Can't make square on canvas move, and now the square won't draw either
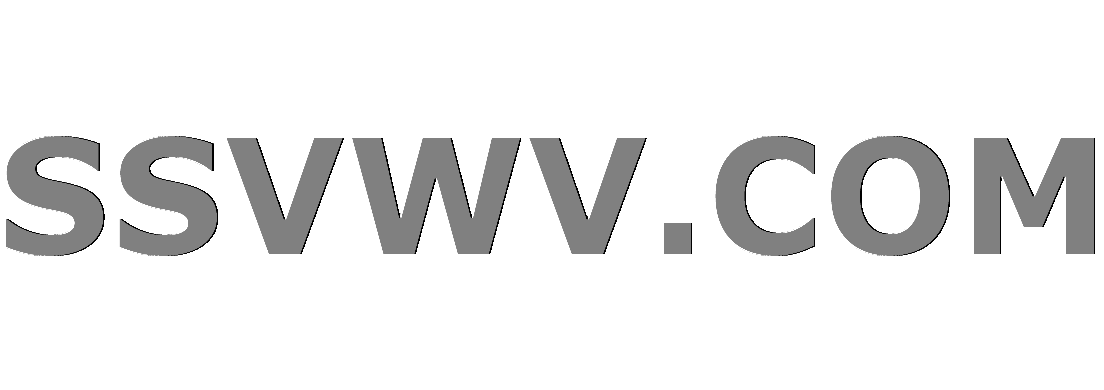
Multi tool use
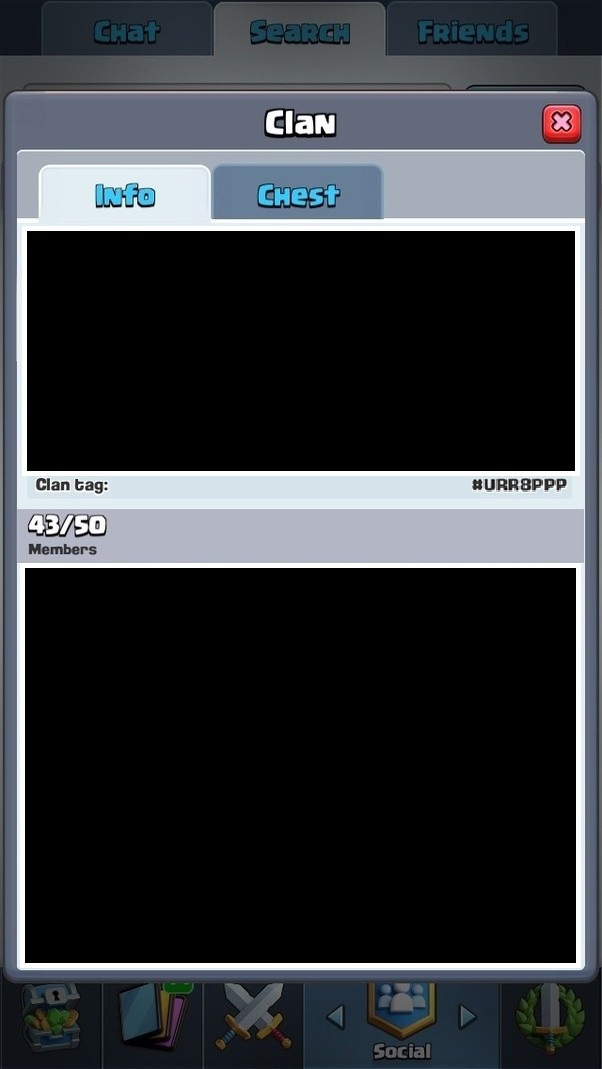

Can't make square on canvas move, and now the square won't draw either
I am trying to make an already drawn square move with the WASD keys.
I wasn't sure how to do this, so I looked up some code, and after about 2 hours came up with my own non-working code. It wasn't working, but at least it was drawing my square... Or was.
