Mutate value only for numeric columns AND only in the first and last row of a data frame using dplyr
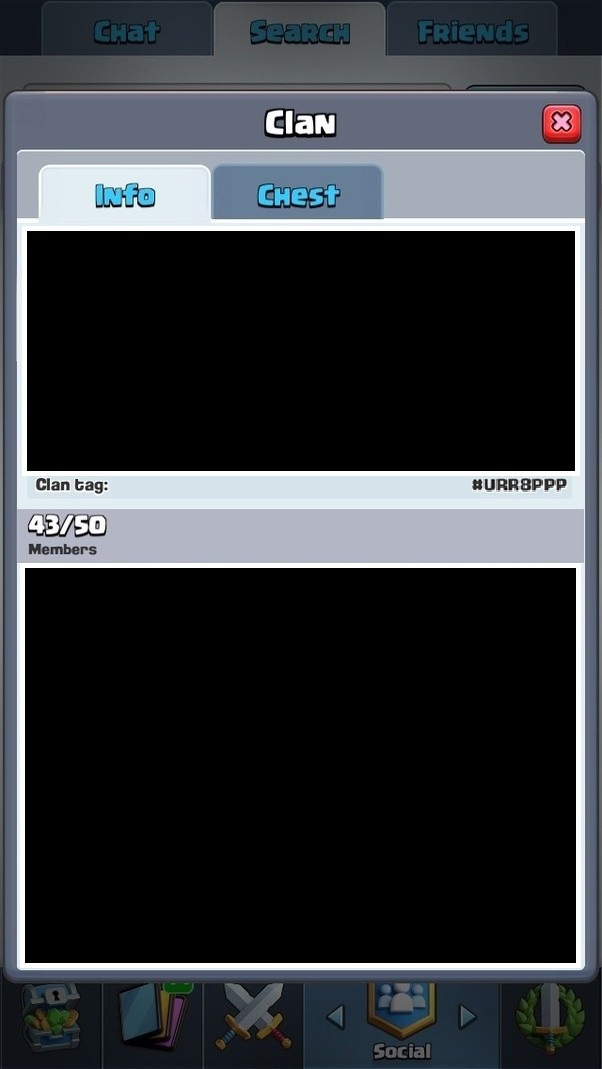

Mutate value only for numeric columns AND only in the first and last row of a data frame using dplyr
Given a data frame like:
library(dplyr)
library(lubridate)
df <- data.frame(
date = seq(ymd('2018-01-01'), ymd('2018-01-10'), by = 'days'),
location = "AMS",
V1 = seq(1:10),
V2 = seq(11:20)
)
I would like to use dplyr
to change the value of the first and last row, and only in numeric columns.
dplyr
I can do it for one column, as in:
df %>%
mutate(V1 = ifelse(row_number()==1, mean(V1)*100, V1)) %>%
mutate(V1 = ifelse(row_number()==nrow(.), mean(V1)*100, V1))
However I cannot manage to find a way to use mutate_at
or mutate_if
to do that for all numeric columns at once. Could you help me with that?
mutate_at
mutate_if
seq()
V1
V2
:
1 Answer
1
I think this addresses your issue:
df <- data.frame(
date = seq(ymd('2018-01-01'), ymd('2018-01-10'), by = 'days'),
location = "AMS",
V1 = 1:10,
V2 = 11:20
)
df %>% mutate_at(vars(V1, V2),
funs(ifelse(row_number() %in% c(1, n()), mean(.)*100, .)))
date location V1 V2
1 2018-01-01 AMS 550 1550
2 2018-01-02 AMS 2 12
3 2018-01-03 AMS 3 13
4 2018-01-04 AMS 4 14
5 2018-01-05 AMS 5 15
6 2018-01-06 AMS 6 16
7 2018-01-07 AMS 7 17
8 2018-01-08 AMS 8 18
9 2018-01-09 AMS 9 19
10 2018-01-10 AMS 550 1550
If you'd like to do it on all numeric columns, you can just use mutate_if
with is.numeric
as the .predicate
argument.
mutate_if
is.numeric
.predicate
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I suspect you don't mean to use
seq()
forV1
andV2
, as the:
notation already returns a vector– zack
16 mins ago