TypeError: Cannot read property 'map' of undefined ReactJS API calls
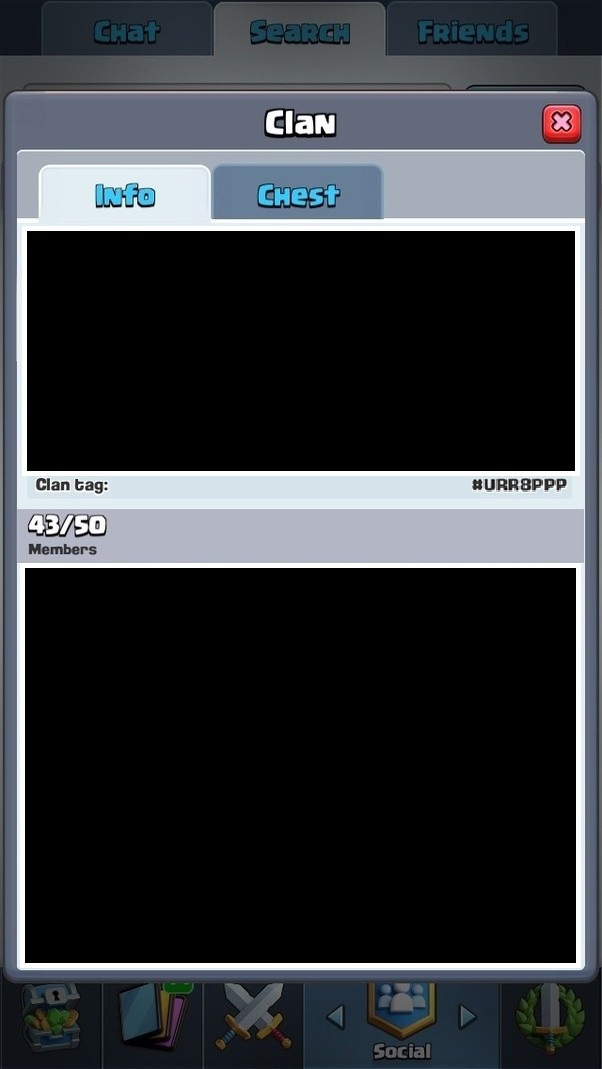

TypeError: Cannot read property 'map' of undefined ReactJS API calls
I have recently been trying to play with APIs in React.js,
I believed the below code would have worked, based on a tutorial from https://reactjs.org/docs/faq-ajax.html, However when I run this code I keep getting
TypeError: Cannot read property 'map' of undefined
Below is my code, this is a component called DOTA and is exported to my App.js
import React from 'react';
const API_KEY ="some-api-key";
const DEFAULT_QUERY = 'redux';
class DOTA extends React.Component {
constructor(props) {
super(props);
this.state = {
error: null,
isLoaded: false,
info: ,
};
}
componentDidMount() {
fetch(API_KEY + DEFAULT_QUERY)
.then(response => response.json(console.log(response)))
.then((result) => {
console.log(result)
this.setState({
isLoaded: true,
info: result.info
});
},
// Note: it's important to handle errors here
// instead of a catch() block so that we don't swallow
// exceptions from actual bugs in components.
(error) => {
this.setState({
isLoaded: true,
error
});
}
)
}
render() {
const { error, isLoaded, info } = this.state;
if (error) {
return <div>Error: {error.message}</div>;
} else if (!isLoaded) {
return <div>Loading...</div>;
} else {
return (
<ul>
{this.props.info.map(item => (
<li key={ item.all_word_counts}>
</li>
))}
</ul>
);
}
}
}
export default DOTA
App.js
import React, { Component } from 'react';
import DOTA from './components/DOTA/DOTA'
import './App.css';
class App extends Component {
render() {
return (
<div className="App">
<DOTA />
</div>
);
}
}
export default App;
I have looked on here already for an answer but I can't seem to find someone with the same issue. Do I need to run .map() outside of JSX? Above the render? Or am I just missing something?
Thanks!
OP destructing
info
from state, so it should be just info
.– devserkan
2 mins ago
info
info
1 Answer
1
DOTA
component does not get any props
but you are trying to map
over this.props.info
. Actually you are destructing info
from your state top of your render.
DOTA
props
map
this.props.info
info
Here how this works. info
is in the state and you are destructing it as:
info
const { error, isLoaded, info } = this.state;
This grabs error
, isLoaded
and info
from state and assign it variables. So, you can use info
instead of this.state.info
. Actually you are using error
like this top of if
block.
error
isLoaded
info
info
this.state.info
error
if
So, use it in the related part like:
<ul>
{info.map(item => (
<li key={ item.all_word_counts}>
</li>
))}
</ul>
But, with your current code, there is not any value inside your li
s. I hope your are just testing right now.
li
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
It should be "this.state.info"
– Rohan Veer
15 mins ago