Java Prime Number Method
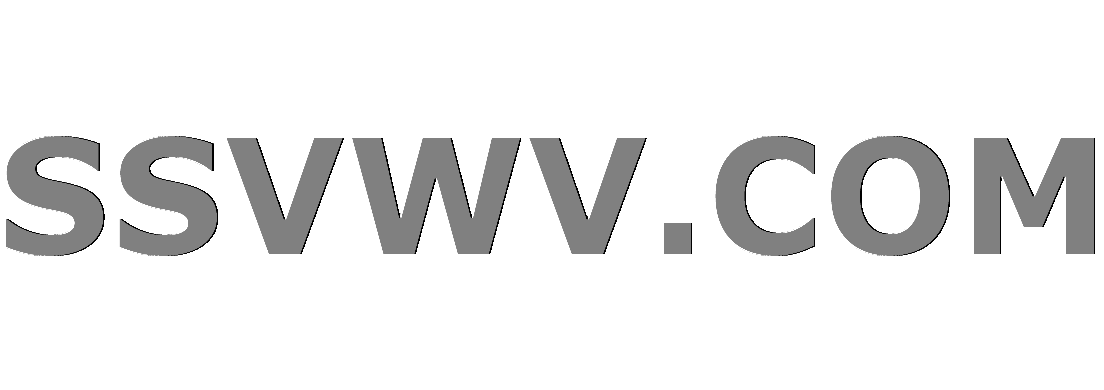
Multi tool use
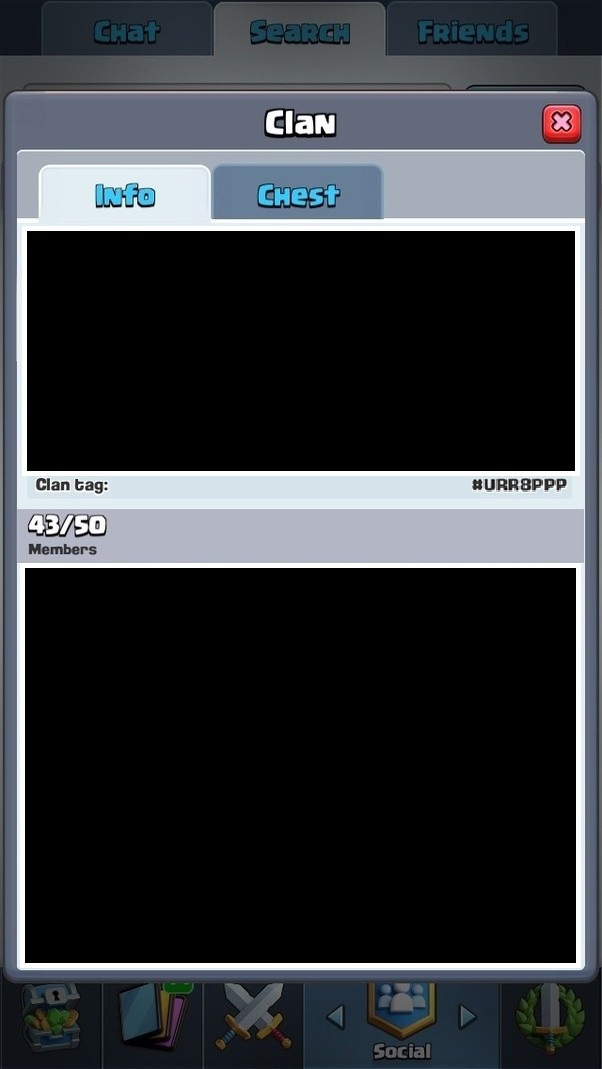

Java Prime Number Method
The question is exactly as follows:
Write a method that determines whether a number is prime.
Then use this method to write an application that determines and displays all the prime numbers less than 10,000.
I have already written a program that finds all prime numbers up to 10,000, but then found a simpler and more efficient one on StackOverflow, and that is this:
package prime;
import java.util.Scanner;
public class Prime
{
public static void main(String args)
{
for(int i = 1; i <= 10000; i++)
{
int factors = 0;
int j = 1;
while(j <= i)
{
if(i % j == 0)
{
factors++;
}
j++;
}
if (factors == 2)
{
System.out.println(i);
}
}
}
}
Since I am very new to Java and am especially not good at methods, this problem is especially difficult for me. I tried making a method, but nothing is being returned, and when I try to return something I get error after error after error.
The help I need is mainly just Pseudo-Code for what I should do to tackle this problem; I'm not asking you for the answer, I'm just asking for a start.
It should display integers, one after another.
– VMoza
Oct 8 '14 at 0:43
Ok, it does that already in the main method, so what you're asking is, how to put all that code into another method which you would then call in the main method?
– DreadHeadedDeveloper
Oct 8 '14 at 0:47
Yup------------
– VMoza
Oct 8 '14 at 0:52
Please don't replace questions with a 'final version'. Questions and their answers are meant to be helpful to future visitors too, not just yourself.
