Update multiple input values on option change
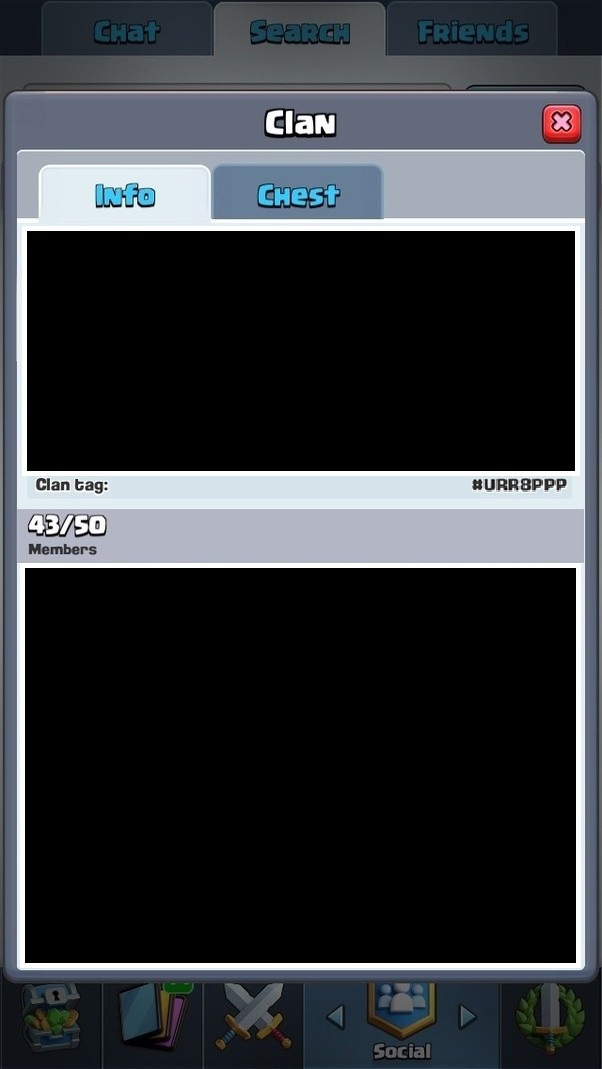

Update multiple input values on option change
I want to change multiple input values every time the <option>
changes, but the if
statement only evaluates the first condition and changes the values to '200'
and '300'
; it doesn't evaluate the other conditions, so it won't change the values when other options are selected. How can I fix this in jQuery?
<option>
if
'200'
'300'
$(function() {
$('input, #city').change(function(){
if ($('#a-city').attr('id') == 'a-city') {
$('#price1').val('200');
$('#price2').val('300');
} else if ($('#b-city').attr('id') == 'b-city') {
$('#price1').val('400');
$('#price2').val('500');
} else {
$('#price1').val('600');
$('#price2').val('700');
}
})
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<select id="city">
<option>Select a city</option>
<option id="a-city">Citya</option>
<option id="b-city">Cityb</option>
<option id="c-city">Cityc</option>
</select>
<input type="text" id="price1" value="50" readonly>
<input type="text" id="price2" value="100" readonly>
4 Answers
4
$("input, #city").change(function() {
const id = $(this).children(":selected").attr("id");
let priceOne, priceTwo;
switch (id) {
case "a-city": {
priceOne = 200;
priceTwo = 300;
break;
}
case "b-city": {
priceOne = 400;
priceTwo = 500;
break;
}
case "c-city": {
priceOne = 600;
priceTwo = 700;
break;
}
default: {
priceOne = 0;
priceTwo = 0;
}
}
$("#price1").val(priceOne);
$("#price2").val(priceTwo);
});
id
<select>
nice switch would be a better solution, allows more options to be added.
– Harry Chilinguerian
2 hours ago
Thanks a lot great switch answers, picked this one though, it is neater.
– D.C
52 mins ago
I am assuming you mean:
<select id="city">
<option>Select a city</option>
<option value="a-city">Citya</option>
<option value="b-city">Cityb</option>
<option value="c-city">Cityc</option>
</select>
<input type="text" id="price1" value="50" readonly>
<input type="text" id="price2" value="100" readonly>
$(function() {
$('#city').on('change', function(){
if ($(this).val('id') == 'a-city') {
$('#price1').val('200');
$('#price2').val('300');
} else if ($(this).val('id') == 'b-city') {
$('#price1').val('400');
$('#price2').val('500');
} else {
$('#price1').val('600');
$('#price2').val('700');
}
})
});
If the city changes then the price changes, you won't need to pass in the input tag since then are not watching for the change event and you have them tagged as readonly.
id
should go on the <select>
as well, as it should be unique within the document.– Özgür Can Karagöz
2 hours ago
id
<select>
Here is one way to do it.
$(function() {
$('input, #city').change(function() {
var selectedId = $(this).children(":selected").attr('id'),
$price1 = $('#price1'),
$price2 = $('#price2');
switch (selectedId) {
case 'a-city':
$price1.val('200');
$price2.val('300');
break;
case 'b-city':
$price1.val('400');
$price2.val('500');
break;
default:
$price1.val('600');
$price2.val('700');
}
})
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<select id="city">
<option>Select a city</option>
<option id="a-city">Citya</option>
<option id="b-city">Cityb</option>
<option id="c-city">Cityc</option>
</select>
<input type="text" id="price1" value="50" readonly>
<input type="text" id="price2" value="100" readonly>
The condition you're checking,
$('#a-city').attr('id') == 'a-city'
asks whether #a-city
's ID is, in fact, a-city
. This will always be true, and none of the other branches will ever run. To get the ID of the selected <option>
, use
#a-city
a-city
<option>
$('#city :selected').attr('id')
You can implement this without any conditionals at all:
$(function () {
const cities = {
'a-city': {
'#price1': 200,
'#price2': 300
},
'b-city': {
'#price1': 400,
'#price2': 500
},
'c-city': {
'#price1': 600,
'#price2': 700
}
};
$('#city').change(function () {
const selectedCityID = $('#city :selected').attr('id');
const city = cities[selectedCityID];
for (let priceID in city) {
$(priceID).val(city[priceID]);
}
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<select id="city">
<option disabled selected>Select a city</option>
<option id="a-city">Citya</option>
<option id="b-city">Cityb</option>
<option id="c-city">Cityc</option>
</select>
<input type="text" id="price1" value="50" readonly>
<input type="text" id="price2" value="100" readonly>
Instead of the city ID being an if
condition or switch
case
, here it's the key to an object containing that city's prices. We then iterate over that object and set both prices.
if
switch
case
Also, only #city
needs to be monitored for changes, and since "Select a city" isn't actually a city, I made it disabled
.
#city
disabled
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
id
should go on the<select>
as well, as it should be unique within the document.– Özgür Can Karagöz
2 hours ago