return float array from a function c++
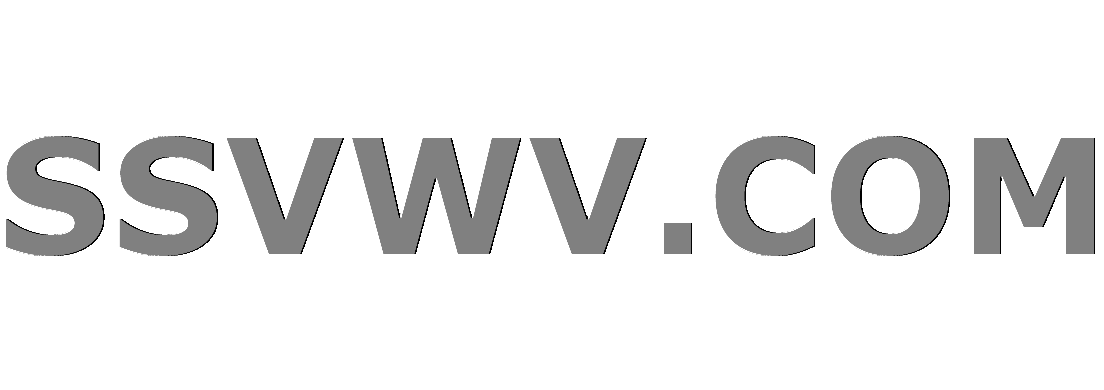
Multi tool use
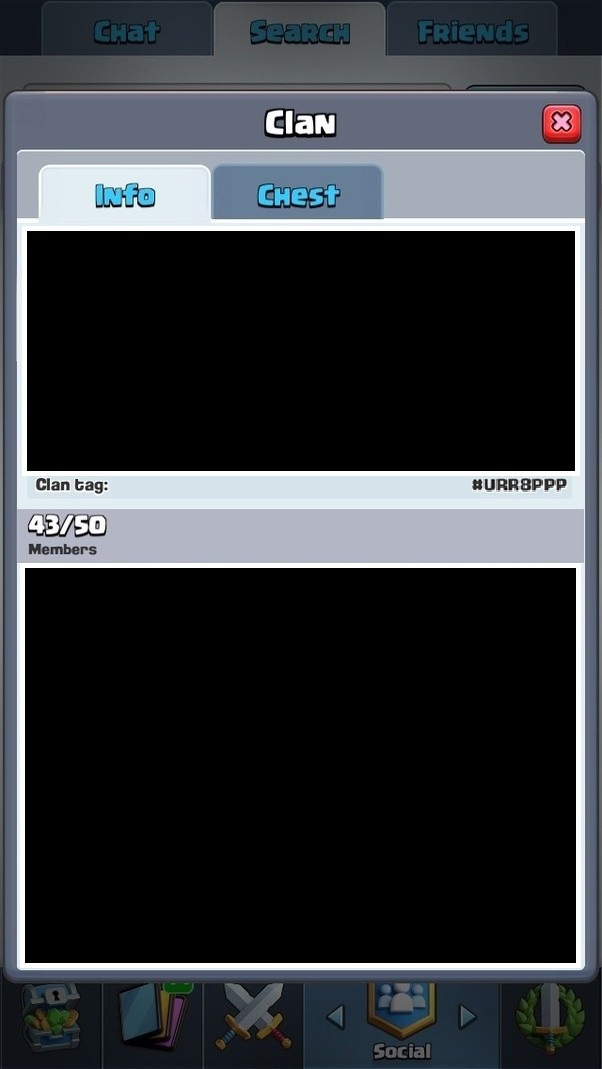

return float array from a function c++
What am I doing wrong?
In the first print the values are fain, in the second it's all zeros.
