Why is an if statement working but not a switch statement
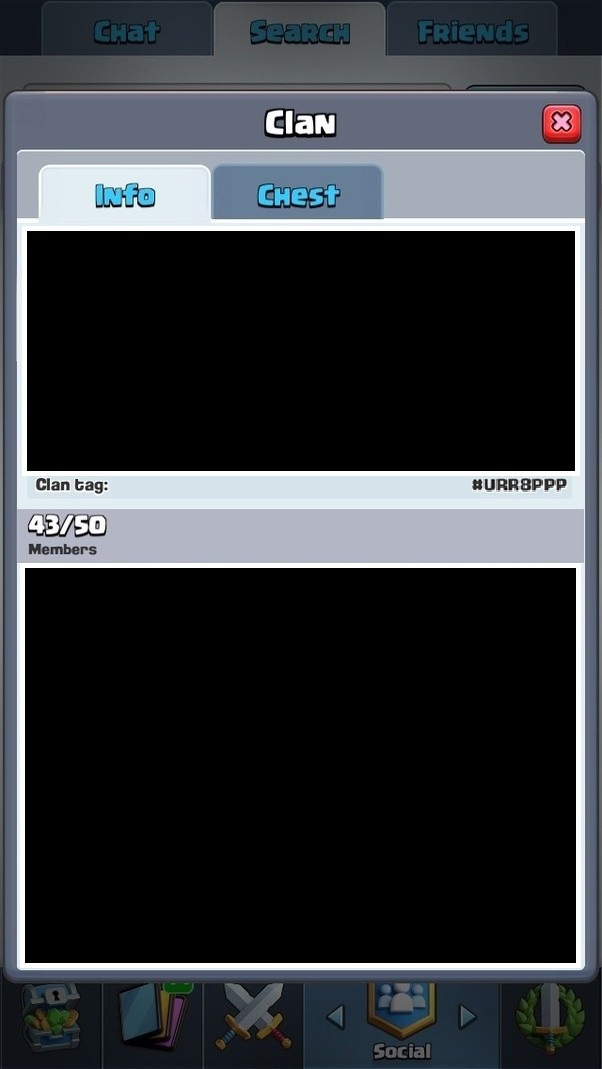

Why is an if statement working but not a switch statement
Title is terrible, but I wasn't too sure what to put.
I'm trying to create Switch Statement
using the char index of a string and an Enum using this wrapper to get the value of the selected enum from a Description, it pretty much allows you to store a string to an enum value.
Here is my if statement
Switch Statement
if statement
if (msgComingFromFoo[1] == Convert.ToChar(Message.Code.FOO_TRIGGER_SIGNAL.EnumDescriptionToString()))
{
//foo
}
and here is my Switch statement
Switch statement
switch (msgComingFromFoo[1])
{
case Convert.ToChar(Message.Code.FOO_TRIGGER_SIGNAL.EnumDescriptionToString()):
break;
}
Why is it accepting the if statement
and not the switch statement
?
I tried converting it to a char since I'm selected an index from a string, but unfortunately i didn't work.
if statement
switch statement
Update:
Here is the Message.Code
Enum
Message.Code
public class Message
{
public enum Code
{
[Description("A")]
FOO_TRIGGER_SIGNAL
}
}
As you can see, I need the Description assigned to the enun not the enum value that is 0, using Message.Code.FOO_TRIGGER_SIGNAL.EnumDescriptionToString()
from the mentioned wrapper returns A
not 0
Message.Code.FOO_TRIGGER_SIGNAL.EnumDescriptionToString()
A
0
3 Answers
3
You cannot have expressions in the case, but you can in the switch, so this will work:
switch (ConvertToMessageCode(msgComingFromFoo[1]))
{
case Message.Code.FOO_TRIGGER_SIGNAL:
break;
}
Where you will need to write ConvertToMessageCode to do the necessary conversion to the Message.Code enum. ConvertToMessageCode just abstracts the necessary conversion details, you may find you do not need a separate method, but can make do with inline code in the switch statement, e.g., a cast.
A case
in a switch
statement must refer to a constant value. You cannot evaluate an expression in a case
.
case
switch
case
CORRECT! : with that being said is there anything that can be done so there is not a massive if else statement? I have quite a few enum values and a switch would certainly make it a lot cleaner.
– AndrewE
32 mins ago
@AndrewE One possible solution is to redesign your code so that you can switch directly on the enum values directly instead of the description string.
– Code-Apprentice
30 mins ago
how about converting the msgComingFromFoo[1] to an enum value before doing the switch
– Kenneth Garza
30 mins ago
To add to @code-apprentice 's answer.
If you're finding the if
statement is becoming too long or has multiple conditions within if else
's. You can look at refactoring the code and encapsulating your logic into an object and using the visitor pattern to control the work to be done.
if
if else
Something like:
public interface IMessageLogic
{
void ProcessMessage()
}
public class TriggerSignal : IMessageLogic
{
public void ProcessMessage()
{
// Do trigger stuff
}
}
public class FooMessage : IMessageLogic
{
public void ProcessMessage()
{
// Do foo stuff
}
}
public class MessageHandler
{
public void HandleMessage(IMessageLogic messageLogic)
{
messageLogic.ProcessMessage();
}
}
public static void Main()
{
IMessageLogic messageLogic = GetMessage();
var handler = new MessageHandler();
handler.HandleMessage(messageLogic);
}
Visitor Pattern
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Please include the error you get with the switch statement as part of your question.
– Code-Apprentice
35 mins ago