C++: Weird segmentation fault related to const pointers to objects
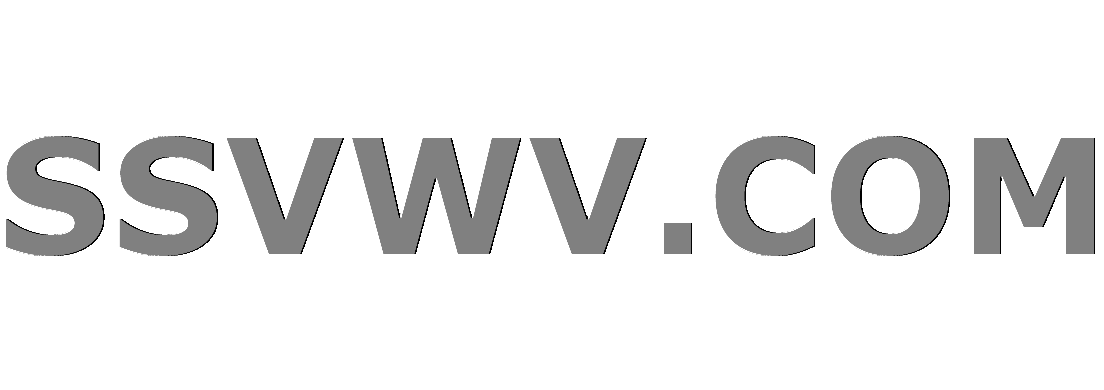
Multi tool use
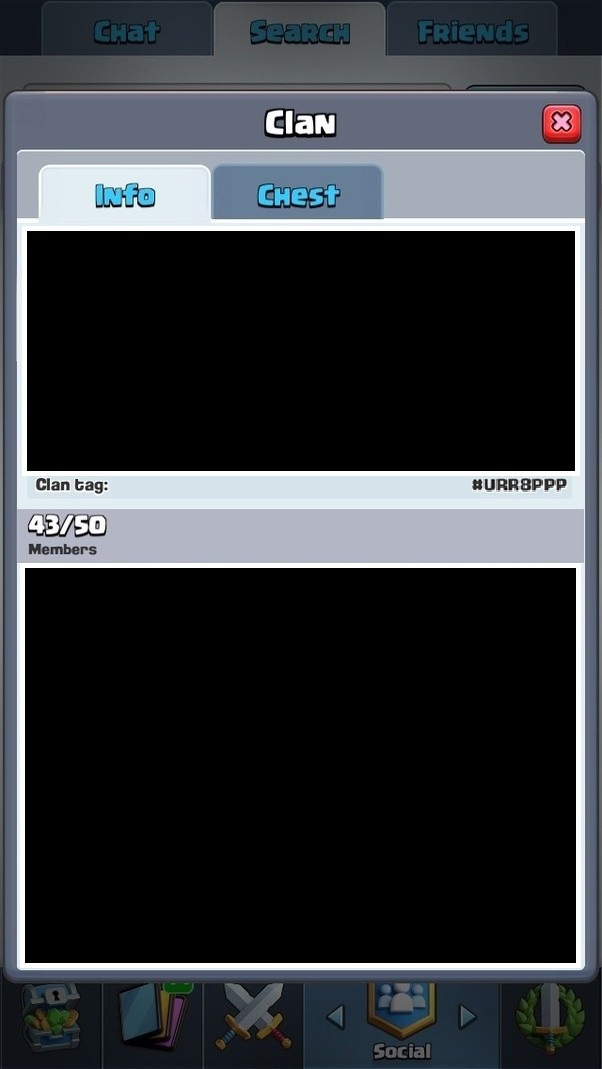

C++: Weird segmentation fault related to const pointers to objects
class A {
public:
A();
~A();
int** var;
void resetVar(); //sets variable to an init value
};
class B {
public:
B();
~B();
//either one of the below is declared, but not both in my code
A* const a_obj1; // const ptr to an instance of A, (option 1)
A* a_obj2; // ptr to an instance of A, (option 2)
};
A::A() {
var = new int*[2];
for( int i = 0; i < 2; i++ ) {
var[i] = new int[2];
}
resetVar();
}
A::~A() {
for ( int i = 0; i < 2; i++ ) {
delete var[i];
}
delete var;
}
A::resetVar() {
for ( int i = 0; i < 2; i++ ) {
for ( int j = 0; j < 2; j++ ) {
var[i][j] = 0; //point of segmentation fault, explained below
}
}
}
B::B():
a_obj1( new A() ) // option 1
{
a_obj2 = new A(); //option 2
a_obj2->resetVar(); //ok with option 2
a_obj1->resetVar(); //segmentation fault in this function call
}
B::~B() {
delete a_obj1;
delete a_obj2;
}
Note that this is a toned down version of my code.
There are 2 options in the above code:
B::a_obj1
is a const pointer to an instance of A
B::a_obj1
B::a_obj2
is a non-const pointer to an instance of A
B::a_obj2
A::resetVar()
is called twice for each object - once in A's constructor and again in B's constructor body. The problem is only with option 1 (const pointer) and segmentation fault occurs only when A::resetVar()
is called for the second time in B's constructor. I added an assertion in A::resetVar()
to see if the memory for A::var
actually exists. It gets triggered during the second call to resetVar()
in option 1.
A::resetVar()
A::resetVar()
A::resetVar()
A::var
resetVar()
What's going on?
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.