The first print - q: 0.965926 0.000000 0.258819 0.000000
The Second print - q: 0.000000 0.000000 0.000000 0.000000
float *AnglesToQaternion(float roll, float pitch, float yaw) { // Convert Euler angles in degrees to quaternions
static float q[4];
roll = roll * DEG_TO_RAD;
pitch = pitch * DEG_TO_RAD;
yaw = yaw * DEG_TO_RAD;
float t0 = cosf(yaw * 0.5);
float t1 = sinf(yaw * 0.5);
float t2 = cosf(roll * 0.5);
float t3 = sinf(roll * 0.5);
float t4 = cosf(pitch * 0.5);
float t5 = sinf(pitch * 0.5);
q[0] = t0 * t2 * t4 + t1 * t3 * t5;
q[1] = t0 * t3 * t4 - t1 * t2 * t5;
q[2] = t0 * t2 * t5 + t1 * t3 * t4;
q[3] = t1 * t2 * t4 - t0 * t3 * t5;
printf(">> q-1: %f %f %f %f n", q[0], q[1], q[2], q[3]);
return q;
}
float q[4] = *AnglesToQaternion(0, 30.0, 0);
printf(">> q-2: %f %f %f %f n", q[0], q[1], q[2], q[3]);
Why is this a C++ question? All I see is C
– hellow
1 hour ago
float q[4] = *AnglesToQaternion(0, 30.0, 0);
should be float *q = AnglesToQaternion(0, 30.0, 0);
– Susmit Agrawal
1 hour ago
float q[4] = *AnglesToQaternion(0, 30.0, 0);
float *q = AnglesToQaternion(0, 30.0, 0);
1) Don't use
static
for local variables. 2) don't return pointers to locals 3) use C++ arrays std::array<float, 4>
– Dmitry Sazonov
1 hour ago
static
std::array<float, 4>
Possible duplicate of Returning an array using C
– Sander De Dycker
1 hour ago
6 Answers
6
Solution 1: You have to create your array dynamically inside your function if you want to be able to access it from outside. Then you must think to delete it to avoid memory leaks.
float *AnglesToQaternion(float roll, float pitch, float yaw) { // Convert Euler angles in degrees to quaternions
float *q = new float[4];
// Or C-style: float *q = malloc(sizeof(float)*4);
roll = roll * DEG_TO_RAD;
pitch = pitch * DEG_TO_RAD;
yaw = yaw * DEG_TO_RAD;
float t0 = cosf(yaw * 0.5);
float t1 = sinf(yaw * 0.5);
float t2 = cosf(roll * 0.5);
float t3 = sinf(roll * 0.5);
float t4 = cosf(pitch * 0.5);
float t5 = sinf(pitch * 0.5);
q[0] = t0 * t2 * t4 + t1 * t3 * t5;
q[1] = t0 * t3 * t4 - t1 * t2 * t5;
q[2] = t0 * t2 * t5 + t1 * t3 * t4;
q[3] = t1 * t2 * t4 - t0 * t3 * t5;
printf(">> q-1: %f %f %f %f n", q[0], q[1], q[2], q[3]);
return q;
}
int main() {
float *q = AnglesToQaternion(0, 30.0, 0);
printf(">> q-2: %f %f %f %f n", q[0], q[1], q[2], q[3]);
delete q;
// Or C-style: free(q);
}
Solution 2: You can make a static array creation and then pass the address of its first element to your function.
void AnglesToQaternion(float *q, float roll, float pitch, float yaw) { // Convert Euler angles in degrees to quaternions
roll = roll * DEG_TO_RAD;
pitch = pitch * DEG_TO_RAD;
yaw = yaw * DEG_TO_RAD;
float t0 = cosf(yaw * 0.5);
float t1 = sinf(yaw * 0.5);
float t2 = cosf(roll * 0.5);
float t3 = sinf(roll * 0.5);
float t4 = cosf(pitch * 0.5);
float t5 = sinf(pitch * 0.5);
q[0] = t0 * t2 * t4 + t1 * t3 * t5;
q[1] = t0 * t3 * t4 - t1 * t2 * t5;
q[2] = t0 * t2 * t5 + t1 * t3 * t4;
q[3] = t1 * t2 * t4 - t0 * t3 * t5;
printf(">> q-1: %f %f %f %f n", q[0], q[1], q[2], q[3]);
}
int main() {
float q[4];
AnglesToQaternion(q, 0, 30.0, 0);
printf(">> q-2: %f %f %f %f n", q[0], q[1], q[2], q[3]);
}
This second solution is much better for performance (you should avoid new/delete as much as you can if you can).
How can you send to a function local and accept it as a pointer on your second solution?
