Qt async call: how to run something after an async call has finished its job
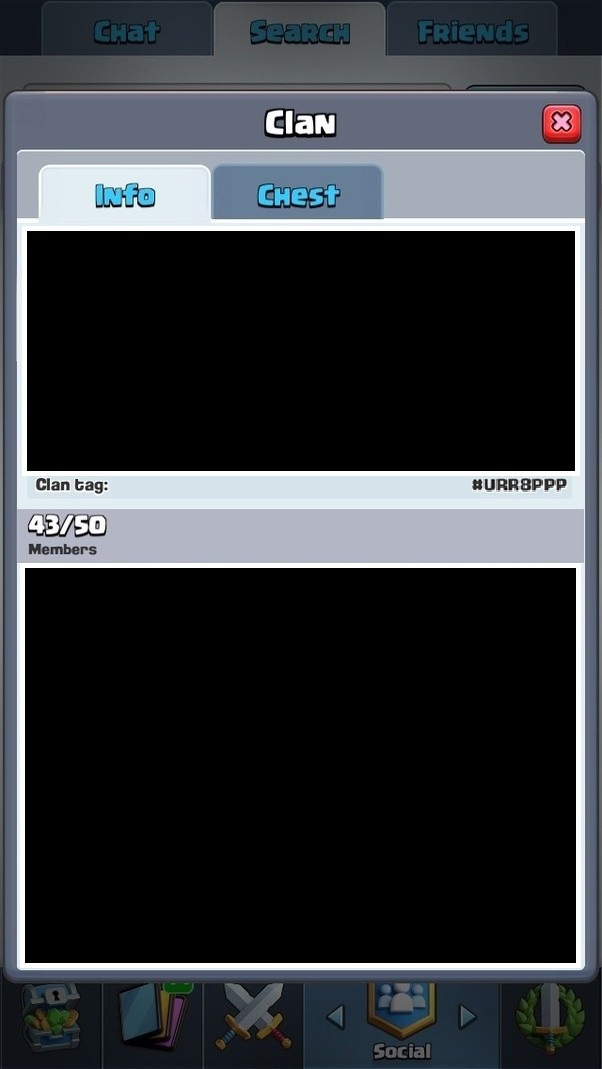

Qt async call: how to run something after an async call has finished its job
I have code like below which does an async call:
QMetaObject::invokeMethod(this, "endSelectionHandling", Qt::QueuedConnection);
I want to modify the code like this:
QMetaObject::invokeMethod(this, "endSelectionHandling", Qt::QueuedConnection);
// I want to add statements here which depend on the result of the above async call.
// How can I wait for the above async call to finish its jobs?
How can I wait for Qt asycn call to finish its job? Is there a better approach?
@Azeem Actually there is more code in between. The original code is written by someone else who is not accessible. Doing the direct blocking call breaks somethings in the application.
– user3405291
yesterday
Right. Please take a look at my answer and example. Hope that helps.
– Azeem
yesterday
1 Answer
1
In your question, it looks like you don't need an asynchronous call at all because you are waiting for the result right after making it an async call.
But, in case you have some code in between, you may use C++11's std::async
to call the function asynchronously and then wait on its std::future
whenever and wherever you need its result after doing other stuff.
std::async
std::future
Here's an example:
#include <iostream>
#include <future>
#include <thread>
#include <chrono>
#define LOG() std::cout << __func__ << " : "
void test()
{
LOG() << "INn";
using namespace std::chrono_literals;
std::this_thread::sleep_for( 1s );
LOG() << "OUTn";
}
int main()
{
LOG() << "Calling test()...n";
auto f = std::async( std::launch::async, test );
LOG() << "Running test()...n";
// ... ...
// ... You can do other stuff here ...
// ... ...
f.wait(); // Blocking call to wait for the result to be available
LOG() << "Exiting...n";
return 0;
}
Here's the output:
main : Calling test()...
main : Running test()...
test : IN
test : OUT
main : Exiting...
Here's the live example: https://ideone.com/OviYU6
UPDATE:
However, in Qt realm, you may want to use QtConcurrent::run
and QFuture
to do things in Qt way.
QtConcurrent::run
QFuture
Here's the example:
#include <QDebug>
#include <QtConcurrent>
#include <QFuture>
#include <QThread>
#define LOG() qDebug() << __func__ << ": "
void test()
{
LOG() << "IN";
QThread::sleep( 1 );
LOG() << "OUT";
}
int main()
{
LOG() << "Calling test()...";
auto f = QtConcurrent::run( test );
LOG() << "Running test()...";
// ... ...
// ... You can do other stuff here ...
// ... ...
f.waitForFinished(); // Blocking call to wait for function to finish
LOG() << "Exiting...";
return 0;
}
Here's the output:
main : Calling test()...
main : Running test()...
test : IN
test : OUT
main : Exiting...
Great. Thanks. I'm going to test this approach.
– user3405291
yesterday
@user3405291: You are welcome! Sure, check this out and kindly accept if it answers your question.
– Azeem
yesterday
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
If you want to wait right after the function call then why don't you call it directly i.e. blocking call? That would be the perfect solution in your case unless there's something in between that you want to do.
– Azeem
yesterday