Specify equality comparer for model binding to collection types
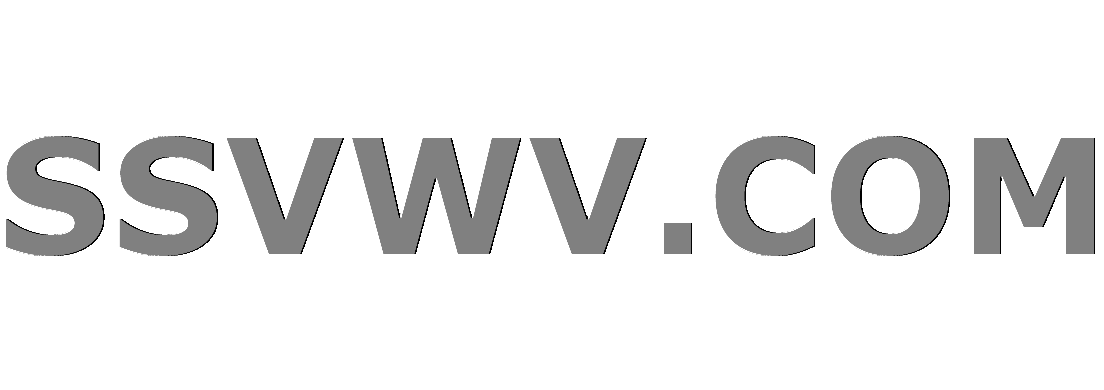
Multi tool use
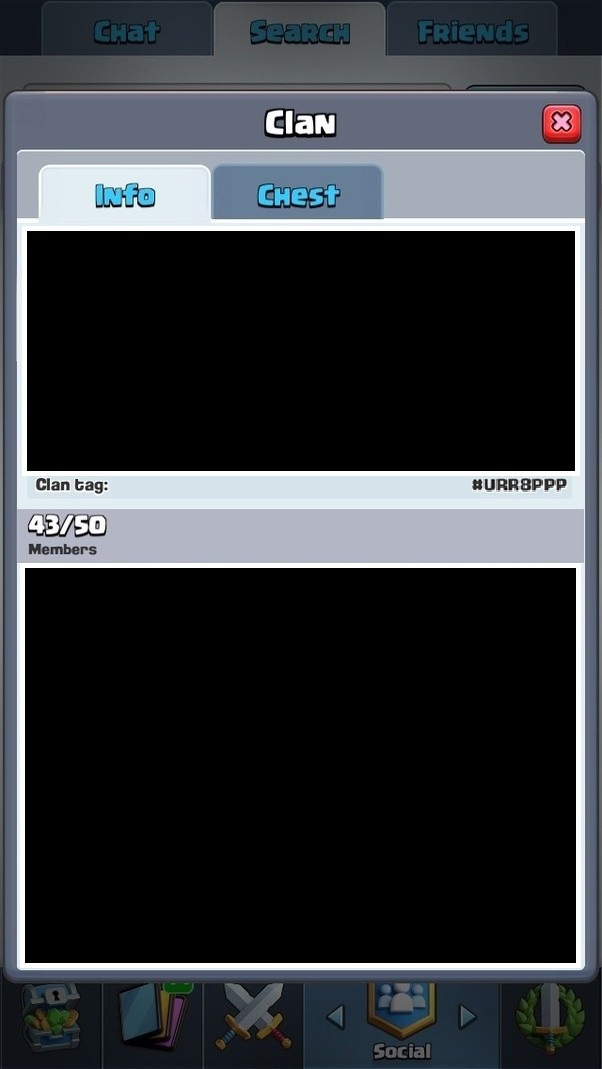

Specify equality comparer for model binding to collection types
I have an API where you can specify a list of names to get. Duplicate names are not allowed and if two names differ only by casing then they are considered duplicates.
GET /api/people?names=john&names=alice
In my own .NET code I would gather all these names in a HashSet
with a custom equality comparer.
HashSet
var names = new HashSet<string>(StringComparer.OrdinalIgnoreCase);
But I don't think ASP.NET Core model binding is flexible enough for that.
// GET /api/people?names=john&names=JOHN
[HttpGet("api/people")]
public GetPeople([FromQuery] HashSet<string> names)
{
// this works but names contains both john and JOHN
}
What do I have to change so that the names
set only contains john and not JOHN?
names
2 Answers
2
You can use a List<string>
in the method header, and then load it into a HashSet according to your rules:
List<string>
public GetPeople([FromQuery] List<string> names)
{
var hashed = new HashSet<string>(names, StringComparer.OrdinalIgnoreCase);
}
As an alternative you could look into custom Model Binding, but it may only be worth the effort if you need it in multiple places.
You can receive it in Json Serialize format. Just change your calling method
GET /api/people?names={"john","alice"}
Then need to deserialize
Can you give a c# example for deserializing without duplicates?
– Steven Liekens
18 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
That's not valid json
– Steven Liekens
19 mins ago