Asking for user input twice in a while loop
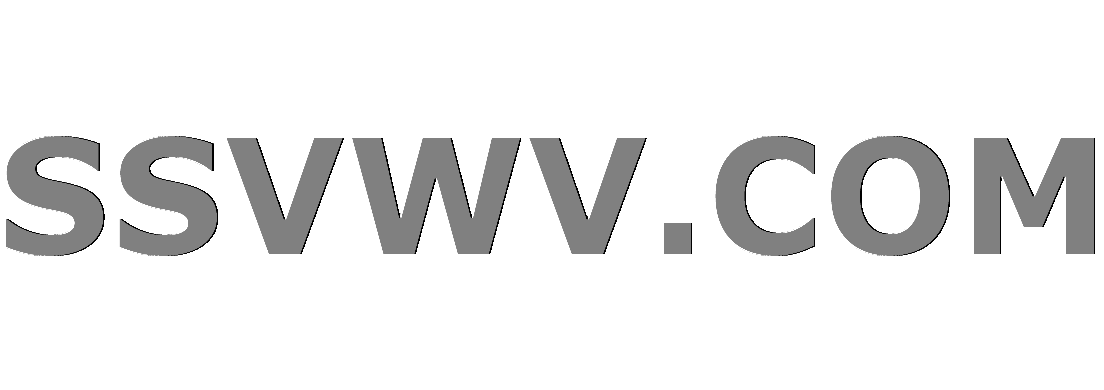
Multi tool use
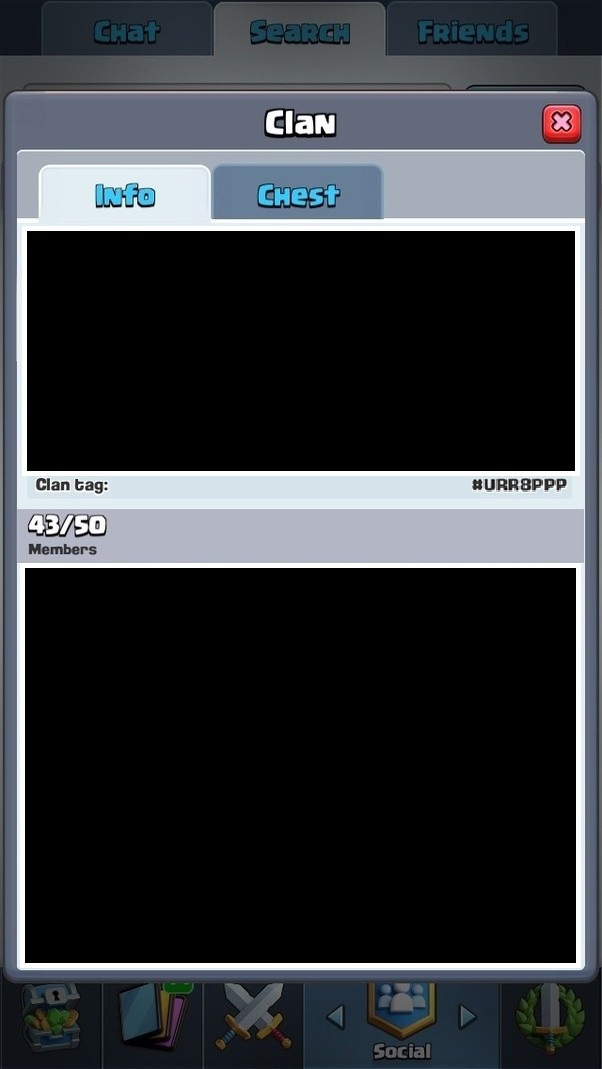

Asking for user input twice in a while loop
I'm currently doing a java project. The project involves a database about NHL Statistics and about accessing bits of information from the database through user input. I'm using a while loop to ask for user input in multiple circumstances, such as asking for the number of penalties received, the number of goals scored, amount of points etc. I am having difficulty with one specific part, it asks the user input for which statistic they want (points, goals, assists,penalties, assists, player, club). After that, if the user types player as their choice, then the system is supposed to ask the user which players statistics they wish to see. The problem is that since I'm using a while loop after the user inputs the first time, the loop is executed and the program ends before the player can input the second time. Any help would be appreciated, here is my code:
import java.util.Scanner;
import nhlstats.NHLStatistics;
public class NhlStatisticsPart2 {
public static void main(String args) {
Scanner reader = new Scanner(System.in);
System.out.println("NHL statistics service");
while (true) {
System.out.println("");
System.out.print("command (points, goals, assists, penalties, player, club, quit): ");
String command = reader.nextLine();
if (command.equals("quit")) {
break;
}
if (command.equals("points")) {
NHLStatistics.sortByPoints();
NHLStatistics.top(10);
// Print the top ten players sorted by points.
} else if (command.equals("goals")) {
NHLStatistics.sortByGoals();
NHLStatistics.top(10);
// Print the top ten players sorted by goals.
} else if (command.equals("assists")) {
NHLStatistics.sortByAssists();
NHLStatistics.top(10);
// Print the top ten players sorted by assists.
} else if (command.equals("penalties")) {
NHLStatistics.sortByPenalties();
NHLStatistics.top(10);
// Print the top ten players sorted by penalties.
} else if (command.equals("player")) {
System.out.println("Which player statistics?");
NHLStatistics.searchByPlayer(command);
// Ask the user first which player's statistics are needed and then print them.
} else if (command.equals("club")) {
// Ask the user first which club's statistics are needed and then print them.
// Note: When printing statistics they should be ordered by points (so the players with the most points come first).
}
}
}
}
1 Answer
1
Well you need to ask the user to input the player. So you need to use the scanner to scan another value. If you do it when you are in the if condition it won't continue until the user inputs the value.
.....
} else if (command.equals("player")) {
System.out.println("Which player statistics?");
command = reader.nextLine(); //Read again from the input
NHLStatistics.searchByPlayer(command); //now use that second value
}
.......
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.