49
pack()
5 Answers
5
You can use frame.setSize(width, height)
in order to set its size or frame.setBounds(x, y, width, height)
for setting both the location and size.
frame.setSize(width, height)
frame.setBounds(x, y, width, height)
A better choice would be to call frame.pack()
after you add some components to its content pane.
frame.pack()
YES! it worked :). Thanks.
– Sam
Oct 27 '12 at 12:23
Try this way...
Use the setSize(width, height)
method of JFrame.
setSize(width, height)
public class Myframe extends JFrame{
public Myframe(){
this.setSize(300,300);
}
public static void main(String args){
EventQueue.invokeLater(new Runnable(){
public void run(){
Myframe f = new Myframe("Frame");
f.setVisible(true);
}
});
}
}
What is
f.visible()
?– nIcE cOw
Oct 27 '12 at 12:37
f.visible()
f is the instance of the class Myframe that has extends the JFrame
– Kumar Vivek Mitra
Oct 27 '12 at 12:53
No no what is visible ? Which method is this ?
– nIcE cOw
Oct 27 '12 at 12:57
@GagandeepBali sorry for that, i meant to say
setVisible(true);
. I have corrected it.... Sorry for the trouble....– Kumar Vivek Mitra
Oct 27 '12 at 18:24
setVisible(true);
If you want to maximize it you could try
this.setVisible(false);
this.setExtendedState(MAXIMIZED_BOTH);
this.setVisible(true);
this.setResizable(false);
Else for some specific size use
this.setSize(width,height);
You just need to add one line line so that you can give your frame a size.The line is
frame.setSize(300,300);
Here is the full code:
import javax.swing.JFrame;
public class Window {
private static void demo()
{
JFrame frame =new JFrame();
frame.setSize(300,300);
frame.setVisible(true);
}
public static void main(String args) {
javax.swing.SwingUtilities.invokeLater(new Runnable(){
public void run()
{
demo();
}
});
}
}
you must have a public components method like:
public gestion_name_of_class (){
initComponents();
}
Cordially.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Swing JComponents by default accepting only PreferredSize,
– mKorbel
Oct 27 '12 at 12:35