Access a JavaFX TextArea within Tab returned from a TabPane
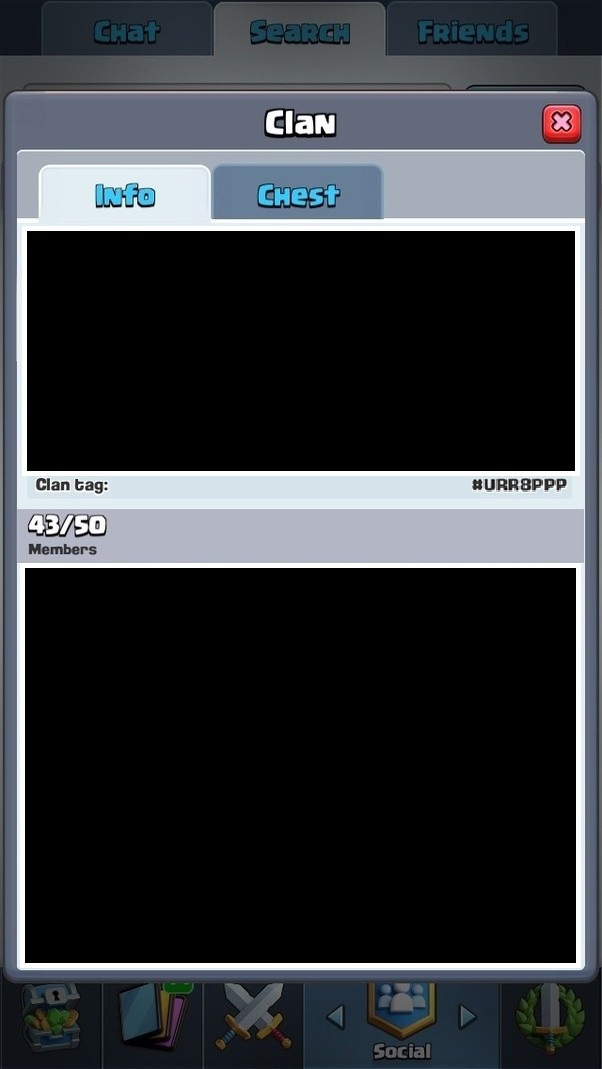

Access a JavaFX TextArea within Tab returned from a TabPane
I would like to access a text area placed within a Tab. All the Tabs in the TabPane will have a TextArea. Unfortunately Tab is not an interface and there does not seem a way to change the type held inside of the TabPane so I can not see a way to make code know that there is going to be a TextArea inside of the generic tab without keeping a separate list of them somewhere outside. TabPane can only return a Tab and tab does not distinguish that it holds a TextArea and even if I make an extension of Tab and give it to the TabPane it will still only return a Tab. Keeping an outside list seems really hacky and I dont think that would ever be the intended design. So what am I missing here. I am not using FXML.
Tab
TextArea
Show how you intend to use the code. We can't recommend a design without knowing how it will be used.
– user1803551
20 hours ago
1 Answer
1
You will need to create your own extension of a Tab
whose content is a TextArea
and provide a getTextArea()
method for it.
Tab
TextArea
getTextArea()
That way, you can know each Tab
will have a TextArea
and you can manipulate it however you would like.
Tab
TextArea
Below is a simple application to demonstrate:
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Tab;
import javafx.scene.control.TabPane;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Simple UI
VBox root = new VBox(10);
root.setAlignment(Pos.CENTER);
root.setPadding(new Insets(10));
// Create the TabPane
TabPane tabPane = new TabPane();
// Create some TextAreaTabs to put into the TabPane
tabPane.getTabs().addAll(
new TextAreaTab("Tab 1", "This is tab 1!"),
new TextAreaTab("Tab 2", "This is tab 2!"),
new TextAreaTab("Tab 3", "This is tab 3!")
);
// A button to get the text from each tab
Button button = new Button("Print Text");
// Set the action for the button to loop through all the tabs in the TabPane and print the contents of its TextArea
button.setOnAction(e -> {
for (Tab tab : tabPane.getTabs()) {
// You'll need to cast the Tab to a TextAreaTab in order to access the getTextArea() method
System.out.println(tab.getText() + ": " +
((TextAreaTab) tab).getTextArea().getText());
}
});
// Add the TabPane and button to the root layout
root.getChildren().addAll(tabPane, button);
// Show the stage
primaryStage.setScene(new Scene(root));
primaryStage.setWidth(300);
primaryStage.setHeight(300);
primaryStage.show();
}
}
// This is the custom Tab that allows you to set its content to a TextArea
class TextAreaTab extends Tab {
private TextArea textArea = new TextArea();
public TextAreaTab(String tabTitle, String textAreaContent) {
// Create the tab with the title provided
super(tabTitle);
// Set the Tab to be unclosable
setClosable(false);
// Set the text for the TextArea
textArea.setText(textAreaContent);
// Set the TextArea as the content of this tab
setContent(textArea);
}
public TextArea getTextArea() {
return textArea;
}
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You can of course cast the content of a
Tab
. A more direct way than looking for it in this node is probably more hacky than storing theTextArea
s in a proper data structure...– fabian
yesterday