Polynomials and dictionaries
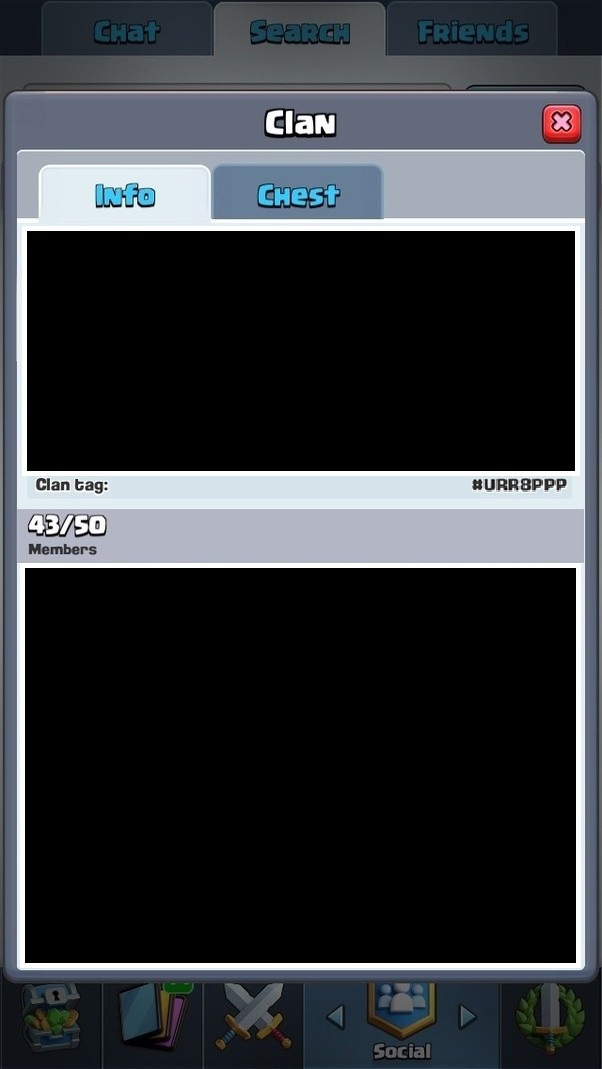

Polynomials and dictionaries
i have this exercise about polynomials and dictionaries which i did (see below) but i am sure there is a better and easier way to solve it (for question 2 and 3). can anyone show me another way to approach question 2 or 3? Thanks.
here is the exercice:
In this exercise we want to work with polynomials of any degree. Each polynomial can be represented by a dictionary, whose keys correspond to the powers of x, and the values to the coefficients. For example, to represent the polynomial x ^ 6 + 3 * x ^ 2, we can use the dictionary: {6: 1, 2: 3}
1. Write a function evaluer(p, x) that takes a polynomial p and a number x into arguments, and returns the value of polynomial at point x.
Execution example:
evaluer({3: 1, 1: 2, 0: -1}, 2)
OUT: 11
2. Write a function somme_polynomes(p1, p2) which takes two polynomials (dictionaries) into arguments and which
returns a new dictionary representing the sum of the two polynomials p1 and p2.
Execution example:
somme_polynomes ({3: 1, 2: 1, 0: 1}, {4: 2, 2: 3})
OUT: {0: 1, 2: 4, 3: 1, 4: 2}
3. Write a function produit_polynomes(p1, p2) that takes two polynomials as arguments and returns the product of two polynomials in a new dictionary.
Execution example:
produit_polynomes ({3: 1, 2: 1, 0: 1}, {4: 2, 2: 3})
OUT: {2: 3, 4: 5, 5: 3, 6: 2, 7: 2}
here is what i did:
# 1)
def evaluer(p,x):
c = 0
for key,value in p.items():
c += value*(x**key)
return c
# 2)
def somme_polynomes(p1,p2):
p3 = {}
for key,value in p1.items():
for k,v in p2.items():
p3.update({key:value})
p3.update({k:v})
for key in p1:
if key in p2:
add = p1[key]+p2[key]
p3.update({key:add})
if add == 0:
del p3[key]
return p3
# 3)
def produit_polynomes(p1,p2):
p3 = {}
for key,value in p1.items():
for k,v in p2.items():
if key+k in p3:
p3[key+k] += value*v
else:
p3.update({key+k:value*v})
return p3
Your code looks good to me!
– bstrauch24
25 mins ago
2 Answers
2
Your code is fine, here are alternative ways of doing it using more of Python's language (generator expression, dict comprehension) and library (itertools
, collections
):
itertools
collections
def evaluer(p, x):
return sum(v * x**k for k, v in p.items())
def somme_polynomes(p1, p2):
return {k: p1.get(k, 0) + p2.get(k, 0) for k in set(p1) | set(p2)}
import itertools as it
from collection import defaultdict
def produit_polynomes(p1, p2):
p3 = defaultdict(int)
for k1, k2 in it.product(p1, p2):
p3[k1+k2] += p1[k1]*p2[k2]
return dict(p3)
If you want to avoid importing any modules then produit_polnomes()
could be written without the conditional as:
produit_polnomes()
def produit_polynomes(p1,p2):
p3 = {}
for k1, v1 in p1.items():
for k2, v2 in p2.items():
p3[k1+k2] = p3.get(k1+k2, 0) + v1*v2
return p3
Exercise 2 can be done in a more Pythonic way by using set union for keys and dict comprehension for sums:
def somme_polynomes(p1, p2):
return {p: p1.get(p, 0) + p2.get(p, 0) for p in set(p1) | set (p2)}
Exercise 3 on the other hand is best done using nested loops aggregating products to the sum of keys, which is what you are already doing. The only slight enhancement I would make is to use the setdefault
method to avoid the if
statement:
setdefault
if
def produit_polynomes(p1,p2):
p3 = {}
for key,value in p1.items():
for k,v in p2.items():
p3[key + k] = p3.get(key + k, 0) + value * v
return p3
You can't use
setdefault()
this way.– AChampion
6 mins ago
setdefault()
You're right. Edited accordingly. Thanks.
– blhsing
4 mins ago
You still don't need
setdefault()
, a simple get()
would do the same.– AChampion
3 mins ago
setdefault()
get()
Yeah. Wasn't thinking straight. Thanks.
– blhsing
2 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
So what do you want us to do? Write the code for you? I suggest you write a more specific question.
– Yohst
30 mins ago