Add null-like value to String in Java
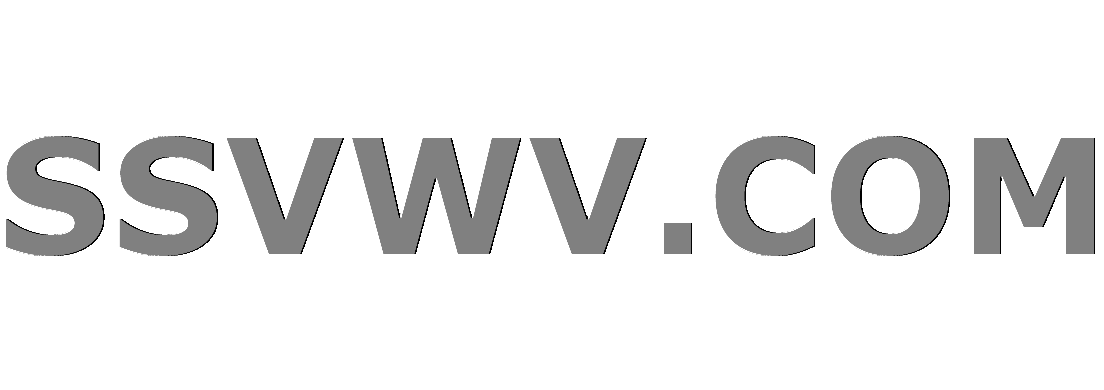
Multi tool use
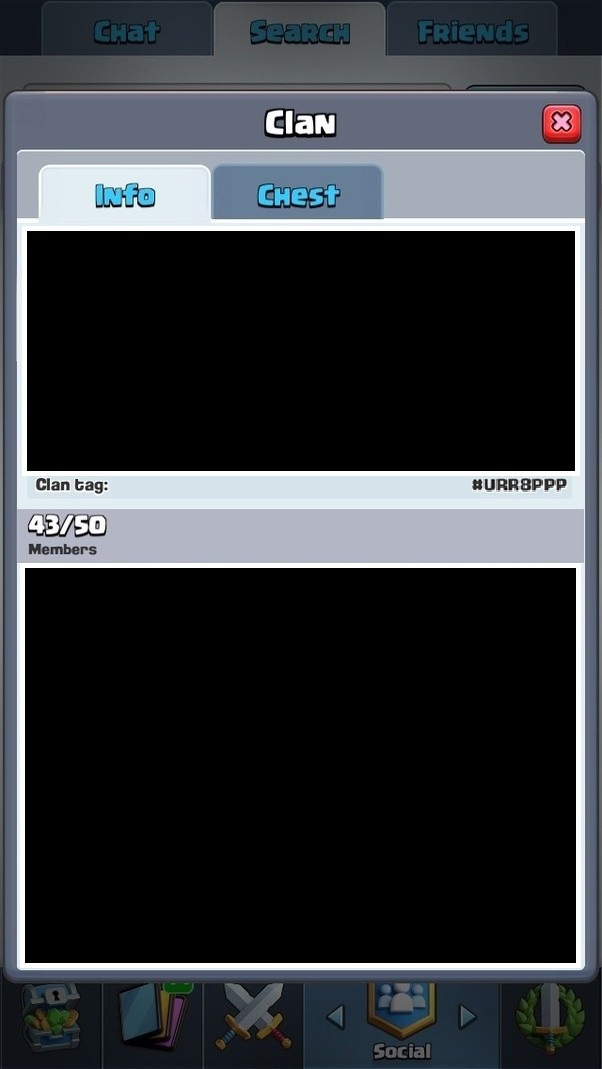

Add null-like value to String in Java
I am writing test method like setTask(Task task)
. And Task
object has several fields, e.g.
setTask(Task task)
Task
public String vehicle;
Method setTask
should be used in different test-cases, so I'd like to have an options for this field to accept values:
setTask
null
some string value
"", "Hello, World!", "Iso Isetta", ...
random
So what can I do to make String
to be SpecialString
which could accept values null
, random
& some string value
? (BTW: I don't want to set it to string value "RANDOM", and chech whether the value is equal to "RANDOM"-string)
String
SpecialString
null
random
some string value
UPDATE: I don't mean random
like random value from a set of values
, I mean random
as well as null
and this is for setTask()
to handle random
(select random from drop-down), and not to pass a random string from a set of values.
random
random value from a set of values
random
null
setTask()
random
1 Answer
1
Don't assign a fixed String
but use a Supplier<String>
which can generate a String
dynamically:
String
Supplier<String>
String
public Supplier<String> vehicleSupplier;
This, you can assign a generator function as you request:
static Supplier<String> nullSupplier () { return () -> null; }
static Supplier<String> fixedValueSupplier (String value) { return () -> value; }
static Supplier<String> randomSupplier (String... values) {
int index = ThreadLocalRandom.current().nextInt(values.length) -1;
return index > 0 && index < values.length ? values[index] : null;
}
In use, this looks like:
task.setVehicleSupplier(nullSupplier()); // or
task.setVehicleSupplier(fixedValueSupplier("value")); // or
task.setVehicleSupplier(randomSupplier("", "Hello, World!", "Iso Isetta"));
and you can get the String by
String value = task.vehicleSupplier().get();
or hide the implementation in a getter function
class Task {
// ...
private Supplier<String> vehicleSupplier;
public void setVehicleSupplier(Supplier<String> s) {
vehicleSupplier = s;
}
public String getVehicle() {
return vehicleSupplier != null ? vehicleSupplier.get() : null;
}
// ...
}
Thanks for reply! To clear up things: I don't mean
random
like random value from a set of values
, I mean random
as well as null
and this is for setTask()
to handle random
(select random from drop-down), and not just to pass random string from a set of values.– Ivan Gerasimenko
5 mins ago
random
random value from a set of values
random
null
setTask()
random
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
It sounds like I misunderstood your question. Could you post a pseudocode sampe of how you think it should look like?
– daniu
2 mins ago