How can i use ' ++ i ' inside this situation?
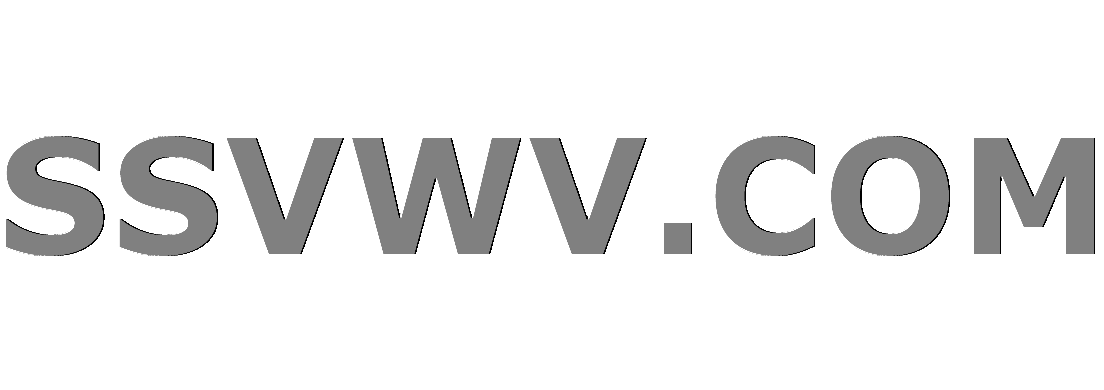
Multi tool use
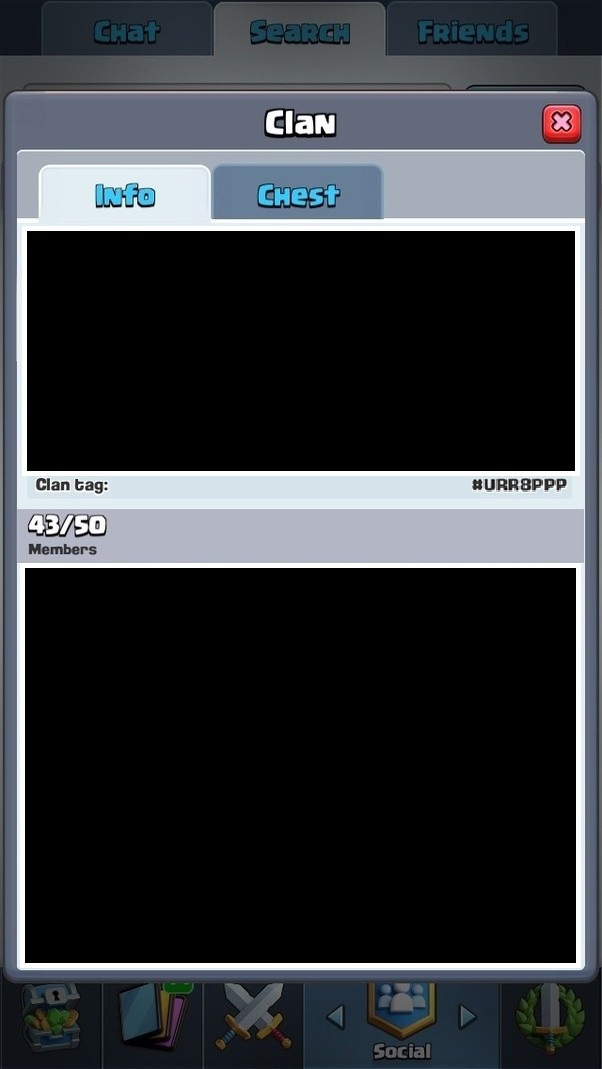

How can i use ' ++ i ' inside this situation?
I want to draw a random name for an event where users can win items specified by their ID (1,2,3 etc.). The random name part is ok by now but how can i display the result like:
The ID : 1 winner is: 'the random name'
The ID : 2 winner is: 'the random name'
etc...
till ID : 27
static void Main(string args)
{
string Names = { "Erik", "Levente", "Noel", "Áron", "Krisztián", "Kristóf", "Bence", "Roland", "Máté", "László", "Bálint" ,
"Regina", "Brigitta", "Gréta", "Hédi", "Hanna", "Boglárka", "Jázmin", "Réka", "Alexandra", "Rebeka", "Lili", "Luca", "Zsófi"};
List<string> alreadyUsed = new List<string>();
Random r = new Random();
while (alreadyUsed.Count < Names.Length)
{
int index = r.Next(0, Names.Length);
if (!alreadyUsed.Contains(Names[index]))
{
alreadyUsed.Add(Names[index]);
Console.WriteLine("The ID : 1 winner is: " + Names[index]);
}
}
Console.ReadKey(true);
}
++i
You don't have a variable called
i
, What exactly are you trying to achieve?– Sayse
24 mins ago
i
@Sayse he's trying to count at
The ID : 1 winner is
– fubo
23 mins ago
The ID : 1 winner is
Worth mentioning you have only 24 names, but you want to go till ID:27?
– Rafalon
20 mins ago
2 Answers
2
Here is a refactored appraoch without that bad performing alreadyUsed
. First I randomize the array, second I iterate and display each item with a incrementing index / id.
alreadyUsed
string Names = { "Erik", "Levente", "Noel", "Áron", "Krisztián", "Kristóf", "Bence", "Roland", "Máté", "László", "Bálint" , "Regina", "Brigitta", "Gréta", "Hédi", "Hanna", "Boglárka", "Jázmin", "Réka", "Alexandra", "Rebeka", "Lili", "Luca", "Zsófi"};
Random r = new Random();
Names = Names.OrderBy(_ => r.Next()).ToArray();
for(int i=0;i< Names.Length;i++)
{
Console.WriteLine("The ID : " + (i+1) + " winner is: " + Names[i]);
}
while (alreadyUsed.Count < Names.Length)
{
int index = r.Next(0, Names.Length);
if (!alreadyUsed.Contains(Names[index]))
{
alreadyUsed.Add(Names[index]);
Console.WriteLine("The ID : " + alreadyUsed.Count + " winner is: " + Names[index]);
}
}
You do not need ++i
, you already have the position contained in alreadyUsed.Count
that will increment itself as you use alreadyUsed.Add(...)
.
++i
alreadyUsed.Count
alreadyUsed.Add(...)
Try it online
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Why do you specifically want to use
++i
?– mjwills
29 mins ago