PHP preg_replace error when using array
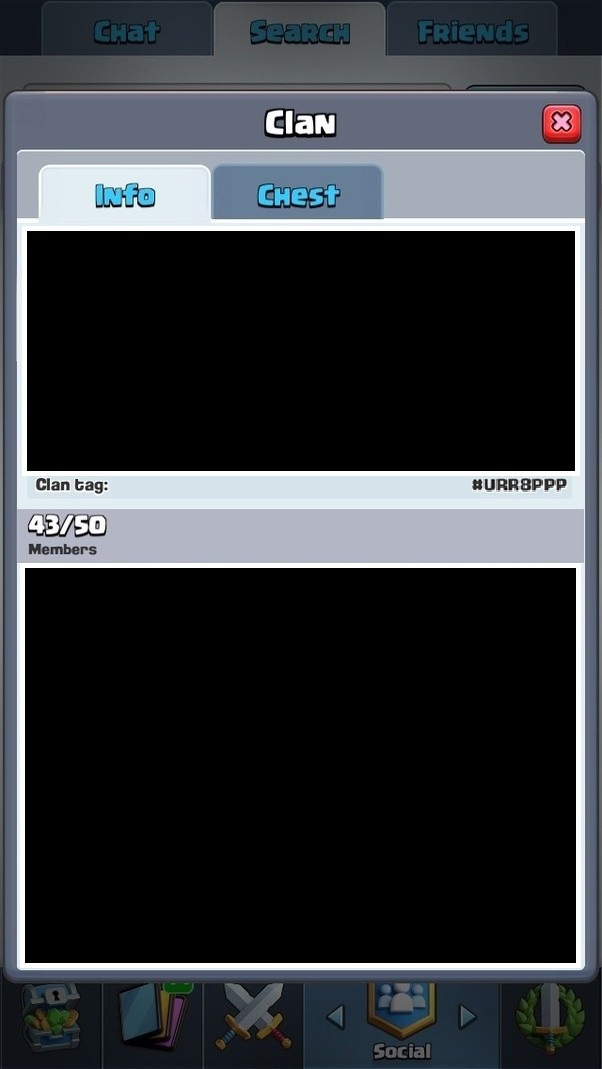

PHP preg_replace error when using array
We have got web app which does replacing some text with another using str_replace().
Find strings and replace strings are stored in template file.
We what to replace str_replace() function to preg_replace() to have possibility to use regex in find strings (to set them in the same template file).
In original scripts we have such parts of php code.
In one file:
class SiteConfig {
// Strings to search for in HTML before processing begins (used with $replace_string)
public $find_string = array();
// Strings to replace those found in $find_string before HTML processing begins
public $replace_string = array();
// a lot of code goes here
public function append(SiteConfig $newconfig) {
foreach (array('find_string', 'replace_string') as $var) {
// append array elements for this config variable from $newconfig to this config
//$this->$var = $this->$var + $newconfig->$var;
$this->$var = array_merge($this->$var, $newconfig->$var);
}
}
// a lot of code goes here
public static function build_from_array(array $lines) {
$config = new SiteConfig();
foreach ($lines as $line) {
$line = trim($line);
// skip comments, empty lines
if ($line == '' || $line[0] == '#') continue;
// get command
$command = explode(':', $line, 2);
// if there's no colon ':', skip this line
if (count($command) != 2) continue;
$val = trim($command[1]);
$command = trim($command[0]);
//if ($command == '' || $val == '') continue;
// $val can be empty, e.g. replace_string:
if ($command == '') continue;
// strip_attr is now an alias for strip.
// In FTR 3.8 we can strip attributes from elements, not only the elements themselves
// e.g. strip: //img/@srcset (removes srcset attribute from all img elements)
// but for backward compatibility (to avoid errors with new config files + old version of FTR)
// we've introduced strip_attr and we'll recommend using that in our public site config rep.
