Convert ISO date string to Timestamp
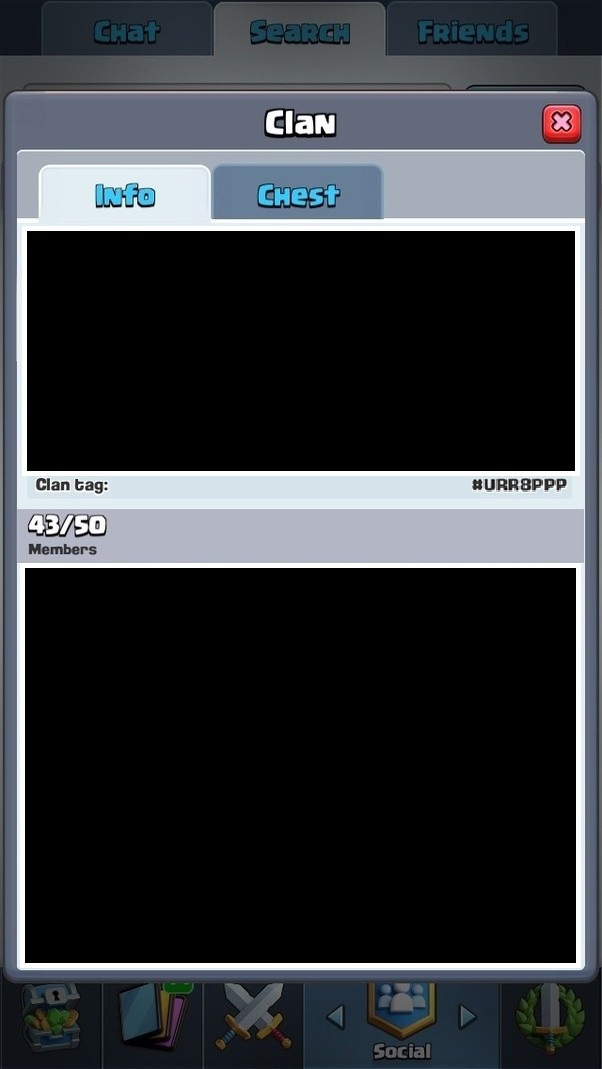

Convert ISO date string to Timestamp
String startDate = "2018-07-29T09:50:49+05:30";
String TAG = "Extra";
final String TIMESTAMP_FORMATE = "yyyy-MM-dd'T'HH:mm:ss.SSSXXX";
DateFormat df = new SimpleDateFormat(TIMESTAMP_FORMATE);
try {
Date date = df.parse(startDate);
System.out.println(TAG + "Start: " + date.getTime());
System.out.println(TAG + "Start: " + date.getDate());
System.out.println(TAG + "Start: " + date.getHours() + ":" + date.getTime());
} catch (ParseException e) {
e.printStackTrace();
}
Its giving an error java.text.ParseException: Unparseable date: "2018-07-29T09:50:49+05:30"
java.text.ParseException: Unparseable date: "2018-07-29T09:50:49+05:30"
Any idea what I am missing here?
You're missing the
.SSS
in your input, the milliseconds– user2718281
15 mins ago
.SSS
@AlmasAbdrazak The Joda Time library is made available in Java 8, under the package
java.util.time
.– MC Emperor
6 mins ago
java.util.time
3 Answers
3
.SSS
is for milliseconds, you do not need it, try:
.SSS
final String TIMESTAMP_FORMATE = "yyyy-MM-dd'T'HH:mm:ssXXX";
You can try this
String time="2018-07-29T09:50:49+05:30";
int ind = time.lastIndexOf('+');
time = time.substring(0, ind);
time = time+"Z";
System.out.println(time);
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ssZ");
try
{
Date date = dateFormat.parse(time.replaceAll("Z$", "+0000"));
System.out.println(date);
String TAG = "Extra";
System.out.println(TAG + "Start: " + date.getTime());
System.out.println(TAG + "Start: " + date.getDate());
System.out.println(TAG + "Start: " + date.getHours() + ":" + date.getTime()) ;
}
The new API turns out to be even easier in this case. Your pattern is the default format for java.time.ZonedDateTime
:
java.time.ZonedDateTime
ZonedDateTime date = ZonedDateTime.parse("2018-07-29T09:50:49+05:30")
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
stackoverflow.com/questions/2201925/… So probably you should use Joda-Time
– Almas Abdrazak
16 mins ago