How to correctly map a set of data onto a table using React
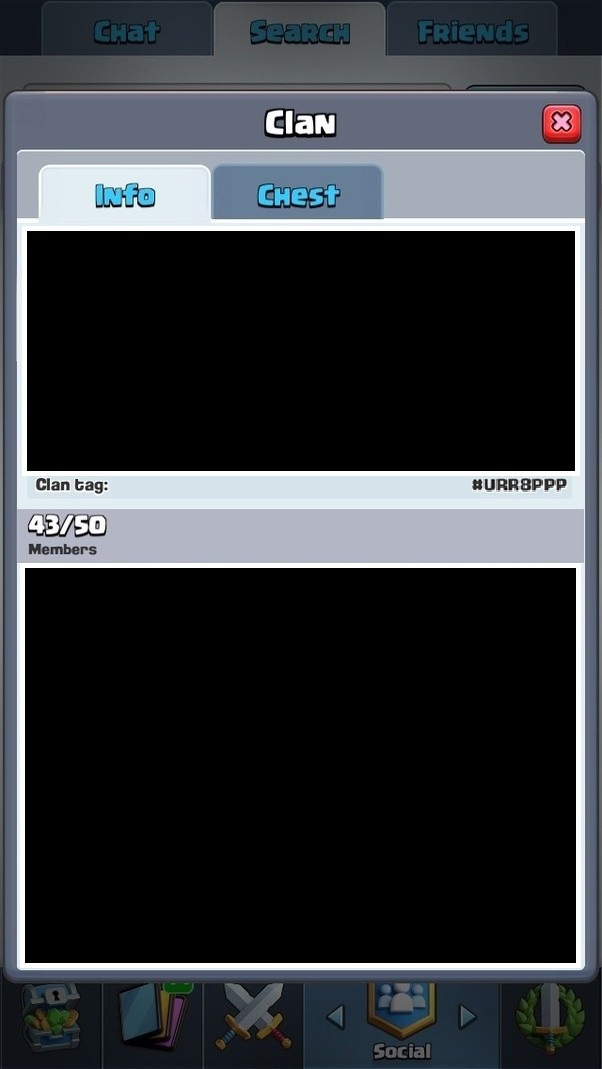

How to correctly map a set of data onto a table using React
I am trying to map data to the correct columns using react and am struggling to get everything to display in the correct column.
Here is my data structure which consists of an array of data - in this original array each object has a norm_data and and feature_data key - which again consists of an array of data with the same keys.
[
{
"norm_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"step_id": 98,
"cell_id": 38,
"feature_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"stim_id": 345
},
{
"norm_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"step_id": 97,
"cell_id": 37,
"feature_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"stim_id": 344
}
]
This is the closest that i have got so far where I have managed to get where I loop through the feature_data array to get the labels for the table.
Within that loop I need to somehow loop through the norm data_and the feature_data from into the next two td's - I have labelled where I need the data to go.
class Feature extends Component {
render() {
const { loading } = this.props;
if (loading) {
return <div>Loading...</div>;
}
const featureData = this.props.data[0].feature_data;
return (
<table>
<tbody><tr>
<td></td>
<td colSpan="2">Title</td>
</tr>
<tr>
<td></td>
<td><h2>actual</h2></td>
<td><h2>norm</h2></td>
</tr>
{featureData.map((data, key) => {
return (
<tr key={key}>
<td>
{data.feature}
</td>
<td>I need this to be the feature_data Value</td>
<td>I need this to be the norm_data Value</td>
</tr>
);
})}
</tbody></table>
);
}
}
Actual
Is the actual value corresponds to
feature_data
meanwhile "norm" is to norm_data
?– ionizer
12 mins ago
feature_data
norm_data
@ionizer yes sorry my mistake will edit
– marcus petty
5 mins ago
1 Answer
1
Based on my understanding to your problem I can only get you this far:
let data = [
{
"norm_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"step_id": 98,
"cell_id": 38,
"feature_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"stim_id": 345
},
{
"norm_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"step_id": 97,
"cell_id": 37,
"feature_data": [
{
"avg": 0,
"panelist": 0,
"feature": "Headline",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Small Print",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Call to Action",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Logo",
"exists": false
},
{
"avg": 0,
"panelist": 0,
"feature": "Subheadline",
"exists": false
}
],
"stim_id": 344
}
];
class App extends React.Component {
render() {
return (
<div>
<table>
<tbody>
<tr>
<td></td>
<td colSpan="2"><h1>Title</h1></td>
</tr>
<tr>
<td></td>
<td><h2>Actual</h2></td>
<td><h2>Norm</h2></td>
</tr>
{
data.map(item => {
return item.feature_data.map((v, i) => {
return (
<tr>
<td>{v.feature}</td>
<td>{v.avg}</td>
<td>{item.norm_data[i].avg}</td>
</tr>
);
})
})
}
</tbody>
</table>
</div>
);
}
}
ReactDOM.render(<App/>, document.getElementById('root'));
table, td {
border: 1px solid black;
}
table {
width: 100%;
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What is
Actual
value?– Olim Saidov
41 mins ago