Terraform Module with Count Can't Have Duplicate Elements
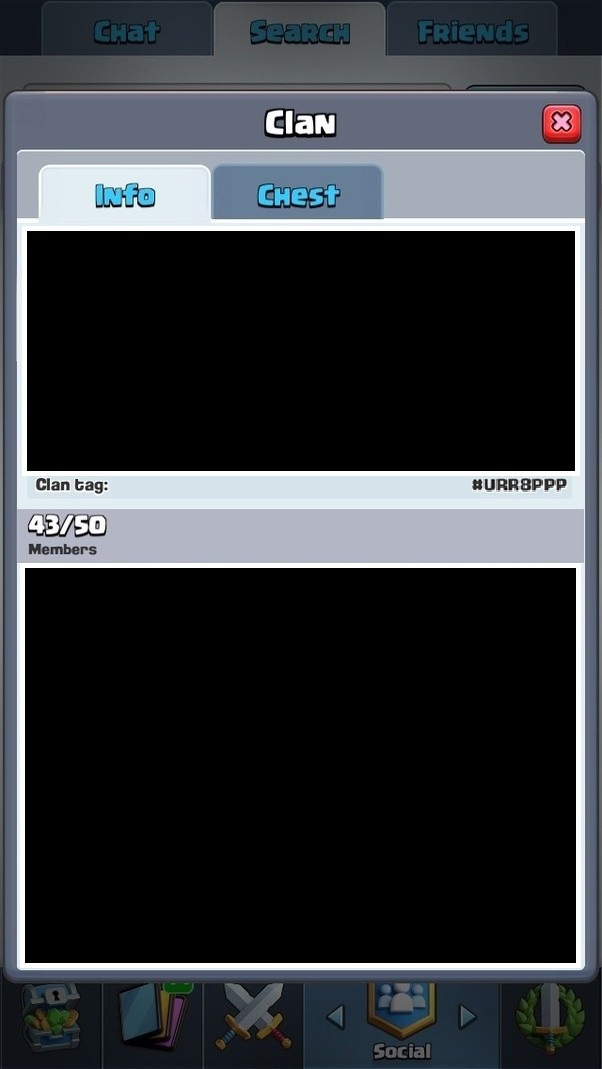

Terraform Module with Count Can't Have Duplicate Elements
Tricky one to explain in a title, or even form a question around it, so I'll start with some code (simplified for, err, simplicity):
resource "digitalocean_domain" "this_domain" {
name = "${var.domain}"
ip_address = "${var.main_ip}"
}
resource "digitalocean_record" "this_a_record" {
count = "${length(var.a_records)}"
domain = "${var.domain}"
type = "A"
name = "${element(keys(var.a_records), count.index)}"
value = "${lookup(var.a_records, element(keys(var.a_records), count.index))}"
}
Given the above being part of a module called dns
, I can call it like this:
dns
module "example_com_dns" {
source = "./modules/dns"
domain = "example.com"
main_ip = "1.2.3.4"
a_records = {
"@" = "5.6.7.8"
"self" = "9.10.11.12"
"www" = "5.6.7.8"
}
}
Running this works as expected. I get the A
records I expect; @, self, www, all pointing to the correct IPs.
A
However, it can't handle duplicate names. For example, putting in multiple @
records results in only one of them being written, I'm guessing because each iteration for the name simply overwrites the previous @
record.
@
@
Is there a way to have multiple duplicate names? i.e. In the example above, have something like:
....
"@" = "5.6.7.8"
"@" = "20.21.22.23"
"@" = "30.31.32.33"
"self" = "9.10.11.12"
"www" = 5.6.7.8"
...
1 Answer
1
In that case instead of using keys to get map keys you should put the A records into a list like:
a_records = [
["@" , "5.6.7.8"],
["@" , "7.6.7.8"],
["@" , "9.9.9.9"],
["self", "7.10.11.12"],
["self", "11.11.111.11"],
["self", "12.12.12.12"],
["self", "13.13.13.13"],
["www" , "14.14.14.14"]
]
and assign name and value as
name = "${element(var.a_records[count.index],0)}"
value = "${element(var.a_records[count.index],1)}"
and you will get all the duplicate A records that you want
In that case instead of using keys to get map keys you should put the A records into a list like:
code
a_records = [ ["@" , "5.6.7.8"], ["@" , "7.6.7.8"], ["@" , "9.9.9.9"], ["self", "7.10.11.12"], ["self", "11.11.111.11"], ["self", "12.12.12.12"], ["self", "13.13.13.13"], ["www" , "14.14.14.14"] ] code
and assign “name” and “value “ as code
name = "${element(var.a_records[count.index],0)}" value = "${element(var.a_records[count.index],1)}" code
and you will get all the duplicate A records that you want.– Don
16 mins ago
code
code
code
code
@Don You should update your answer accordingly as this seems to be the solution.
– Markus
12 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Thanks for taking the time to answer :) Putting aside for the moment the argument that @ A records need to be unique or not (I don't think they do: tools.ietf.org/html/rfc1035), I'm asking specifically how to achieve this in Terraform. Doing the same thing outside of a loop works perfectly (multiple "@" A records are created).
– Afraz
yesterday