Image Encryption in Swift
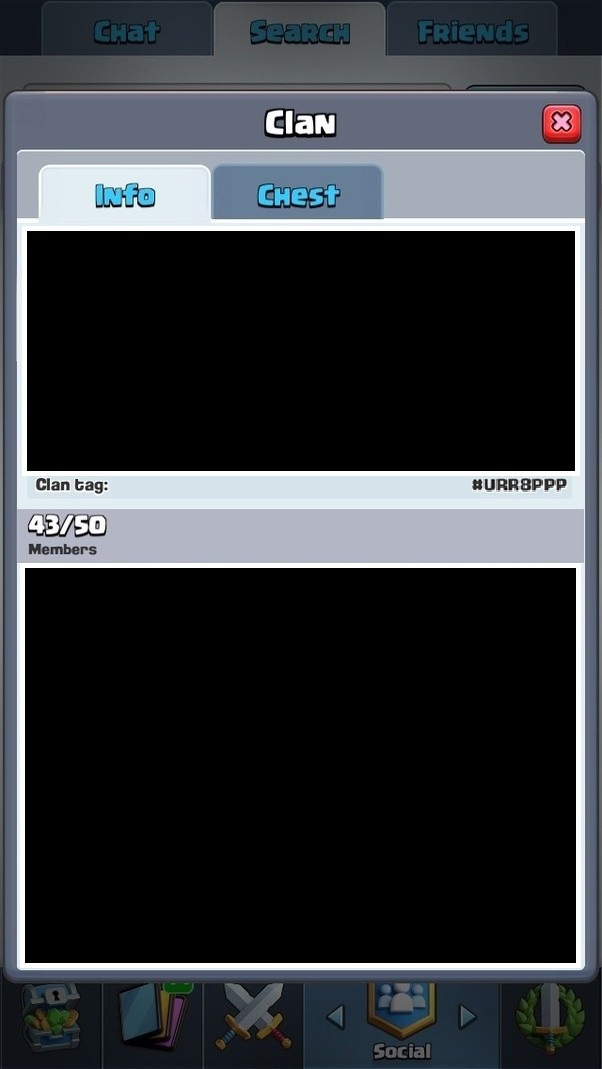

Image Encryption in Swift
I am using IDZSwiftCommonCrypto
for image encryption using StreamCryptor described as an example at its GitHub page: https://github.com/iosdevzone/IDZSwiftCommonCrypto
IDZSwiftCommonCrypto
I am not able to successfully decrypt. Here is my code for encryption and decryption (imageData comes from UIImageView
). The output is different from input after encryption (imageData is different from xx).
UIImageView
Encryption:
func performImageEncryption(imageData: Data) -> Void {
var inputStream = InputStream(data: imageData)
let key = arrayFrom(hexString: "2b7e151628aed2a6abf7158809cf4f3c")
var sc = StreamCryptor(operation:.encrypt, algorithm:.aes, options:.PKCS7Padding, key:key, iv:Array<UInt8>())
var inputBuffer = Array<UInt8>(repeating:0, count:1024)
var outputBuffer = Array<UInt8>(repeating:0, count:1024)
inputStream.open()
var cryptedBytes = 0
var xx = Data()
var count = 0
while inputStream.hasBytesAvailable
{
count = count + 1024
let bytesRead = inputStream.read(&inputBuffer, maxLength: inputBuffer.count)
let status = sc.update(bufferIn: inputBuffer, byteCountIn: bytesRead, bufferOut: &outputBuffer, byteCapacityOut: outputBuffer.count, byteCountOut: &cryptedBytes)
xx.append(contentsOf: outputBuffer)
}
let status = sc.final(bufferOut: &outputBuffer, byteCapacityOut: outputBuffer.count, byteCountOut: &cryptedBytes)
xx.append(contentsOf: outputBuffer)
inputStream.close()
performImageDecryption(encryptedImageData: xx)
}
Decryption:
func performImageDecryption(encryptedImageData: Data) -> Void {
let key = arrayFrom(hexString: "2b7e151628aed2a6abf7158809cf4f3c")
var sc = StreamCryptor(operation:.decrypt, algorithm:.aes, options:.PKCS7Padding, key:key, iv:Array<UInt8>())
var inputStreamD = InputStream(data: encryptedImageData)
var inputBuffer = Array<UInt8>(repeating:0, count:1024)
var outputBuffer = Array<UInt8>(repeating:0, count:1024)
inputStreamD.open()
var cryptedBytes = 0
var xx = Data()
while inputStreamD.hasBytesAvailable
{
let bytesRead = inputStreamD.read(&inputBuffer, maxLength: inputBuffer.count)
let status = sc.update(bufferIn: inputBuffer, byteCountIn: bytesRead, bufferOut: &outputBuffer, byteCapacityOut: outputBuffer.count, byteCountOut: &cryptedBytes)
xx.append(contentsOf: outputBuffer)
}
let status = sc.final(bufferOut: &outputBuffer, byteCapacityOut: outputBuffer.count, byteCountOut: &cryptedBytes)
xx.append(contentsOf: outputBuffer)
inputStreamD.close()
}
@IOSSingh Except for the fact that base64 isn't an encryption algorithm and provides no security whatsoever.
– Luke Joshua Park
Jul 20 '17 at 14:18
1 Answer
1
xx.append(outputBuffer, count: cryptedBytes)
should help.
below is sample code for picking up an encrypted image file and returning the data.
func decryptImage(from path:URL)-> Data? {
var decryptData = Data()
let sc = StreamCryptor(operation:.decrypt, algorithm:.aes, options:.PKCS7Padding, key:key, iv:iv)
guard let encryptedInputStream = InputStream(fileAtPath: path.relativePath) else {
return nil
}
var inputBuffer = [UInt8](repeating: 0, count: Int(1024))
var outputBuffer = [UInt8](repeating: 0, count: Int(1024))
encryptedInputStream.open()
var cryptedBytes : Int = 0
while encryptedInputStream.hasBytesAvailable
{
let bytesRead = encryptedInputStream.read(&inputBuffer, maxLength: inputBuffer.count)
let status = sc.update(bufferIn: inputBuffer, byteCountIn: bytesRead, bufferOut: &outputBuffer, byteCapacityOut: outputBuffer.count, byteCountOut: &cryptedBytes)
if (status != Status.success) {
encryptedInputStream.close()
return nil
}
if(cryptedBytes > 0)
{
decryptData.append(outputBuffer, count: cryptedBytes)
}
}
let status = sc.final(bufferOut: &outputBuffer, byteCapacityOut: outputBuffer.count, byteCountOut: &cryptedBytes)
if (status != Status.success) {
encryptedInputStream.close()
return nil
}
if(cryptedBytes > 0)
{
decryptData.append(outputBuffer, count: cryptedBytes)
}
encryptedInputStream.close()
return decryptData
}
Happy coding :)
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
you can use base_64 for encryption and descryption.
– IOS Singh
Jul 20 '17 at 7:55