Now it isn't, and I have no clue why, here's my JavaScript:
function initCanvas(){
var ctx = document.getElementById('my_canvas').getContext('2d');
ctx.addEventListener("keydown", move, true);
function move(event){
//W
if(event.keyCode == 87){
y = y + 20;
}
//A
else if(event.keyCode == 65){
x = x - 20;
}
//S
else if(event.keyCode == 83){
y = y + 20;
}
//D
else if(event.keyCode == 68){
x = x + 20;
}
}
var x = 0;
var y = 0;
ctx.fillStyle = "green";
ctx.fillRect(x + 20, y + 20, 20, 20);
}
window.addEventListener('load', function(event){
initCanvas();
});
And HTML/CSS (entire page): http://pastebin.com/wjXv5tdK
It probably has to do with the Event Listener because it seems to work without it.
TL;DR
So I basically want a square to be drawn on the canvas, and have the user control it using the WASD keys.
They probably downvoted because you didn't put your code here to look at.. this website won't mess up your code, everybody else manages
– Daniel Stanley
Jan 28 '15 at 17:16
Maybe it was downvoted because you haven't been very clear - "I am trying to make something move" what something? "drawing my square" - so you want a square drawn? Honestly, it could be a little clearer. I don't think it warrants downvoting though, the downvoter should have commented and suggested improvements.
– theonlygusti
Jan 28 '15 at 17:17
When I enter my code here it doesn't show up in a box like it should. Instead, it just bunches it up into like 6-8 lines. I ended up messing around with how it works and got only half of it to work, while the other half wouldn't show up at all.
– Maddoga
Jan 28 '15 at 17:18
Thanks for the suggestions. I modified it, and took some suggestions that were submitted into the question.
– Maddoga
Jan 28 '15 at 17:20
2 Answers
2
Listen for keyboard events on the document, not the context.
document.addEventListener("keydown",move,false);
Here's some annotated code to get your started again:
// create canvas related variables
var canvas=document.getElementById("canvas");
var ctx=canvas.getContext("2d");
var cw=canvas.width;
var ch=canvas.height;
// set canvas to be a tab stop (necessary to give it focus)
canvas.setAttribute('tabindex','0');
// set focus to the canvas
canvas.focus();
// create an x & y indicating where to draw the rect
var x=150;
var y=150;
// draw the rect for the first time
draw();
// listen for keydown events on the document
// the canvas does not trigger key events
document.addEventListener("keydown",handleKeydown,false);
// handle key events
function handleKeydown(e){
// if the canvas isn't focused,
// let some other element handle this key event
if(e.target.id!=='canvas'){return;}
// change x,y based on which key was down
switch(e.keyCode){
case 87: x+=20; break; // W
case 65: x-=20; break; // A
case 83: y+=20; break; // S
case 68: y-=20; break; // D
}
// redraw the canvas
draw();
}
// clear the canvas and redraw the rect in its new x,y position
function draw(){
ctx.clearRect(0,0,cw,ch);
ctx.fillRect(x,y,20,20);
}
body{ background-color: ivory; padding:10px; }
#canvas{border:1px solid red;}
<h4>Click in the canvas to have it respond to keys</h4>
<canvas id="canvas" width=300 height=300></canvas>
It works when I remove the focus things. I also had to change the keycodes because the way you had them made up go right, and etc, but that's not a problem at all. Thanks a ton for all the help!
– Maddoga
Jan 29 '15 at 13:00
The reason the square doesn't draw anymore is you are trying to attach an event listener to a canvas context, and you can only attach listeners to the DOM object (the canvas). So if you change the statements to (for example):
var canvas = document.getElementById('my_canvas');
canvas.addEventListener("keydown", move, true);
And leave the ctx statements as they are the canvas will draw again. Unless you really need a canvas you might be better off using an svg img.
CTX was just what I named my var canvas. I tried changing those lines to Canvas, but no luck. I went back and changed everything to canvas and still had no luck. I ended up changing it back to CTX. Not sure what I'm doing wrong, but it has something to do with the event listener because it works without that.
– Maddoga
Jan 28 '15 at 19:13
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Who downvoted it, and why?
– Maddoga
Jan 28 '15 at 17:02