Adding Text and Value to ComboBox in WPF Application
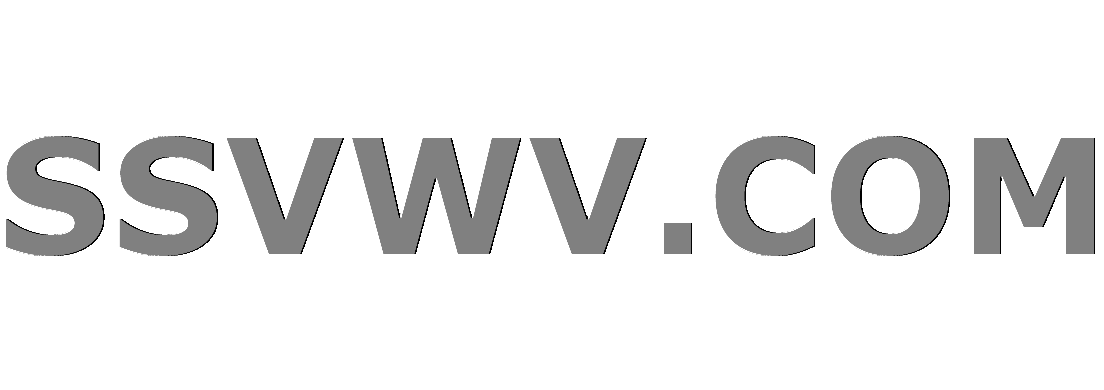
Multi tool use
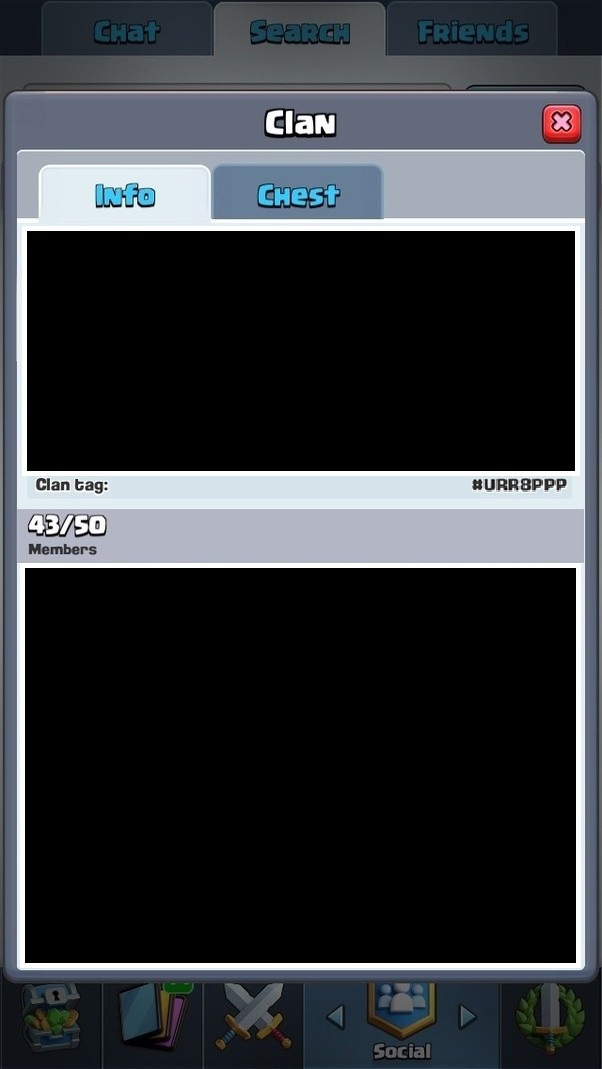

Adding Text and Value to ComboBox in WPF Application
Following code is okey in vb.net and Windows Form Application.
