I'm trying to work with Graphics and draw some Rectangles
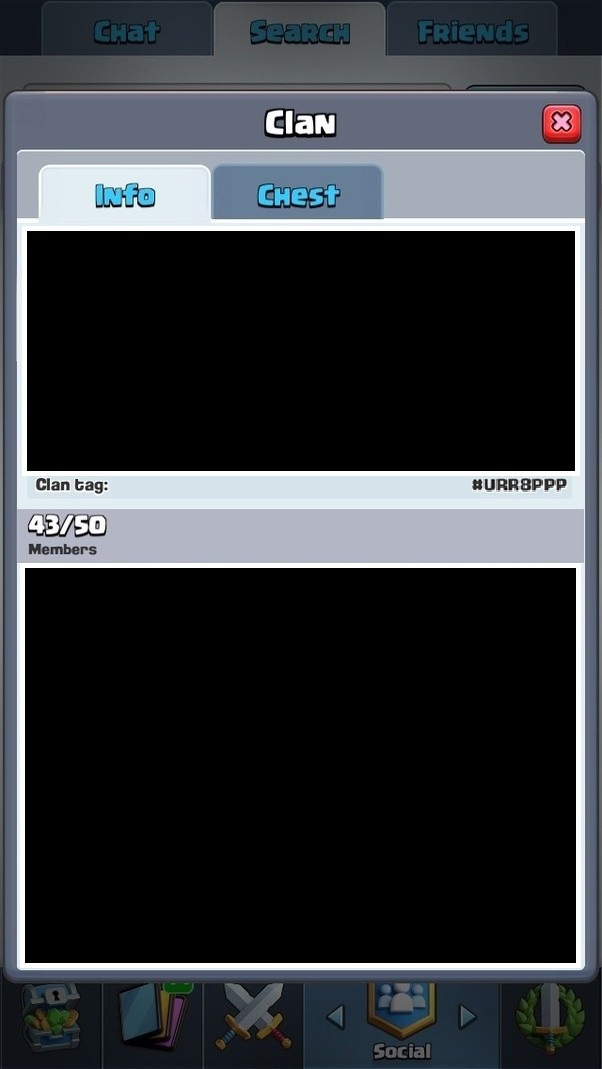

I'm trying to work with Graphics and draw some Rectangles
Hello i'm trying to learn Graphics in java and at the same time Classes and Objects. My goal now is to make a programm that contains different classes with Rectangles or Circles and then I want to use those Rectangles and Circles in other classes and change their parameters like size, color and position to draw some kind of Pattern.
My problem right now is that I can make a rectangle and I think I can even make a second one, but i can't change parameters of it (Color, size and position) I tried adding variables to this part of code Rect rect = new Rect(int variables);
but it didn't work.
Rect rect = new Rect(int variables);
Normally I can solve easy problems like this but i really don't understand how classes and objects works in java if someone can give me some help would be great.
Here is my code
public class Main{
public static void main(String args ) {
Pattern.drawPattern();
}
}
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JPanel;
public class Rect extends JPanel{
public static Color myColor = Color.RED;
public static int myX = 10;
public static int myY = 10;
public static int myWidth = 200;
public static int myHeight = 200;
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(myColor);
g.fillRect(myX, myY, myWidth, myHeight);
}
}
import java.awt.Color;
import java.awt.Container;
import javax.swing.JFrame;
public class Pattern {
public static void drawPattern() {
JFrame window = new JFrame("test");
window.setSize(1000, 800);
window.setVisible(true);
window.setResizable(false);
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Rect rect = new Rect();
Rect rect1 = new Rect();
window.add(rect);
window.add(rect1);
Rect.myColor = Color.lightGray;
}
}
Hmm put everything in one class in IntelliJ works great. But maybe it helps if you trigger the repaint manually. Because thats what you need to do if you change the properties of the content you draw.
– sascha10000
3 hours ago
Please see update to answer. Please ask if any questions
– Hovercraft Full Of Eels
1 hour ago
1 Answer
1
So many problems here, but main ones I can see are:
ArrayList<Rect>
Minor quibbles:
For instance, Rect (or here named Rect2 to show it's different from your class) could look something like:
// imports here
public class Rect2 {
private Color myColor = Color.RED;
private int x = 10;
private int y = x;
private int width = 200;
private int height = width;
public Rect2() {
// default constructor
}
public Rect2(Color myColor, int x, int y, int width, int height) {
this.myColor = myColor;
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
// method used to allow the rectangle to be drawn
public void draw(Graphics g) {
g.setColor(myColor);
g.fillRect(x, y, width, height);
}
public void setMyColor(Color myColor) {
this.myColor = myColor;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
// more setters and some getters if need be
}
and the drawing JPanel something like:
// imports here
public class DrawingPanel extends JPanel {
private static final long serialVersionUID = 1L;
private List<Rect2> rectList = new ArrayList<>();
// ..... more code
public void addRect2(Rect2 rect) {
rectList.add(rect);
repaint();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// iterate through the rectList and draw all the Rectangles
for (Rect2 rect : rectList) {
rect.draw(g);
}
}
// ...... more code
}
and it could be put into a JFrame like so....
Rect2 rectA = new Rect2();
Rect2 rectB = new Rect2();
rectB.setMyColor(Color.BLUE);
rectB.setX(300);
rectB.setY(300);
// assuming that the class's constructor allows sizing parameters
DrawingPanel drawingPanel = new DrawingPanel(1000, 800);
drawingPanel.addRect2(rectA);
drawingPanel.addRect2(rectB);
JFrame frame = new JFrame("Main2");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(drawingPanel);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
See: Custom Painting Approaches
– camickr
5 hours ago