How to read what's remaining in a Socket's InputStream after writing in it?
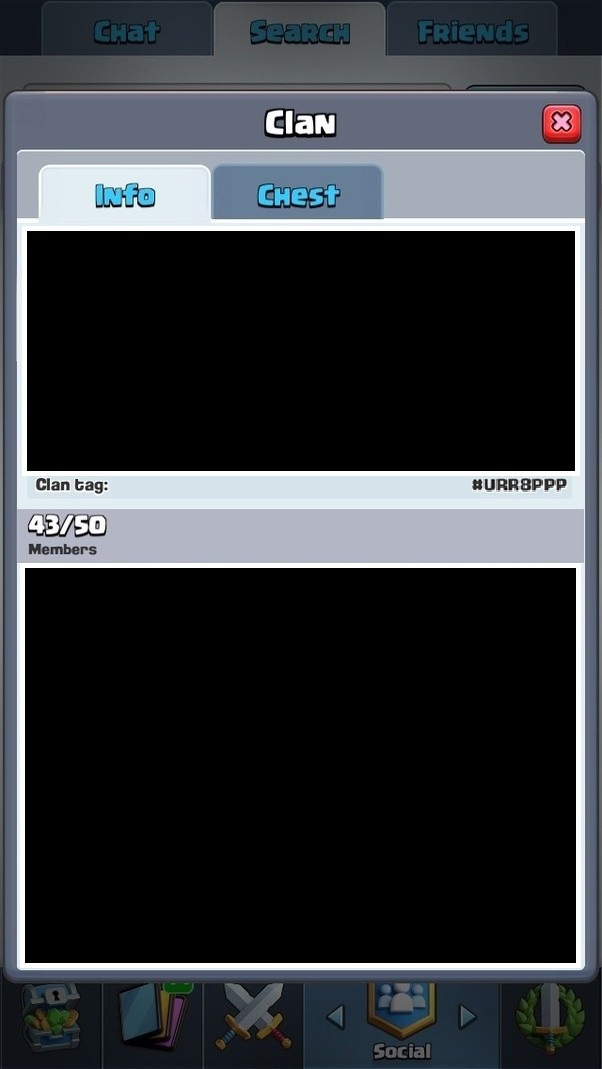

How to read what's remaining in a Socket's InputStream after writing in it?
I'm trying to read the full content of a socket inputstream that just got closed. If I just try reading it normally works however, I'm constantly trying to write in that socket's output stream in the same time. It looks like writing into it "breaks" the socket's inputstream and makes it impossible to read what's remaining.
Take this small example I made to illustrate my problem:
@Test
public void socketTest() throws Throwable
{
new Thread(() -> {
try
{
ServerSocket serverSocket = new ServerSocket(5555);
while(true)
{
//keep listening
Socket socket = serverSocket.accept();
InputStream in = socket.getInputStream();
System.out.println(in.read());
Thread.sleep(2000);
try
{
socket.getOutputStream().write(3);
}
catch(Throwable ex)
{
System.out.println("Error writing");
ex.printStackTrace();
}
System.out.println(in.read());
in.close();
socket.close();
System.exit(0);
}
}
catch(Throwable ex)
{
System.out.println("Error reading");
ex.printStackTrace();
}
}).start();
Socket socket = new Socket("localhost", 5555);
OutputStream out = socket.getOutputStream();
out.write(1);
out.write(2);
out.close();
socket.close();
synchronized(this)
{
wait();
}
}
I get the output:
1
java.net.SocketException: Software caused connection abort: recv failed
at java.net.SocketInputStream.socketRead0(Native Method)
at java.net.SocketInputStream.socketRead(SocketInputStream.java:116)
at java.net.SocketInputStream.read(SocketInputStream.java:170)
at java.net.SocketInputStream.read(SocketInputStream.java:141)
at java.net.SocketInputStream.read(SocketInputStream.java:223)
at net.jumpai.test.SocketTTest.lambda$socketTest$0(SocketTTest.java:55)
at java.lang.Thread.run(Thread.java:745)
Error reading
However the 2 was never read and writing 3 in the stream never made an error. I don't care if writing in the stream throws but I'd like to read the 2. Is there any way to do that?
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.