How to access nested object in array which is already in object with loops
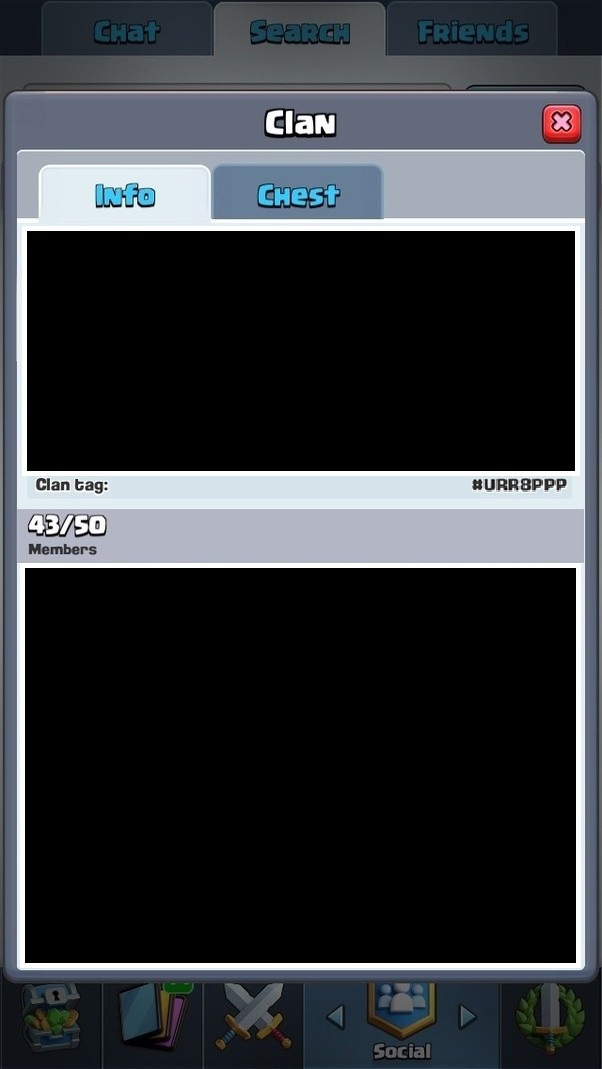

How to access nested object in array which is already in object with loops
var jsonObj = [
{
property1 : "one",
property2 : "two",
property3 : "three",
property4 : [
{
name: "nicholas",
name1: "cage"
},
{
name: "stuart",
name1: "walker"
}
]
}
];
var strBuilder = ;
for(var key in jsonObj){
if (jsonObj.hasOwnProperty(key)) {
strBuilder.push("Key is " + key + ", value is " + jsonObj[key] + "n");
}
}
alert(strBuilder.join(""));
I am getting object object when it reached near to property 4,
but I want to access the whole code with a loop.
3 Answers
3
When you get to property4
your function tries to turn an array of objects into a string, which as you noticed doesn't work very well. You need to take each of those objects in the array and process them in the same way you're processing the current object. One way to do that is to create a function that takes an object, prints the keys, just like you are, but when it sees an array, it takes each one of them and passes it back through the function. This is called recursion. It's a good way to handed nested data especially if you don't know ahead of time how the data will be nested.
property4
Here's something to get it started:
var jsonObj={property1 : "one",property2 : "two",property3 : "three",property4 : [{name: "nicholas",name1: "cage"},{name: "stuart",name1: "walker"}]};
var strBuilder = ;
function stringer(obj) {
for (var key in obj) {
if (obj.hasOwnProperty(key)) {
if (Array.isArray(obj[key])) {
strBuilder.push("Key is " + key + ", value is an array containing: n")
obj[key].forEach(item => stringer(item)) // call it recursively
} else {
strBuilder.push("Key is " + key + ", value is " + obj[key] + "n");
}
}
}
}
stringer(jsonObj)
console.log(strBuilder.join(''))
You should be able to modify this for better formatting and to handle different kinds of nested things (like objects) if you want.
As you can see, The object is casted to string itself not what's inside the object. You might want to get the content first.
So I modified your code into a recursive function.
var jsonObj = [
{
property1 : "one",
property2 : "two",
property3 : "three",
property4 : [
{
name: "nicholas",
name1: "cage"
},
{
name: "stuart",
name1: "walker"
}
]
}
];
function objectStringer(obj){
var strBuilder = ;
for(var key in obj){
if (obj.hasOwnProperty(key)) {
if(typeof(obj[key]) == 'object')
strBuilder.push(objectStringer(obj[key]).join(""))
else
strBuilder.push("Key is " + key + ", value is " + obj[key] + "n");
}
}
return strBuilder;
}
console.log(objectStringer(jsonObj).join(""));
Create another loop while key is an object
for(var key in jsonObj){
if (jsonObj.hasOwnProperty(key)) {
strBuilder.push("Key is " + key + ", value is " + jsonObj[key] + "n");
}
if(typeof key==object){
for(var x in key){
if (jsonObj.hasOwnProperty(x)) {
strBuilder.push("Key is " + x + ", value is " + key[x] + "n");
}
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
The object is casted to string itself not what's inside the object. you might want to get the content first.
– Community
1 hour ago