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim comboSource As New Dictionary(Of String, String)()
comboSource.Add("1", "Sunday")
comboSource.Add("2", "Monday")
comboSource.Add("3", "Tuesday")
comboSource.Add("4", "Wednesday")
comboSource.Add("5", "Thursday")
comboSource.Add("6", "Friday")
comboSource.Add("7", "Saturday")
ComboBox1.DataSource = New BindingSource(comboSource, Nothing)
ComboBox1.DisplayMember = "Value"
ComboBox1.ValueMember = "Key"
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim key As String = DirectCast(ComboBox1.SelectedItem, KeyValuePair(Of String, String)).Key
Dim value As String = DirectCast(ComboBox1.SelectedItem, KeyValuePair(Of String, String)).Value
MessageBox.Show(key & " " & value)
End Sub
End Class
I want to convert above codes to WPF Application.
xaml
<Window x:Class="MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<ComboBox x:Name="ComboBox1" Height="20" Width="150"/>
<Button x:Name="Button1" Height="20" Width="80" VerticalAlignment="Top" Content="Click Me"/>
</Grid>
</Window>
vb.net
Class MainWindow
Private Sub MainWindow_Loaded(sender As Object, e As RoutedEventArgs) Handles Me.Loaded
Dim comboSource As New Dictionary(Of String, String)()
comboSource.Add("1", "Sunday")
comboSource.Add("2", "Monday")
comboSource.Add("3", "Tuesday")
comboSource.Add("4", "Wednesday")
comboSource.Add("5", "Thursday")
comboSource.Add("6", "Friday")
comboSource.Add("7", "Saturday")
ComboBox1.DataSource = New BindingSource(comboSource, Nothing)
ComboBox1.DisplayMember = "Value"
ComboBox1.ValueMember = "Key"
End Sub
Private Sub Button1_Click(sender As Object, e As RoutedEventArgs) Handles Button1.Click
Dim key As String = DirectCast(ComboBox1.SelectedItem, KeyValuePair(Of String, String)).Key
Dim value As String = DirectCast(ComboBox1.SelectedItem, KeyValuePair(Of String, String)).Value
MessageBox.Show(key & " " & value)
End Sub
End Class
Linked picture shows the errors: https://prnt.sc/kcig7j
So, how can I solve that errors?
1 Answer
1
The WPF equivalents for the WinForms properties are as follows:
DataSource -> ItemsSource
DisplayMember -> DisplayMemberPath
ValueMember -> SelectedValuePath
Also, bear in mind that you can set properties in either code-behind or in XAML, so your ComboBox
definition could look like this:
ComboBox
<ComboBox x:Name="ComboBox1" Height="20" Width="150" DisplayMemberPath="Value" SelectedValuePath="Key" />
I personally would find it easier to set the ItemsSource
property in code-behind:
ItemsSource
Dim comboSource As New Dictionary(Of String, String)()
comboSource.Add("1", "Sunday")
comboSource.Add("2", "Monday")
comboSource.Add("3", "Tuesday")
comboSource.Add("4", "Wednesday")
comboSource.Add("5", "Thursday")
comboSource.Add("6", "Friday")
comboSource.Add("7", "Saturday")
ComboBox1.ItemsSource = comboSource
W.R.T. your event handler, you have a double cast to KeyValuePair(Of String, String)
; you should probably cast only once and store the casted value in a variable. But in this particular case, you don't have to even cast the SelectedItem
. Instead, you can cast the sender
to a ComboBox
and read the Text
and Value
properties:
KeyValuePair(Of String, String)
SelectedItem
sender
ComboBox
Text
Value
Dim cmb As ComboBox = sender
MessageBox.Show($"Key: {cmb.SelectedValue}, Value: {cmb.Text}')
Also note that you can just as easily use an Integer
for your dictionary key as a String
:
Integer
String
Dim days = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}
Dim comboSource = days
.Select(Function(x, index) (x, index+1))
.ToDictionary(Function(x) x.Item2, Function(x) x.Item1)
Thanks a lot. Solved.
– Mark Markowitz
24 mins ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
WPF and WinForms are two completely different UI frameworks. You can't convert a WinForms application to WPF without basic knowledge about how WPF works. Read a WPF book before you continue.
– Clemens
1 hour ago