How to find the type of the caller of a function in Rust?
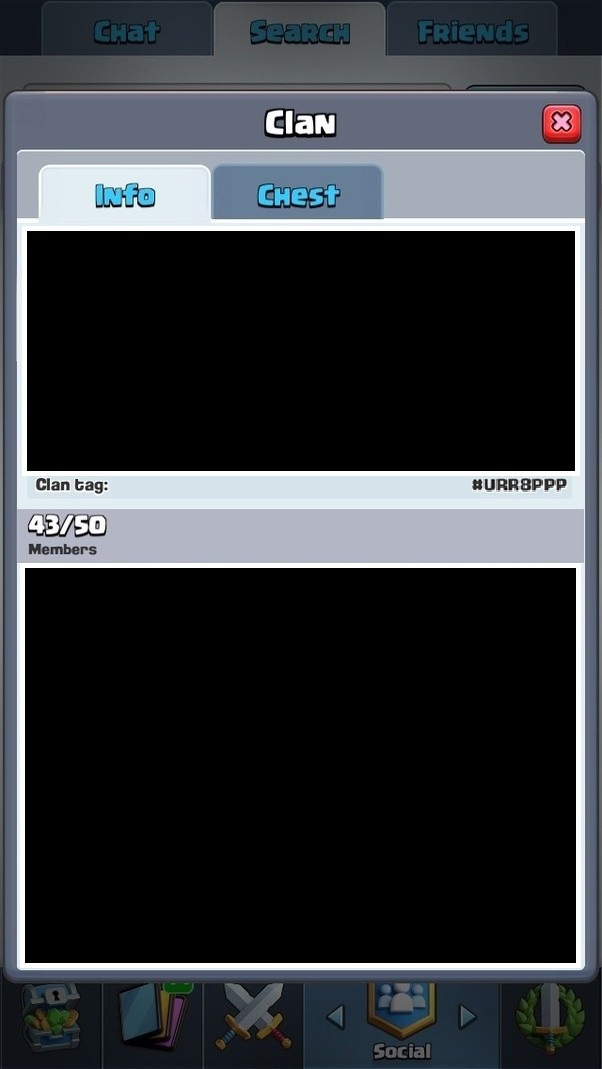

How to find the type of the caller of a function in Rust?
How can I get the caller type in my function?
struct A;
struct B;
impl A {
fn new() -> Self {
A
}
fn call_function(&self) {
B::my_function();
}
}
impl B {
pub fn my_function() {
println!("Hello");
// println!("{}" type_of_the_caller) // I want to get type A here
// Is it possible to get the caller type which is A in this case?
}
}
fn main() {
let a = A::new();
a.call_function();
}
Here is the working code in playground. This is simplified code for an example.
fn call_function(&self)
@Boiethios clarified the question
– Akiner Alkan
4 hours ago
You didn't understand my question: why do you want the type of the caller? Rust is not a dynamic language nor has reflection so it isn't possible as is. I suspect that you're doing some kind of cargo cult.
– Boiethios
4 hours ago
I see, I was trying to implement publish subscribe design and did not wanted to explicitly specify the subscriber type, since the subscriber calls the subscribe method itself. So I guess, I need to pass it's reference or at least it's type explicity for subscription?
– Akiner Alkan
4 hours ago
Ok. In this case, you need to use a trait
Suscriber
(for example) with the desired methods in it, and each suscriber must implement this trait.– Boiethios
4 hours ago
Suscriber
1 Answer
1
Rust doesn't have any machinery like this built-in. If you want to know some context inside a function then you'll need to pass it in as an argument.
Also, Rust doesn't have a way to get the name of a type, so you'd have to provide that too. For example, using a trait:
trait Named {
fn name() -> &'static str;
}
impl Named for A {
fn name() -> &'static str {
"A"
}
}
Which you might use like this:
impl B {
pub fn my_function<T: Named>(_: &T) {
println!("Hello");
println!("{}", T::name());
}
}
You have to pass in the caller when you call it:
impl A {
fn call_function(&self) {
B::my_function(self);
}
}
Outputs:
Hello
A
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I guess you meant
fn call_function(&self)
? Please add more precision about what you want to do with the type of the caller– Boiethios
5 hours ago