// strip_attr: //img/@srcset
if ($command == 'strip_attr') $command = 'strip';
// check for commands where we accept multiple statements
if (in_array($command, array('title', 'body', 'author', 'date', 'strip', 'strip_id_or_class', 'strip_image_src', 'single_page_link', 'single_page_link_in_feed', 'next_page_link', 'native_ad_clue', 'http_header', 'test_url', 'find_string', 'replace_string'))) {
array_push($config->$command, $val);
// check for single statement commands that evaluate to true or false
} elseif (in_array($command, array('tidy', 'prune', 'autodetect_on_failure', 'insert_detected_image'))) {
$config->$command = ($val == 'yes');
// check for single statement commands stored as strings
} elseif (in_array($command, array('parser'))) {
$config->$command = $val;
// special treatment for test_contains
} elseif (in_array($command, array('test_contains'))) {
$config->add_test_contains($val);
// special treatment for if_page_contains
} elseif (in_array($command, array('if_page_contains'))) {
$config->add_if_page_contains_condition($val);
// check for replace_string(find): replace
} elseif ((substr($command, -1) == ')') && preg_match('!^([a-z0-9_]+)((.*?))$!i', $command, $match)) {
if (in_array($match[1], array('replace_string'))) {
array_push($config->find_string, $match[2]);
array_push($config->replace_string, $val);
} elseif (in_array($match[1], array('http_header'))) {
$_header = strtolower(trim($match[2]));
$config->http_header[$_header] = $val;
}
}
}
return $config;
}
}
In another file:
public function process($html, $url, $smart_tidy=true) {
// a lot of code goes before
// do string replacements
if (!empty($this->config->find_string)) {
if (count($this->config->find_string) == count($this->config->replace_string)) {
$html = str_replace($this->config->find_string, $this->config->replace_string, $html, $_count);
$this->debug("Strings replaced: $_count (find_string and/or replace_string)");
} else {
$this->debug('Skipped string replacement - incorrect number of find-replace strings in site config');
}
unset($_count);
}
// a lot of code goes after
}
I tried to replace str_replace() with preg_replace(), but while testing it shows an error:
Warning: preg_replace(): No ending matching delimiter '>' found in this line:
$html = preg_replace($this->config->find_string, $this->config->replace_string, $html, $_count);
Where is the error and how to replace str_replace() function to preg_replace() correctly?
I'm very very beginning in php, so any help is badly needed.
Big thanks in advance!
preg_replace
str_replace
'/'.preg_quote($this->config->find_string).'/'
This error goes even I have no any regular expression string in template.
– Jesty
2 hours ago
regex in php expects a leading and trailing character as part of the pattern, eg to match
hello
your expression would be /hello/
php.net/manual/en/regexp.reference.delimiters.php– Scuzzy
2 hours ago
hello
/hello/
Yes, of cause, when I tried to set regular expression in template it was: find_string: /teststing/
– Jesty
2 hours ago
You're going to have to us show what's in
$this->config->find_string
and $this->config->replace_string
i'd say.– Scuzzy
2 hours ago
$this->config->find_string
$this->config->replace_string
1 Answer
1
Try
$new_config_find_string = array_map(function($new_pattern)
{
return '/'.$new_pattern.'/';
},$this->config->find_string);
$html = preg_replace($new_config_find_string, $this->config->replace_string, $html, $_count);
@Ersian it gives
Warning: preg_quote() expects parameter 1 to be string, array given in
that string– Jesty
3 mins ago
Warning: preg_quote() expects parameter 1 to be string, array given in
check my code, no more
preg_quotes
– Erisan Olasheni
1 min ago
preg_quotes
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
With that kind of error, we're going to need to see how you're constructing your regular expression string.
preg_replace
is not a "drop in" replacement forstr_replace
, at minimum you may need'/'.preg_quote($this->config->find_string).'/'
for non regex based patterns, but then that's just silly and has no benefit over the former.– Scuzzy
3 hours ago