How do I implement search to happen across pages without making a call to the DB in MVC?
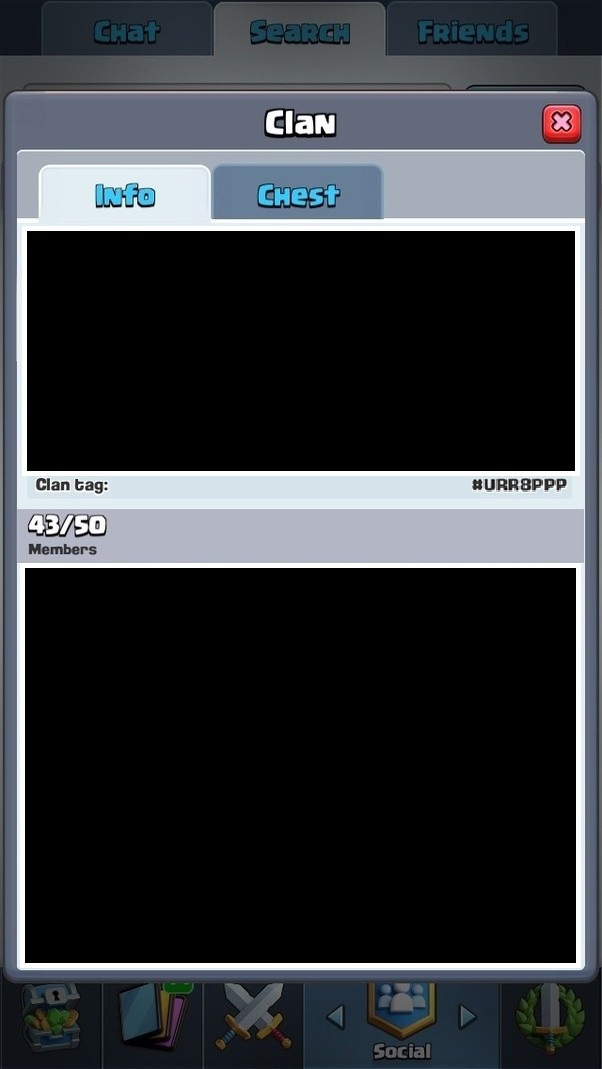

How do I implement search to happen across pages without making a call to the DB in MVC?
I have a search function that works fine,, but only for the first page. I have implemented the pagination concept in my application. Here's my search function:
function Search() {
var se = $('#SearchGrid').val().toUpperCase();
var i, td;
debugger;
var x = document.getElementById("VolunteerGrid");
var tr = x.getElementsByTagName("tr");
for (i = 0; i < tr.length; i++) {
td = tr[i].getElementsByTagName("td")[0];
if (td) {
if (td.innerHTML.toUpperCase().indexOf(se) > -1) {
tr[i].style.display = "";
} else {
tr[i].style.display = "none";
}
}
}
}
I want this search function to get all the rows and not just the rows on the first page and show the result.
Here's my simple view:
<div id="VolunteerGrid">
<table class="table">
<tr>
<th>
Name
</th>
<th>
Email
</th>
<th>
Phone Number
</th>
<th></th>
</tr>
@foreach (var volunteer in Model)
{
//var id = volunteer.VolunteerId;
<tr>
<td>
@Html.DisplayFor(modelItem => volunteer.Name)
</td>
<td>
@Html.DisplayFor(modelItem => volunteer.EmailAddress)
</td>
<td>
@Html.DisplayFor(modelItem => volunteer.PhoneNumber)
</td>
<td>
<a href="javascript:void(0)" id="Edit_btn" onclick ="EditVolunteer()">Edit</a> | <a href="#" id="Delete_btn">Delete</a>
</td>
</tr>
}
</table>
@Html.PagedListPager(Model, page => Url.Action("LoadVolunteers", new{page }))
I do not want to make a DB call to search my table. I know I can do that. Please look at the snapshot below
On the second page (not shown here), there's someone with letter 'E' in their name. When I search, it does not show the details of that person. It only takes the rows from the first page.
How do I return all the people based on the search input, without making a call to the DB? Thanks.