– Martijn Pieters♦
May 22 '15 at 14:36
7 Answers
7
package prime;
public class Prime
{
public static void main(String args)
{
for(int i = 1; i <= 10000; i++)
{
if (isPrimeNumber(i))
{
System.out.println(i);
}
}
}
public static boolean isPrimeNumber(int i) {
int factors = 0;
int j = 1;
while(j <= i)
{
if(i % j == 0)
{
factors++;
}
j++;
}
return (factors == 2);
}
}
Thanks for the help Subin, However, the program does not compile. If you could fix that up it'd be great. Thanks again!
– VMoza
Oct 8 '14 at 0:53
+1 for not including the for loop in the calculation of the method. This allows the method to be used elsewhere should the need arise
– DreadHeadedDeveloper
Oct 8 '14 at 0:54
see the latest edit by @dramzy
– ssk
Oct 8 '14 at 1:05
Write a method that determines whether a number is prime. Then use
this method to write an application that determines and displays all
the prime numbers less than 10,000.
It seems to me that you need a method that returns true if the number you pass it is prime and false otherwise. So, all you really need to do here is to move the portion of the code that deals with determining if a number is prime and, inside that method, instead of printing the number when it is prime, return true, otherwise return false. Then, inside the main method, print the number if that function indicates that the current integer is prime (by returning true) or do nothing otherwise. This is the best I can do to explain the solution without giving you the actual code.
EDIT:Since you asked for pseudocode:
main()
for i in 1..10000 :
if isPrime(i)
print i
isPrime(i):
factors = 0
num = 1
while num is less than or equal to i:
if i mod nums is 0:
increment factors
increment nums
return (factors == 2)
Thanks for the help dramzy, However, that isn't exactly Pseudo-Code - it's more like what the problem is stating in a different way. I do appreciate, however, your mentioning of the "true or false" idea. I believe Subin (above) was on that track as well. Thanks again!
– VMoza
Oct 8 '14 at 0:57
I have found one more solution using java streams:
import java.util.stream.LongStream;
public static boolean isPrime(long num) {
return num > 1 && LongStream.rangeClosed(2, (long)Math.sqrt(num)).noneMatch(div-> num % div== 0);
}
Here goes a test case to prove this works:
// First 168 primes, retrieved from https://en.wikipedia.org/wiki/Prime_number
private static final List<Integer> FIRST_168_PRIMES =
Arrays.asList(2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541, 547, 557, 563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619, 631, 641, 643, 647, 653, 659, 661, 673, 677, 683, 691, 701, 709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787, 797, 809, 811, 821, 823, 827, 829, 839, 853, 857, 859, 863, 877, 881, 883, 887, 907, 911, 919, 929, 937, 941, 947, 953, 967, 971, 977, 983, 991, 997);
@Test
public void testNumberIsPrime() {
FIRST_168_PRIMES.stream().forEach(integer -> assertTrue(isPrime(integer)));
}
@Test
public void testNumberIsNotPrime() {
List<Integer> nonPrime = new ArrayList<>();
for (int i = 2; i < 1000; i++) {
if (!FIRST_168_PRIMES.contains(i)) {
nonPrime.add(i);
}
}
nonPrime.stream().forEach(integer -> assertFalse(isPrime(integer)));
}
Works like a charm
– sebadagostino
Dec 6 '17 at 23:07
public boolean isPrime(long num)
{
double limit = Math.sqrt(num);
for (long i = 2; i < limit; i++)
if(num%i == 0)
return false;
return true;
}
This is optimal solution, but sqrt is calculated on every step. for(long i=2, sqrt = Math.sqrt(num); i <sqrt; i++){}
– oleg.cherednik
Aug 20 '17 at 2:41
Correct! Excellent spot, thanks for telling me!
– Brenann
Aug 26 '17 at 23:02
This works for detecting primes. But I am getting false positives, such as 4 or 9.
– sebadagostino
Dec 6 '17 at 23:05
/**
* List all prime numbers from 101 to 200
* for responding your question, I think it is a good view to have it done
* @ Joannama
*
*/
public class PrimeNum
{
public static void main(String args)
{
for ( int i = 101; i <= 200 ; i += 2)
{
// get rid of even number from 101 to 200
//because even number are never a prime number
boolean f = true;
//assume these numbers are prime numbers
for ( int j = 2; j < i; j++)
{
if ( i % j == 0)
{
f = false;
// if the reminder = 0,
//which means number is not a prime,
//therefore f = false and break it
break;
}
}
if ( ! f)
{
continue;
}
System.out.print("u0020" + i);
}
}
}
As the Brenann posted. Best way to calculate if its a prime, but with only one correction to the limit. You must add 1 to it, or it detects false positives.
public boolean isPrime(long num){
double limit = Math.sqrt(num) + 1;
for (long i = 2; i < limit; i++)
if(num%i == 0)
return false;
return true;
}
static int primeNumber(int n){
int i = 1, factor = 0;
while(i <= n){
if(n % i == 0){
factor++;
}
i++;
}
if (factor == 2){
return 1;
}
return 0;
}
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
So your question is how to make a method that returns a String holding the answers that you found? or Integer?
– DreadHeadedDeveloper
Oct 8 '14 at 0:42