How to create a image of a chart with Java
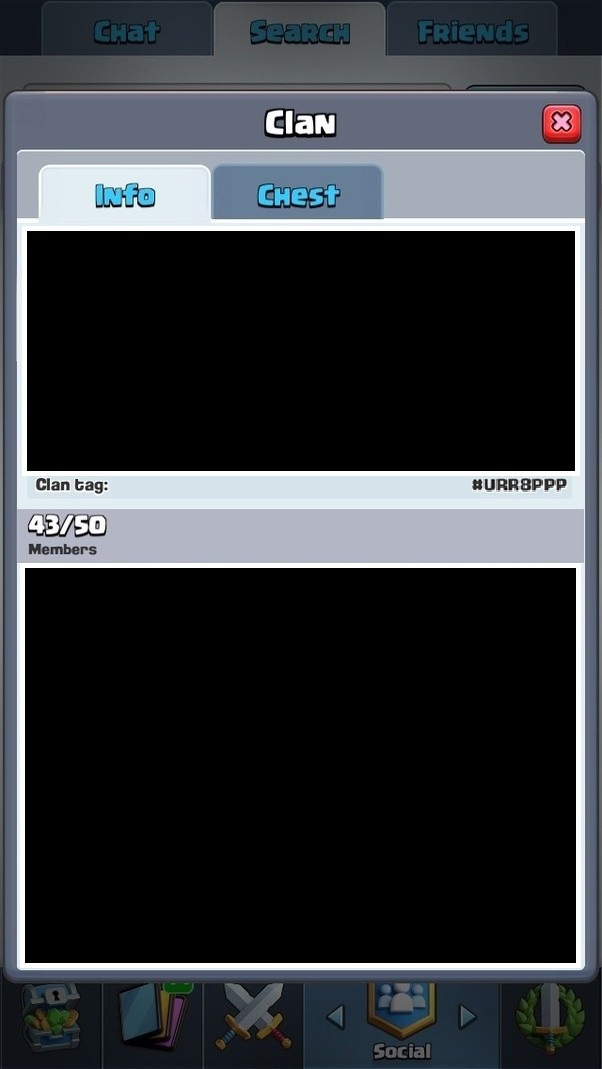

How to create a image of a chart with Java
I need to create a chart by reading some entries in my DB. I just want to create a image of the chart and create a new png. I prefer to do this with Java native libraries, so far I was able to get the following working.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.embed.swing.SwingFXUtils;
import javafx.scene.Scene;
import javafx.scene.image.WritableImage;
import javafx.stage.Stage;
import javafx.scene.chart.*;
import javafx.scene.Group;
import java.io.File;
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
import javax.imageio.ImageIO;
public class Test extends Application {
@Override public void start(Stage stage) {
Scene scene = new Scene(new Group());
stage.setTitle("Imported Fruits");
stage.setWidth(500);
stage.setHeight(500);
ObservableList<PieChart.Data> pieChartData =
FXCollections.observableArrayList(
new PieChart.Data("Grapefruit", 13),
new PieChart.Data("Oranges", 25),
new PieChart.Data("Plums", 10),
new PieChart.Data("Pears", 22),
new PieChart.Data("Apples", 30));
final PieChart chart = new PieChart(pieChartData);
chart.setTitle("Imported Fruits");
((Group) scene.getRoot()).getChildren().add(chart);
stage.setScene(scene);
WritableImage img = new WritableImage(800, 800);
scene.snapshot(img);
writeImage(Paths.get("/Users/images"), "test.png", img);
}
public static void writeImage(Path path, String fileName, WritableImage image) {
File file = new File(Paths.get(path.toString(), fileName).toString());
try {
ImageIO.write(SwingFXUtils.fromFXImage(image, null), "png", file);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String args) {
launch(args);
}
}
In the above sample I need to launch an application and get the chart created. I simply need to create a image of the chart and write it to a file, is there a way to get this done without launching the App? Or is there a better way to do this?
1 Answer
1
Following is How you can get this done with JavaFX,
import javafx.application.Platform;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.embed.swing.JFXPanel;
import javafx.embed.swing.SwingFXUtils;
import javafx.scene.Scene;
import javafx.scene.chart.PieChart;
import javafx.scene.image.WritableImage;
import javafx.stage.Stage;
import java.io.File;
import java.io.IOException;
import java.nio.file.Paths;
import javax.imageio.ImageIO;
public class Test {
public static void main(String args) {
String chartGenLocation = "/Users/work/tmp";
new JFXPanel();
ObservableList<PieChart.Data> pieChartData =
FXCollections.observableArrayList(
new PieChart.Data("Failed", 10),
new PieChart.Data("Skipped", 20));
final PieChart chart = new PieChart(pieChartData);
chart.setAnimated(false);
Platform.runLater(() -> {
Stage stage = new Stage();
Scene scene = new Scene(chart, 500, 500);
stage.setScene(scene);
WritableImage img = new WritableImage(500, 500);
scene.snapshot(img);
File file = new File(Paths.get(chartGenLocation, "a.png").toString());
try {
ImageIO.write(SwingFXUtils.fromFXImage(img, null), "png", file);
} catch (IOException e) {
//logger.error("Error occurred while writing the chart image
}
});
}
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
See stackoverflow.com/q/23590974/1247781 which this question may be a duplicate of, at its root.
– Vulcan
Jul 14 at 18:39