Edited after Rohits suggestion Still not working though
@using System.Web.UI.WebControls
@using VMS.Controllers
@using VMS.Models
@using System.Web.Optimization
@using DocumentFormat.OpenXml.Office.CustomUI
@using MvcPaging
@using Alignment = DocumentFormat.OpenXml.Math.Alignment
@using PagedList.Mvc;
@using PagedList;
@model PagedList.IPagedList<VMS.Models.VolunteerInfo>
@{
ViewBag.Title = "LoadVolunteer";
}
<script type="text/javascript" src="https://code.jquery.com/jquery-3.3.1.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/1.10.19/js/jquery.dataTables.min.js"></script>
<link rel="stylesheet" type="text/css" src="https://cdn.datatables.net/1.10.19/css/jquery.dataTables.min.css">
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.10.19/css/jquery.dataTables.css">
<script type="text/javascript" charset="utf8" src="https://cdn.datatables.net/1.10.19/js/jquery.dataTables.js"></script>
<h2>
Registered Volunteers
<button type="button" class="btn btn-group-sm btn-success pull-right" id="Excel_Btn">Export to Excel</button>
<img src="~/Images/search.png" width="20px" height="5px" style="position: fixed; margin: 0.4pc 0px 0pc 3.8pc; height: 4%;"/> <input type="text" id="SearchGrid" onkeyup="Search()" class="col-md-offset-1 customChanges" placeholder="Search for volunteer"/>
</h2>
<div id="VolunteerGrid">
<table class="table" id="tblVolunteer">
<tr>
<th>
Name
</th>
<th>
Email
</th>
<th>
Phone Number
</th>
<th></th>
</tr>
@foreach (var volunteer in Model)
{
//var id = volunteer.VolunteerId;
<tr>
<td>
@Html.DisplayFor(modelItem => volunteer.Name)
</td>
<td>
@Html.DisplayFor(modelItem => volunteer.EmailAddress)
</td>
<td>
@Html.DisplayFor(modelItem => volunteer.PhoneNumber)
</td>
<td>
<a href="javascript:void(0)" id="Edit_btn" onclick="EditVolunteer()">Edit</a> | <a href="#" id="Delete_btn">Delete</a>
</td>
</tr>
}
</table>
@Html.PagedListPager(Model, page => Url.Action("LoadVolunteers", new {page}))
</div>
<script type="text/javascript">
$(document).ready(function() {
$('#Excel_Btn').on('click',
function() {
$.ajax({
url: '@Url.Action("ExportToExcel", "ViewEditVolunteer")'
});
});
$('#tblVolunteer').dataTable({
"ajax": {
"url": '/ViewEditVolunteerController/LoadVolunteers',
"type": "GET",
"dataType": 'json',
"data": model
},
oLanguage: { "sZeroRecords": "No Records Found" },
bDestroy: true,
bFilter: false,
bInfo: true,
aaSorting: ,
bLengthChange: false,
columns: [
{ "sTitle": "Name", "data": "name" },
{ "sTitle": "Email", "data": "Email" },
{ "sTitle": "Phone No", "data": "phone" },
{ "sTitle": "Action", "data": "" }
],
"fnRowCallback": function(nRow, aaData) {
$("td:eq(4)", nRow).html("<a onClick='EditVolunteerInfo(" +
aaData.VolunteerId +
")'>Edit</a> | <a onClick='DeleteVolunteerInfo(" +
aaData.VolunteerId +
")'>Delete</a>");
}
});
});
function Search() {
var se = $('#SearchGrid').val().toUpperCase();
var i, td;
var x = document.getElementById("VolunteerGrid");
var tr = x.getElementsByTagName("tr");
for (i = 0; i < tr.length; i++) {
td = tr[i].getElementsByTagName("td")[0];
if (td) {
if (td.innerHTML.toUpperCase().indexOf(se) > -1) {
tr[i].style.display = "";
} else {
tr[i].style.display = "none";
}
}
}
}
</script>
Previously loaded? It is already loaded isin't it? I mean that's why I am getting all the rows right?
– Priyanka Dembla
23 hours ago
I have tried to be specific. Sorry if I wasn't specific enough. I want to search my table without making a call to my DB.
– Priyanka Dembla
23 hours ago
Just by looking your code I can't tell whether it's previously loaded or not. It looks everytime you change your current page, the
LoadVolunteers
action is called, thus making a new query on the database.– Alisson
21 hours ago
LoadVolunteers
2 Answers
2
<table id="tblVolunteer" class="display" style="width:100%">
------------------------------------------------------------------------
$(document).ready(function() {
$('#tblVolunteer').dataTable({
"ajax": {
"url": '/HomeController/LoadVolunteerDetails',
"type": "GET",
"dataType": 'json',
"data": model
},
oLanguage: { "sZeroRecords": "No Records Found" },
bDestroy: true,
bFilter: false,
bInfo: true,
aaSorting: ,
bLengthChange: false,
columns: [
{ "sTitle": "Name", "data": "name" },
{ "sTitle": "Email", "data": "Email" },
{ "sTitle": "Phone No", "data": "phone" },
{ "sTitle": "Action", "data": ""}
],
"fnRowCallback": function (nRow, aaData) {
$("td:eq(4)", nRow).html("<a onClick='EditVolunteerInfo(" + aaData.VolunteerId + ")'>Edit</a> | <a onClick='DeleteVolunteerInfo(" + aaData.VolunteerId + ")'>Delete</a>");
}
});
}});
------------------------------------------------------------------------
In addition to the above code, the following two Javascript library files must be added:
https://code.jquery.com/jquery-3.3.1.js
https://cdn.datatables.net/1.10.19/js/jquery.dataTables.min.js
The following CSS library files must be added for use to provide the styling of the table:
https://cdn.datatables.net/1.10.19/css/jquery.dataTables.min.css
Please refer this link for more details: refer this
Hi Rohit. Tried your code but not sure if I have implemented it correct. I have edited my question. It has the full LoadVolunteers.cshtml code. Please tell me what I am doing wrong. Thank you.
– Priyanka Dembla
3 mins ago
Use jQuery DataTable plugin instead of what you did above that will be more flexible to implement and can handle huge records, It's open source.
Please refer: https://datatables.net/ for more info.
Make ajax call and bind the json response in the DOM something like below:
$('#myTable').DataTable( {
ajax: {
url: '/api/myData',
dataSrc: ''
},
columns: [ ... ]
} );
This looks like a comment, not an answer.
– Alisson
yesterday
I am not sure what you're trying to say here. Can you please add a little detail?
– Priyanka Dembla
23 hours ago
@PriyankaDembla, I have provided code snippet for your reference to implement the jQuery DataTable.
– Rohit Gupta
21 hours ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You need to have all the items (from all pages) previously loaded somehow. This could be done either via javascript (storing the data in the client's browser memory), or by making ajax calls to your backend API, which would have the data in a cache (possibly by user). Anyway, the answer to this question is very broad, it would help if you could be more specific.
– Alisson
yesterday