– Gal Dalali
38 mins ago
When you write
float q[4];
, q
is of type float*
if this is what you are asking. q
represents the address of the first cell of your float array.– Benjamin Barrois
35 mins ago
float q[4];
q
float*
q
Oh, I accidently left the "return q;", finally, Thanks a lot
– Gal Dalali
26 mins ago
Don't return an array at all.
using degree = float;
struct Angles {
degree roll;
degree pitch;
degree yaw;
};
struct Quaternion {
float i;
float j;
float k;
float l;
};
Quaternion angles_to_quaternion(Angles angles)
{
float yaw = angles.yaw * DEG_TO_RAD;
float pitch = angles.pitch * DEG_TO_RAD;
float roll = angles.roll * DEG_TO_RAD;
float t0 = cosf(yaw * 0.5);
float t1 = sinf(yaw * 0.5);
float t2 = cosf(roll * 0.5);
float t3 = sinf(roll * 0.5);
float t4 = cosf(pitch * 0.5);
float t5 = sinf(pitch * 0.5);
return {
t0 * t2 * t4 + t1 * t3 * t5,
t0 * t3 * t4 - t1 * t2 * t5,
t0 * t2 * t5 + t1 * t3 * t4,
t1 * t2 * t4 - t0 * t3 * t5,
};
}
You should define the function and q like this:
#include <vector>
std::vector<float> AnglesToQaternion(float roll, float pitch, float yaw)
{
std::vector<float> q{ 0,0,0,0 };
And to use the function like this:
std::vector<float> q{ 0,0,0,0 };
q = AnglesToQaternion(0, 30.0, 0);
Pass the address of the local q in main() to AnglesToQaternion for it to populate.
void AnglesToQaternion(float roll, float pitch, float yaw, float * quaternion)
Use pointers. Make sure you are freeing allocated memory.
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
float *AnglesToQaternion(float roll, float pitch, float yaw);
int main()
{
float *q1 = AnglesToQaternion(0, 30.0, 0);
printf(">> q-2: %f %f %f %f n", q1[0], q1[1], q1[2], q1[3]);
free(q1);
return 0;
}
float *AnglesToQaternion(float roll, float pitch, float yaw) { // Convert Euler angles in degrees to quaternions
float *p = (float *)malloc(sizeof(float)*4) ;
roll = roll * DEG_TO_RAD;
pitch = pitch * DEG_TO_RAD;
yaw = yaw * DEG_TO_RAD;
float t0 = cosf(yaw * 0.5);
float t1 = sinf(yaw * 0.5);
float t2 = cosf(roll * 0.5);
float t3 = sinf(roll * 0.5);
float t4 = cosf(pitch * 0.5);
float t5 = sinf(pitch * 0.5);
p[0] = t0 * t2 * t4 + t1 * t3 * t5;
p[1] = t0 * t3 * t4 - t1 * t2 * t5;
p[2] = t0 * t2 * t5 + t1 * t3 * t4;
p[3] = t1 * t2 * t4 - t0 * t3 * t5;
printf(">> q-1: %f %f %f %f n", p[0],p[1], p[2], p[3]);
return p;
}
Thanks, but my main question is how at the end I can do: float q[4] = q1 // q1 is a pointer
– Gal Dalali
47 mins ago
@GalDalali You can't copy arrays in C++ unless you use a function such as
memcpy
or std::copy
– john
44 mins ago
memcpy
std::copy
Why you are using array?
– mfromla
40 mins ago
As Mentioned in one of the comment under your question, you cannot really return an array, but a pointer. If you really want it as return value, then make sure you allocate memory in that function for that before using it. Something like:
float *f_array = malloc(sizeof(float)*lengthArray);
float *f_array = malloc(sizeof(float)*lengthArray);
An alternative is to pass an array as argument to your function and fill it in the function.
void AnglesToQaternion(float roll, float pitch, float yaw, float resultArray)
void AnglesToQaternion(float roll, float pitch, float yaw, float resultArray)
Thanks, but my main question is how at the end I can do: float q[4] = AnglesToQaternion, I don't want to work with array.
– Gal Dalali
38 mins ago
You simply can't. You need to copy content of the array(returned pointer) into the new array. However, you can do
float *q = AnglesToQaternion(...);
Provided that you have allocated memory in the function.– PhoenixBlue
34 mins ago
float *q = AnglesToQaternion(...);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You can't return an (raw, C-style) array from a function in C++. The closest you can come to it is to return a pointer.
– Ron
1 hour ago